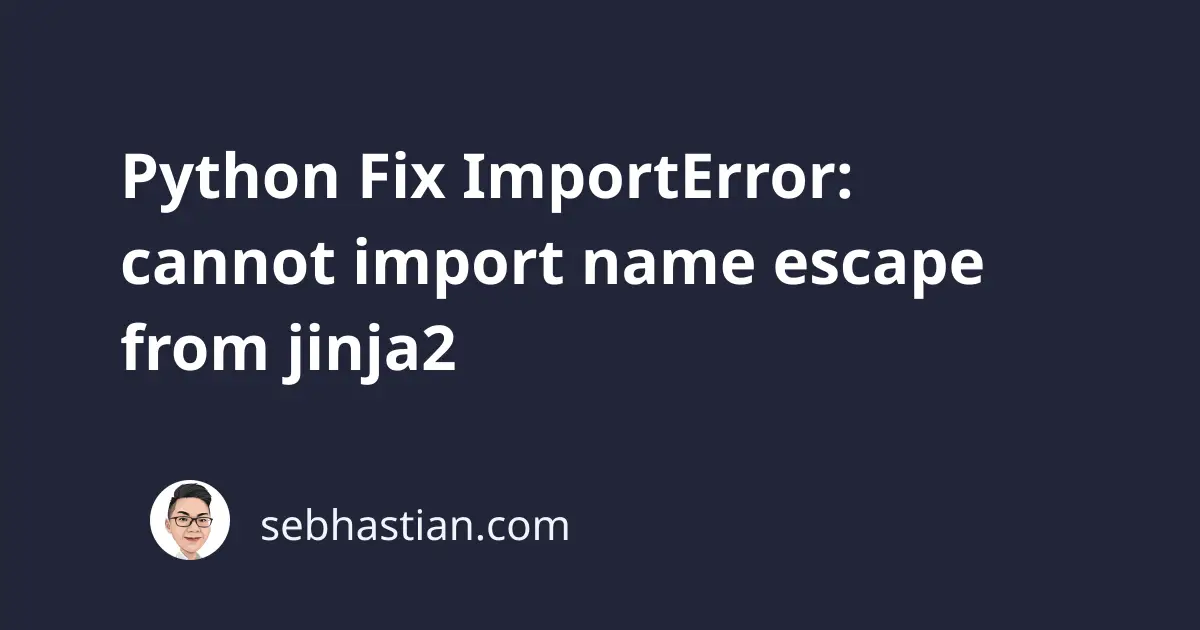
Having trouble importing the escape
function from the Jinja2 module in your Python project? You’re not alone!
The ImportError: cannot import name 'escape' from jinja2
error is a common issue that many developers encounter when working with the Jinja2 module.
In this article, we will discuss why this error occurs and how to fix it.
Why this error occurs?
The ImportError: cannot import name 'escape' from jinja2
occurs because the escape
function was removed from the Jinja2 module in version 3.1.0.
Suppose you have the following import
statement in your code:
from jinja2 import escape
When running with the latest Jinja2 module, Python will give the following error:
Traceback (most recent call last):
File ...
from jinja2 import escape
ImportError: cannot import name 'escape' from 'jinja2'
The escape
function was moved from Jinja2 to the MarkUpSafe module in order to promote better separation of concerns.
Solution#1 - Refactor your import statement
To fix the error, replace your import
statement as follows:
from markupsafe import escape
The MarkUpSafe module is included as a dependency of the Jinja2 module, so you don’t need to use pip
to separately install the module.
Solution#2 - Downgrade your Jinja2 version
Alternatively, you can downgrade your Jinja2 module to the latest version that still supports the escape
function.
The latest Jinja2 version that includes the escape
function is version 3.0.3, so use pip
to downgrade the module as follows:
pip install jinja2==3.0.3 --force-reinstall
# for pip3:
pip3 install jinja2==3.0.3 --force-reinstall
This solution may be viable if you have a complex project and require more time to plan and execute the version upgrade.
For Flask users: Upgrade to Flask version 2
If you are using the Flask framework, then this error happens because Flask version 1 used the escape
function from Jinja2 in its code.
You need to upgrade your Flask module to the latest version using pip
. Run one of the commands below in your terminal:
pip install flask --upgrade
# for pip3:
pip3 install flask --upgrade
The latest Flask version already fixed this issue, so you should have no problem running your web application.
If you have a requirements.txt
file, you can instruct pip to install an explicit version of Jinja2 as follows:
jinja2==3.0.3
This is a good solution to postpone the need to upgrade your Jinja2 version immediately.
Conclusion
In this article, you’ve learned why the ImportError: cannot import name escape from jinja2
error occurs and how to fix it.
We looked at two solutions: refactoring your import statement and downgrading your Jinja2 version.
For Flask users, an upgrade of the Flask module was also necessary to use the latest Jinja2 version.
Pick the solution that works for you, and you should have no problem using the escape
function in your Python project. 🙌