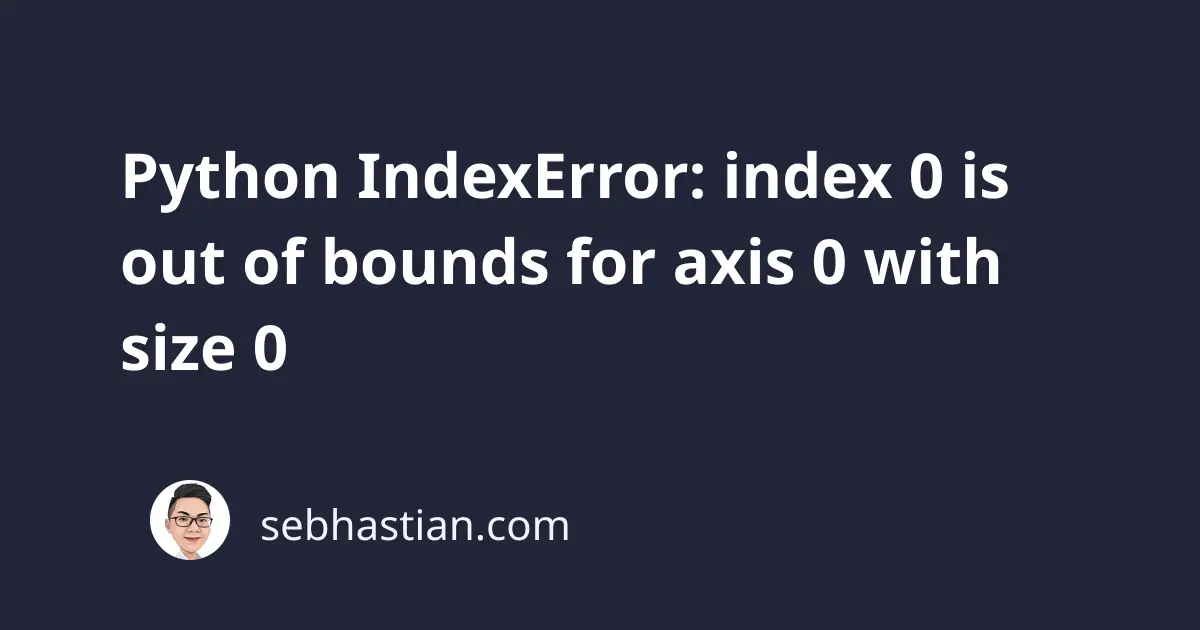
This article will show you how to fix the IndexError: index 0 is out of bounds for axis 0 with size 0
error that occurs in Python.
This error appears when you try to access a NumPy array that is empty.
Here’s an example code that would trigger this error:
import numpy as np
arr = np.array([])
val = arr[0] # ❌
The highlighted line above tries to access the element at index 0
of an empty array.
Python responds with the following error message:
Traceback (most recent call last):
File ...
val = arr[0]
IndexError: index 0 is out of bounds for axis 0 with size 0
The arr
variable is empty, so the index 0 is out of bounds.
To fix this error, you need to ensure that the NumPy array is not empty before trying to access its elements.
You can do this with an if
statement as follows:
import numpy as np
arr = np.array([])
if arr.size > 0:
val = arr[0]
else:
print("Array is empty")
The if
block in the code above only runs when the array size
is greater than 0.
Another way to fix this error is to use the try-except
statement to catch the error and handle it gracefully:
import numpy as np
arr = np.array([])
try:
val = arr[0]
except IndexError:
print("Array is empty")
When working with NumPy arrays, make sure the index you are trying to access is within the bounds of the array.
Another variation of this error is when you get index 1 is out of bounds for axis 0 with size 1
.
This error means that you are trying to access the element at index 1 when the array only has a size of 1
.
The code below triggers this error:
import numpy as np
arr = np.array([1])
val = arr[1]
Numpy array index are zero-based, so the first element is at index 0.
This means arr[1]
is trying to access the second element, which doesn’t exist in the array.
The error message would be:
Traceback (most recent call last):
File ...
val = arr[1]
IndexError: index 1 is out of bounds for axis 0 with size 1
Just like the previous error, you need to make sure you are accessing an element that exists.
I hope this tutorial helps you solve the index out of bounds error. 🙏