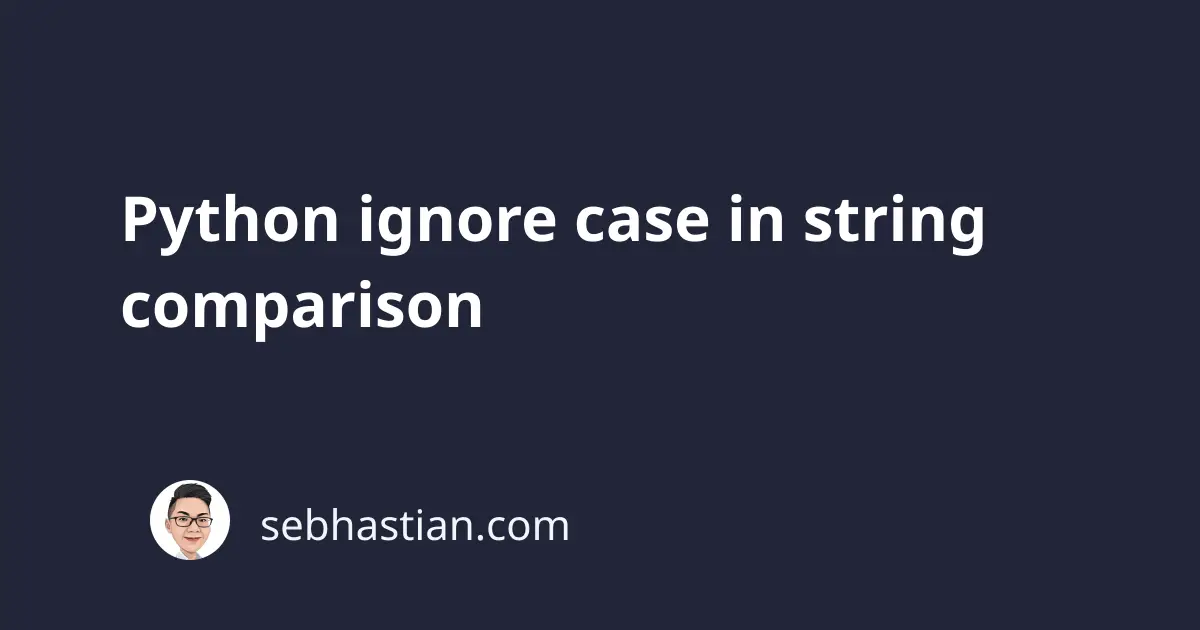
When you need to do a string comparison, Python will take into account the case used by the strings.
This will make identical strings that use different cases to be not equal. The following example returns False
:
string_1 = "HELLO"
string_2 = "hello"
print(string_1 == string_2) # False
If you want to ignore the case in the comparison, you need to call the str.casefold()
method when running the comparison.
The casefold()
returns the string you called this method from in a caseless version:
string_1 = "HELLO"
string_2 = "hello"
res = string_1.casefold() == string_2.casefold()
print(res) # True
The casefold()
method is similar to the lower()
method, only that the casefold()
method converts more characters into lower cases compared to lower()
.
For example, the German letter ‘ß’ will be converted to ‘ss’ using the casefold()
method, while the lower()
method does nothing:
string_1 = "ß"
string_2 = "ss"
print(string_1.casefold() == string_2) # True
print(string_1.lower() == string_2) # False
Keep in mind that the casefold()
method is only available in Python 3.3 and up, so if you need to support Python 2, the best you can do is to use the lower()
method.
And that’s how you do a case-insensitive string comparison in Python. I hope this tutorial helps! 👍