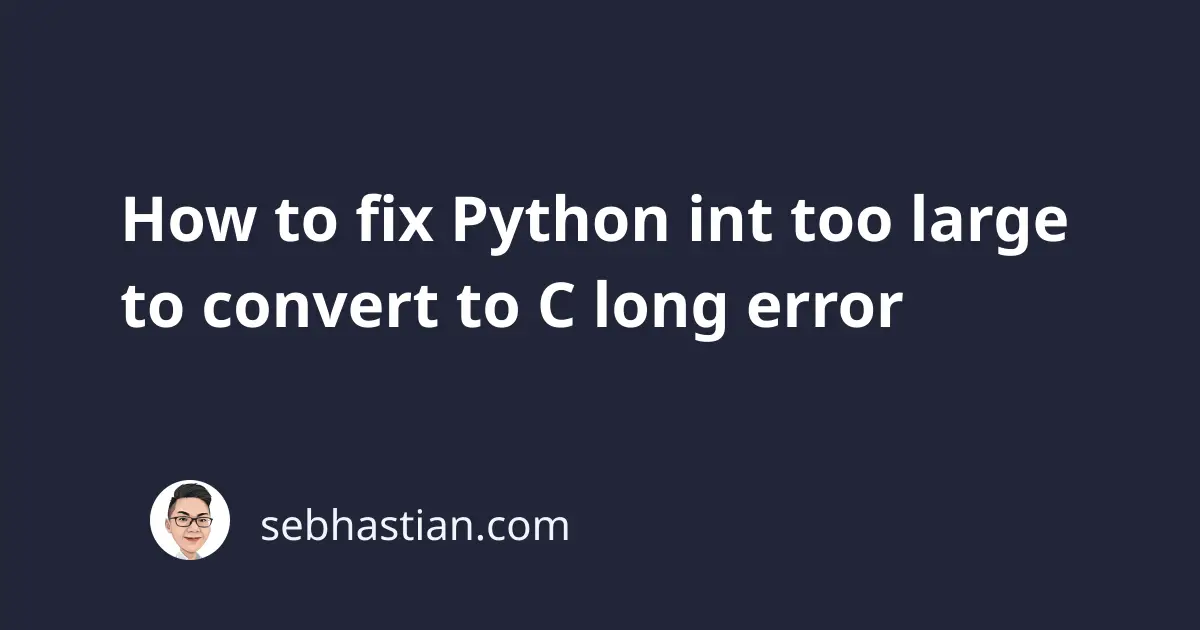
If you’re working with large integer numbers in NumPy, you might get the following error:
OverflowError: Python int too large to convert to C long
This error occurs when the integer number you specified is greater than the size that C long type can handle.
The maximum size that can be handled in C long is as follows:
- The
int
type is 32-bit which can hold a maximum value of2147483647
- The
int64
type is 64-bit which can hold a maximum value of9223372036854775807
This tutorial shows an example that causes this error and how to fix it.
How to reproduce this error
Suppose you’re trying to add a large number to a NumPy array as follows:
import numpy as np
arr = np.array(2147483649, dtype=int)
print(arr)
If you installed the 32-bit Python program in Windows, then the integer number 2147483649
will cause an error because it’s greater than the maximum value the int
type can handle:
Traceback (most recent call last):
File "main.py", line 3, in <module>
arr = np.array(2147483649, dtype=int)
OverflowError: Python int too large to convert to C long
On the other hand, if you have a 64-bit Python program installed, integer numbers greater than 9223372036854775807
will cause this error:
import numpy as np
arr = np.array(9223372036854775808, dtype=int) # ❌
print(arr)
The Numpy int
type is equivalent to the long int
type in C programming language, which is used internally by Python 2.
In Python 3, the int
type is implemented as arbitrary-precision integers, which basically means the integer value is only limited by the available memory in the system.
But the NumPy int
type has yet to use the Python 3 implementation, so it’s still limited to the maximum value a long int
type can handle.
How to fix this error
If you’re on Windows, then you can change the dtype
to int64
to increase the maximum integer value that NumPy can handle:
import numpy as np
arr = np.array(2147483649, dtype=np.int64)
print(arr) # 2147483649
If you’re already on a 64-bit system, then the maximum integer value you can store in NumPy is 9223372036854775807
. Python will raise the error when you try to store a value greater than that.
You can check the maximum allowed integer size in the sys.maxsize
constant:
import sys
print(sys.maxsize)
# 2147483647 / 9223372036854775807
The NumPy int64
type will always be limited by this maximum size, unlike Python 3 int
which can hold super big numbers:
# A list in Python 3
x = [9223372036854775899]
print(x) # [9223372036854775899]
And that’s how you fix the OverflowError: Python int too large to convert to C long
error. Happy coding! 🙌