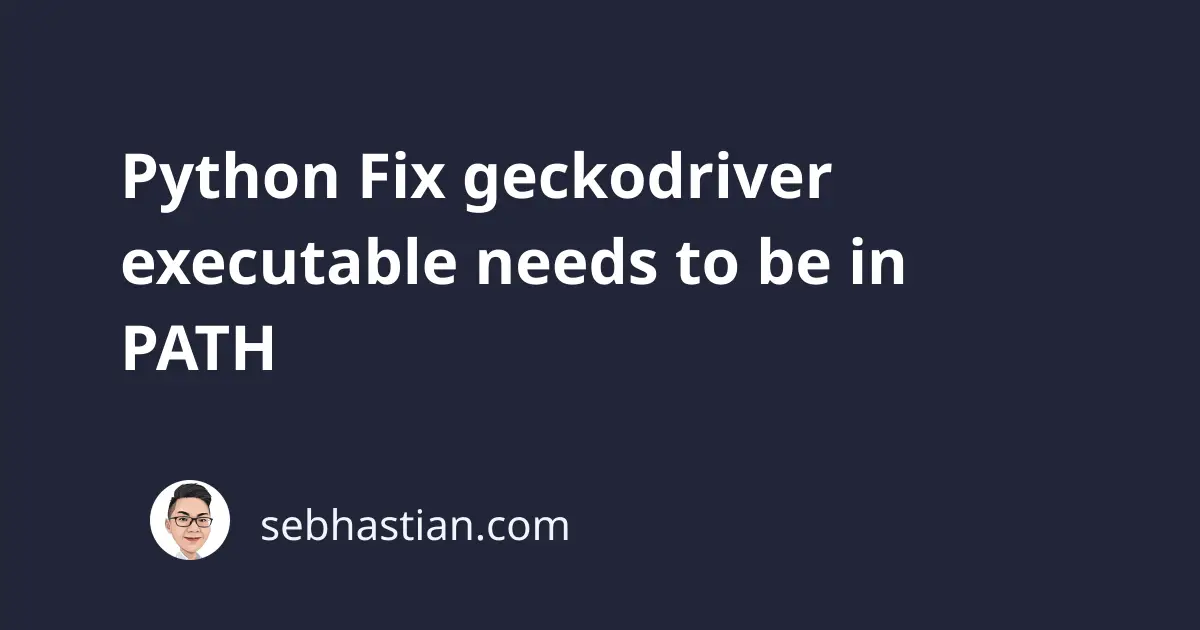
Are you a Python developer who’s struggling with the pesky geckodriver executable needs to be in PATH
error? Don’t worry, you’re not alone.
This error can crop up when running Selenium with Firefox, and it can be confusing for those unfamiliar with the problem.
Fortunately, it’s relatively easy to solve once you know what’s going on. This article will help you to troubleshoot and solve the error.
What Does geckodriver executable needs to be in PATH Mean?
Python shows geckodriver executable needs to be in PATH
message when you use the Selenium library to automate Firefox, but the geckodriver
executable is not found in the system’s PATH.
GeckoDriver is a web browser engine used by Mozilla Foundation to build the Firefox browser. It’s required by Selenium to run automated tests in Firefox.
Suppose you call the webdriver.Firefox()
method as shown below:
from selenium import webdriver
browser = webdriver.Firefox()
Without the geckodriver
, Python will give the following error:
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File ...
raise WebDriverException(
selenium.common.exceptions.WebDriverException:
Message: 'geckodriver' executable needs to be in PATH.
To fix this error, you need to make sure that the geckodriver executable is in the system’s PATH.
There are two solutions to fix this error:
- Use the webdriver_manager library to help you install web drivers
- Manually download Geckodriver and make it available under your PATH
Let’s see how these solutions work next.
Use the webdriver_manager library
The webdriver_manager
library is used to install web drivers. This library can be used to install the geckodriver
required by Selenium to automate Firefox.
First, install the webdriver_manager
library using pip
as follows:
pip install webdriver_manager
# or if you use pip3:
pip3 install webdriver_manager
Once installed, import the GeckoDriverManager
into your code. The code is a bit different depending on your Selenium version:
# selenium 4
from selenium import webdriver
from selenium.webdriver.firefox.service import Service as FirefoxService
from webdriver_manager.firefox import GeckoDriverManager
browser = webdriver.Firefox(
service=FirefoxService(GeckoDriverManager().install()))
# selenium 3
from selenium import webdriver
from webdriver_manager.firefox import GeckoDriverManager
browser = webdriver.Firefox(
executable_path=GeckoDriverManager().install())
The GeckoDriverManager
will automatically check if the geckodriver executable is in the PATH or not. If not, it will download the latest version of geckodriver
and add it to the PATH.
Once you get the geckodriver executable, you should be able to run your automated Firefox tests.
Keep in mind that you may also encounter a similar error when automating other browsers such as Chrome or Edge.
You can download the Chrome driver from webdriver_manager
like this:
# selenium 3
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
from webdriver_manager.core.utils import ChromeType
driver = webdriver.Chrome(
ChromeDriverManager(chrome_type=ChromeType.CHROMIUM).install())
# selenium 4
from selenium import webdriver
from selenium.webdriver.chrome.service import Service as ChromiumService
from webdriver_manager.chrome import ChromeDriverManager
from webdriver_manager.core.utils import ChromeType
driver = webdriver.Chrome(
service=ChromiumService(
ChromeDriverManager(chrome_type=ChromeType.CHROMIUM).install()))
The code examples for other browsers can be found at webdriver_manager pypi page.
Using the webdriver_manager
is convenient because it removes the need to manually download and edit your system PATH.
If you don’t want to use the webdriver_manager
, you can install the geckodriver
manually.
Download the geckodriver manually
You can find geckodriver executable from its GitHub repo and place it in a directory that is already in the system’s PATH.
For Windows, you can place the executable in the System32
folder.
For Mac, you can place the driver in /usr/local/bin
or /usr/bin
.
Alternatively, you can include the directory where the executable was downloaded in your PATH variable.
Update: Selenium 4.6.0 no longer require web drivers
In Selenium version 4.6.0, the Selenium Manager was released to help developers simplify their environment setup.
The Selenium Manager will maintain the web drivers for you, so you no longer need to download the drivers anymore!
To upgrade your Python Selenium bindings, use the pip
command below:
pip install selenium --upgrade
# for pip3:
pip3 install selenium --upgrade
I’ve tested the latest version personally, and my Selenium opens the Firefox browser without any error.
Cheers! 👍