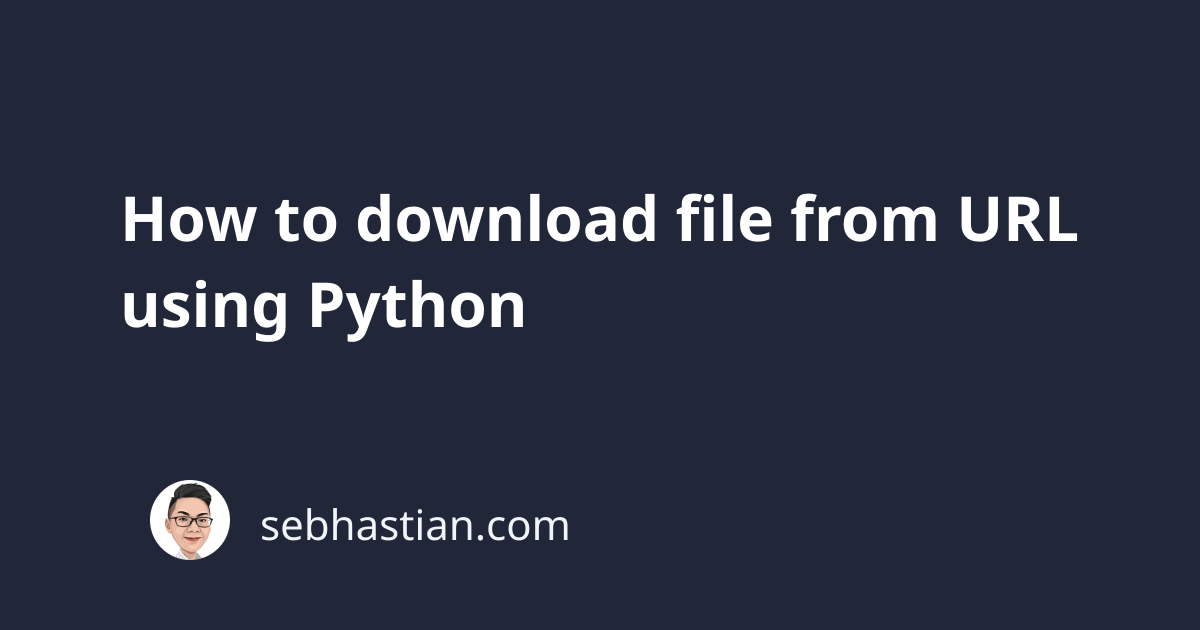
Python has incredible web scraping ability, so downloading a file from a URL is easy peasy using this programming language.
There are 3 simple ways you can download a file from a URL using Python:
- Use the
requests
module - Use the
wget
module - Use the
urllib
module
This tutorial will show you practical examples of using the modules to download a file from a URL.
1. Download a file using requests module
There’s a Python library named requests
that you can use to conveniently download a file from a URL.
First, install the library using pip
as follows:
pip install requests
# If you use pip3:
pip3 install requests
Once installed, you can use the module to download a file from a URL with three easy steps:
- Import the requests module
- Call the
requests.get()
method and pass the URL as its argument - Save the
response.content
usingopen()
andwrite()
functions.
Let’s use the library to download the GitHub favicon file. The icon URL is https://github.com/favicon.ico
so here’s how we do it:
import requests
url = "https://github.com/favicon.ico"
response = requests.get(url)
open("github.ico", "wb").write(response.content)
In the open()
function, you need to specify the name of the file. Here, the file is renamed from favicon.ico
to github.ico
.
The response.content
is then written in the new file. You should see the file in the same folder where you run the Python script.
2. Using the wget module
Besides using the requests
module, you can also use the wget
module to download a file from a URL.
First, install the wget
module using pip
:
pip install wget
# For pip3:
pip3 install wget
Next, you need to import wget
in your script and call the wget.download()
method. There are two arguments you need to pass:
- The file url - required
- The new name for the downloaded file - optional
If you didn’t pass the second argument, then the original file name will be used. See the example below:
import wget
url = "https://github.com/favicon.ico"
wget.download(url, "github.ico")
Run the code above, and you’ll see the github.ico
file downloaded in the same directory where you run the script.
3. Using the urllib module
The urllib
library is a built-in Python library for working with URLs. You can use this library to download a file from a URL.
Since it’s already included in Python, you don’t need to install anything. There are two steps to download a file using urllib
:
- import the
request
module fromurllib
- Call the
request.urlretrieve()
method, passing the URL and the new file name
Here’s an example of using the urllib
module:
from urllib import request
url = "https://github.com/favicon.ico"
request.urlretrieve(url, "github.ico")
With that, you should have the downloaded file appear in the same folder where you run the program.
Conclusion
In this tutorial you learned how to download a file from a URL using Python.
From the three libraries covered in this article, the requests
library is the most popular because it has many configurations for making GET, POST, PUT, DELETE, HEAD, OPTIONS, PATCH requests.
If you already use the library in your code, then it can be used to download a file from a URL. If not, then you can use wget
or urllib
to get the job done.
I hope this tutorial is helpful. Until next time! 😃