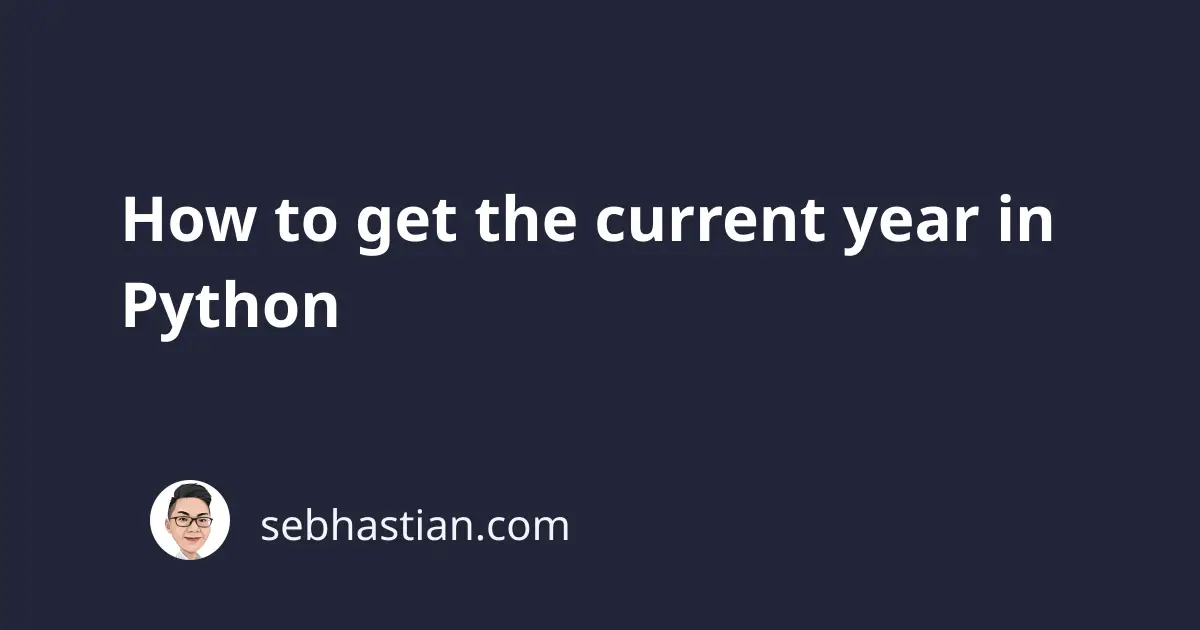
To get the current year in Python, you can use the datetime
module’s datetime
class to create an object that stores the current datetime.
After that, you can access the year
property from the datetime object as follows:
from datetime import datetime
current_date = datetime.now()
print(current_date.year) # 2023
If you need to get only the last 2 digits of the year, you can use the strftime()
method to format the time string with %y
format like this:
from datetime import datetime
current_year = datetime.now().strftime('%Y')
current_year_2 = datetime.now().strftime('%y')
print(current_year) # 2023
print(current_year_2) # 23
The strftime()
method allows you to format the datetime object into a string with your preferred format.
Since we only want the year part, you can use %Y
for a four-digit year and %y
for a two-digit year.
Besides the now()
method, you can also use the today()
method to get the current year:
from datetime import datetime
current_year = datetime.today().year
current_year_2 = datetime.today().strftime('%y')
print(current_year) # 2023
print(current_year_2) # 23
The difference between today()
and now()
is that we can pass the timezone info with now()
.
But since we’re only interested in the year part, the timezone info won’t be needed unless you’re dealing with time-sensitive context such as during the new year.
If you need the year part to be absolutely accurate according to the timezone, then it’s better to use the now()
method.
I hope this tutorial is helpful. Until next time! 👋