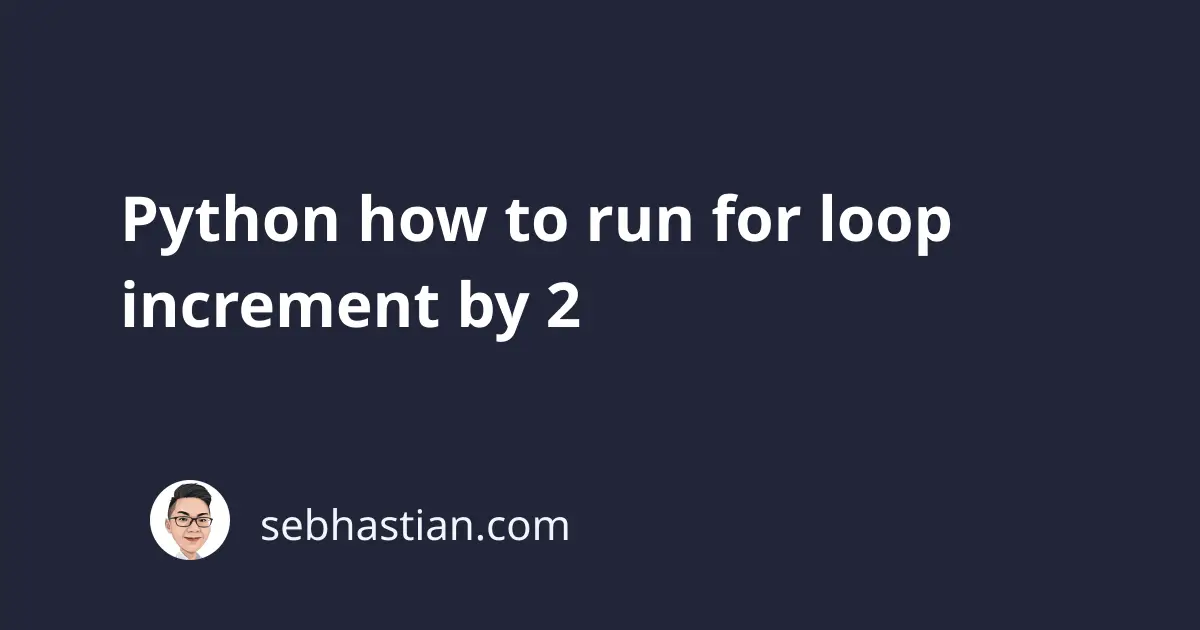
By default, the Python for
loop will increment the iteration counter by 1 in each iteration.
But in some unique cases, you might want to increment the counter by 2. Maybe you only need the odd numbers starting from 1.
This tutorial will show you three possible solutions to increment the for
loop by 2:
- Using the
range()
function - Using the slicing syntax
- With a list, use the
range()
andlen()
functions
Let’s see how the solutions work in practice.
1. Using the range() function
The range()
allows you to specify three parameters: start
, stop
, and step
.
To increment the counter by 2
for each iteration, you only need to pass the number 2
as the step
argument.
In the following example, the for
loop increments by 2 to get all the odd numbers from 1 to 10:
# Start at 1, stop at 10, increment by 2
for i in range(1, 10, 2):
print(i)
Output:
1
3
5
7
9
To adjust the incremental in each iteration, you only need to change the step
argument.
2. Using the slicing syntax
If you have a list variable, then you can use the slicing syntax to get a portion of that list.
The slice syntax is list[start:stop:step]
, so when you encounter a unique case where you need to skip the next item, you can put 2 as the step
argument.
Consider the example below:
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
for i in my_list[0::2]:
print(i)
Output:
1
3
5
7
9
The slice syntax [0::2]
will extract the list from the first index 0
to the end, with the step size (increment) by 2.
The resulting new list then contains [1, 3, 5, 7, 9]
which is printed using the for
loop.
3. With a list, use the range() and len() functions
As an alternative to the slicing syntax, you can also combine the range()
and len()
functions to loop over a list that increments by 2.
Here’s an example:
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
for i in range(1, len(my_list), 2):
print(i)
This solution uses the length of the list to determine the stop
argument of the range()
function.
In this case, the len()
function returns 10, so the range goes from 1 to 10, increments by 2.
The output is as follows:
1
3
5
7
9
Keep in mind that this solution only works if your list contains numbers. If you have other values like strings or booleans, the for
loop will ignore them.
Unless you have a strong reason, it’s better to use the slicing syntax because it takes the list values into account.
Conclusion
Now you’ve learned how to use the range()
function and the slicing syntax to create a for
loop that increments by 2.
I hope this tutorial is helpful. Happy coding! 👍