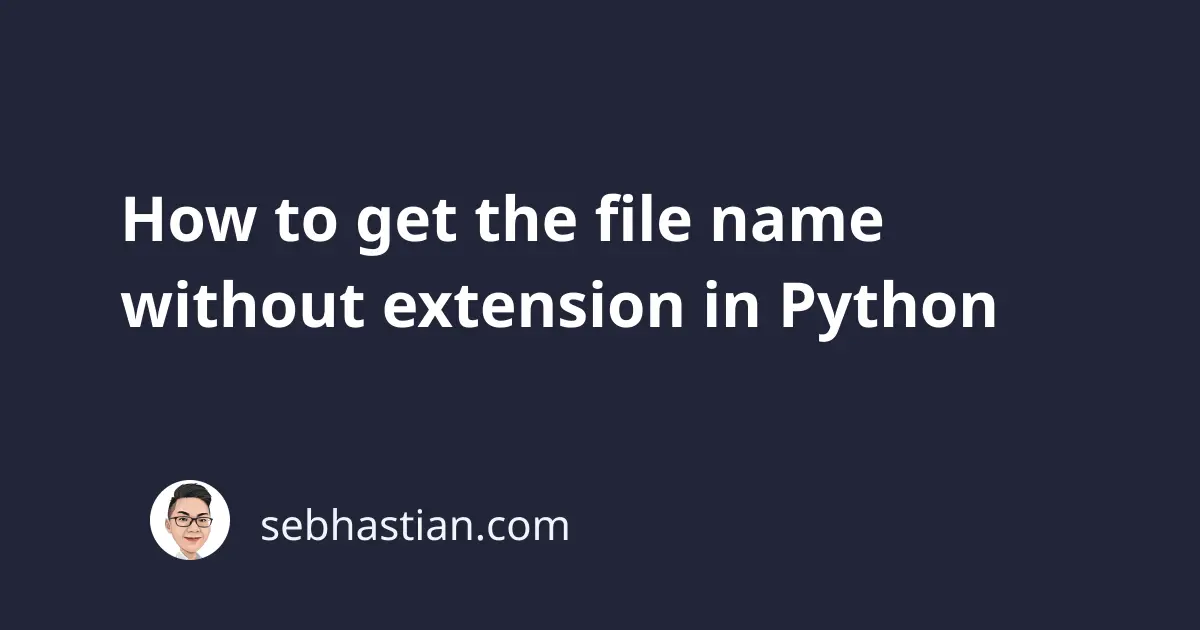
There are three ways you can get the file name without extension in Python:
- Using the
os
module - Using the
pathlib
module - Using the
split()
method
This tutorial will show you how to use the three methods in practice, as well as their differences.
Get file name using the os module
To get the file name without extension in Python, you can use the built-in os
module basename()
method to extract the file name from the full path, then remove the extension using the splitext()
method.
For example, suppose you want to get the file name from a location as follows:
"/path/to/some/file.txt" -> "file"
You can use the os
module as shown below:
import os
path = "/path/to/some/file.txt"
file_name = os.path.basename(path)
print(file_name) # file.txt
file_no_extension = os.path.splitext(file_name)[0]
print(file_no_extension) # file
The os.path.basename()
method returns the last section of a pathname, while the splitext()
method splits the extension from a pathname.
The splitext()
method returns a tuple containing (filename, extension), so we pick the first item in the tuple using [0]
index notation.
Get file name using the pathlib module
Beginning in Python version 3.4, you can use the Path
class from the pathlib
module to create a Path
object.
The Path
object has the stem
property, which stores the last section of the pathname without the extension.
Here’s how to use the Path
class:
from pathlib import Path
path = "/path/to/some/file.zip"
file_no_extension = Path(path).stem
print(file_no_extension) # file
This solution is preferred if you use the latest version of Python, but if you have to support older Python versions, then use the os
module instead.
Get file name with the split() method
If you don’t want to import any module, then you can also use the str.split()
method to get the file name without the extension.
First, call the split()
method from the path
object to get the file name:
path = "/path/to/some/file.txt"
file_name = path.split('/')[-1]
print(file_name) # file.txt
Next, call split()
on the file_name
object to get the file name without the extension:
file_no_extension = file_name.split('.')[0]
print(file_no_extension) # file
The advantage of this method is that you will always get the file name without extension even when you have two or more extensions.
Suppose you have the .tar.gz.bz.7zip
extensions as shown below:
path = "/path/to/some/file.tar.gz.bz.7zip"
file_name = path.split('/')[-1]
print(file_name) # file.tar.gz.bz.7zip
file_no_extension = file_name.split('.')[0]
print(file_no_extension) # file
As you can see, the split()
method correctly returns only the file name in the second call.
But the disadvantage of this method is that the first split call won’t work if you have a path system with a different separator, such as Windows that uses the \\
notation for the path structure.
Instead of calling the split()
method two times, you can change the first call with either os.path.basename()
method or Path()
constructor as follows:
import os
path = "/path/to/some/file.tar.gz.bz.7zip"
file_name = os.path.basename(path)
print(file_name) # file.tar.gz.bz.7zip
file_no_extension = file_name.split('.')[0]
print(file_no_extension) # file
Here’s the alternative using the pathlib
module:
from pathlib import Path
path = "/path/to/some/file.tar.gz.bz.7zip"
file_no_extension = Path(path).stem
print(file_no_extension) # file.tar.gz.bz
if '.' in file_no_extension:
file_no_extension = file_no_extension.split('.')[0]
print(file_no_extension) # file
By combining str.split()
with the os
or pathlib
module, you can extract the file name that has multiple extensions with ease.
I hope this tutorial is helpful. See you again in other tutorials! 👍