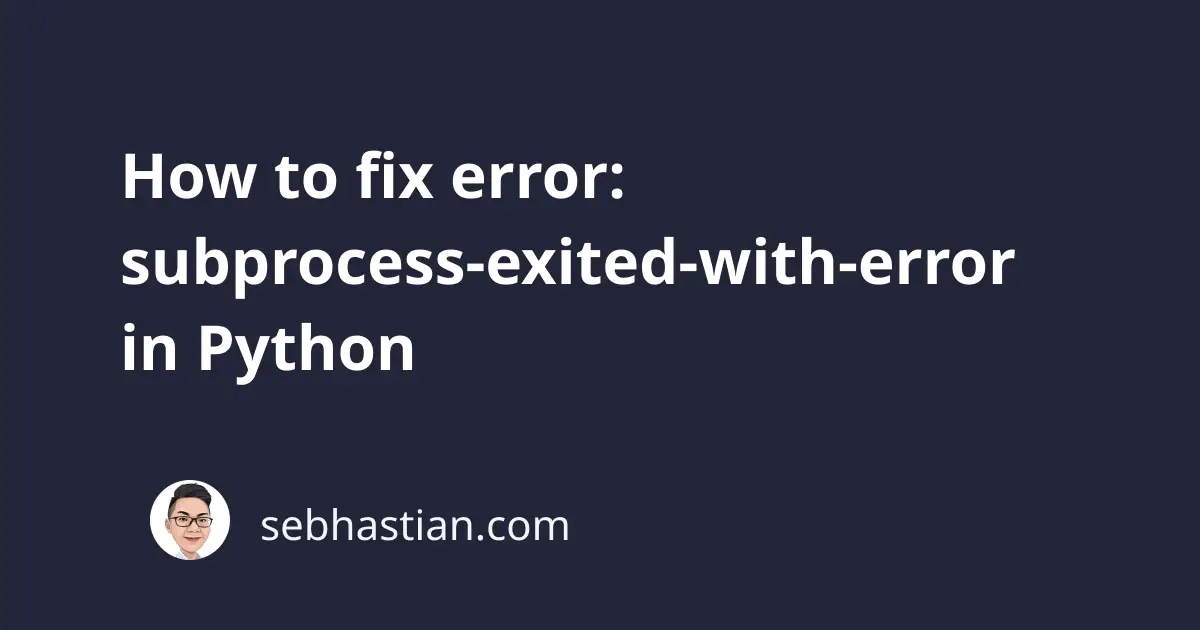
When running the pip install
command, you might encounter an error that says:
error: subprocess-exited-with-error
With some research (googling, of course!) I found this error usually occurs when pip
fails to execute the installation process of a package.
The most common causes for this error are:
- A required build tool is missing
- The package doesn’t support the operating system you’re using
- The package doesn’t support the latest Python version
- The package may require additional steps to install
The cause for this error is usually written in the log a few lines above or below it, so you need to read the standard output carefully.
This tutorial will help you to determine what causes the error and how you can fix it in practice.
1. A required build tool is missing
Sometimes, this error happens because a required build tool is missing from the computer.
For example, the installation of srsly
on my Windows computer shows the following output:
error: Microsoft Visual C++ 14.0 or greater is required. Get it with "Microsoft C++ Build Tools": https://visualstudio.microsoft.com/visual-cpp-build-tools/
[end of output]
note: This error originates from a subprocess, and is likely not a problem with pip.
error: subprocess-exited-with-error
If you’re getting the same error, then you need to install Microsoft Visual C++ Build Tools which you can download here.
Once you installed the tool, run the pip install
command again and see if it works.
If that doesn’t work, try upgrading pip, setuptools, and wheels:
# upgrade pip
pip install --upgrade pip
# or if you have pip3
pip3 install --upgrade pip
# if you don't have pip in PATH
python -m pip install --upgrade pip
python3 -m pip install --upgrade pip
# for Windows
py -m pip install --upgrade pip
# upgrade setuptools and wheel
pip install --upgrade setuptools wheel
pip3 install --upgrade setuptools wheel
python -m pip install --upgrade setuptools wheel
python3 -m pip install --upgrade setuptools wheel
py -m pip install --upgrade setuptools wheel
Once you upgraded these packages, try installing the package again.
2. The package doesn’t support the operating system you’re using
This error can happen when the package you’re trying to install doesn’t support the operating system you used.
For example, the text to speech TTS
package doesn’t support macOS on Apple Silicon chips, so this error occurs:
error: subprocess-exited-with-error
× Running setup.py install for mecab-python3 did not run successfully.
│ exit code: 1
The mecab-python3
package is required by TTS
and it doesn’t support Apple M chips at the moment.
Fortunately, the package can be installed using brew:
brew install mecab
Once mecab is installed, the TTS package can be installed successfully.
If you happen to have a similar error, you might be able to find a similar solution. Otherwise, there’s no way to fix this error except by using another Operating System.
3. The package doesn’t support the latest Python version
Some packages that are no longer maintained can cause this error when you try to install them on the latest version of Python.
You need to search the error message and see if you have a similar output as follows:
RuntimeError: Cannot install on Python version 3.11.2;
only versions >=3.7,<3.11 are supported.
In this case, there’s not much you can do but downgrade your Python version to the one supported by the package. Most likely, the library developers need more time to support the latest Python version.
Until then, you need to use another Python version. Using a virtual environment such as venv or conda should help you deal with this problem.
4. The package requires additional steps to install
This error might occur because the package requires additional steps to complete the installation process.
For example, here’s the output when I tried to install pysqlite3
:
pip3 install pysqlite3
...
Failed to build pysqlite3
Installing collected packages: pysqlite3
Running setup.py install for pysqlite3 ... error
error: subprocess-exited-with-error
× Running setup.py install for pysqlite3 did not run successfully.
After searching for more information, I found that this package needs to be linked to an SQLite database to be installed.
If I don’t want to link the package manually, I can install the binary package, which is statically linked with the most recent SQLite version.
pip install pysqlite3-binary
This time, the package is installed without any errors. You need to google for more information if your particular package has the same exceptions.
Conclusion
The error: subprocess-exited-with-error
occurs when Python fails to execute a subprocess successfully. Most likely, pip
encountered a problem when running the setup.py
script.
To resolve this error, you need to make sure the required build tools are installed, the package supports the operating system you used, and the Python version you used is supported.
I hope this tutorial is helpful. See you again in other tutorials! 👋