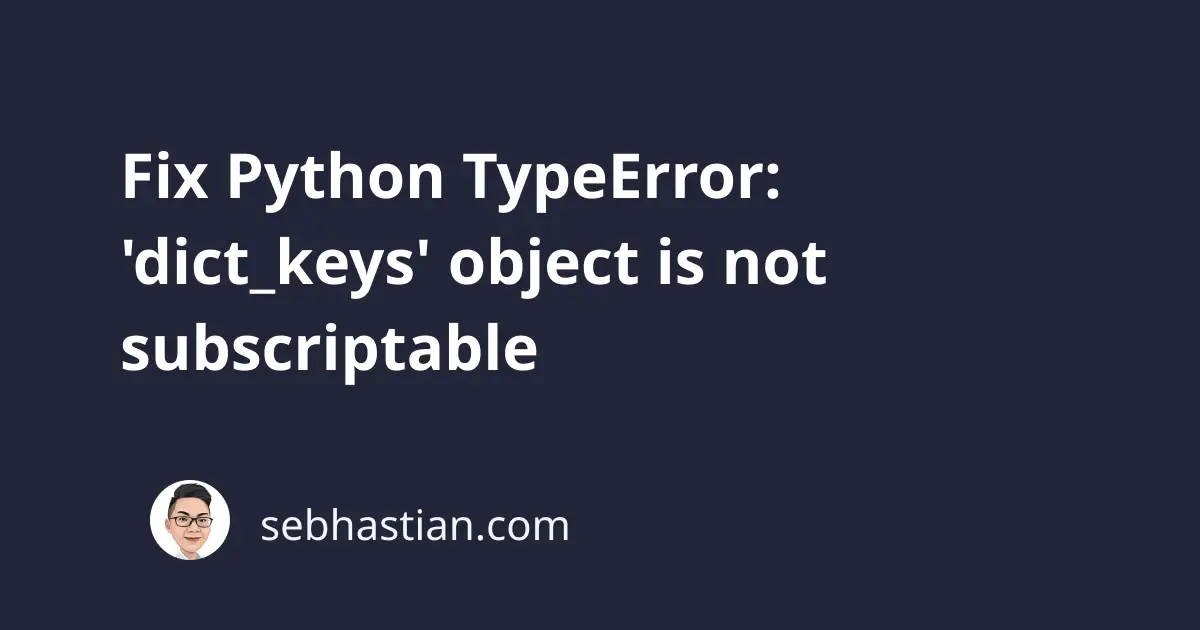
The Python error TypeError: 'dict_keys' object is not subscriptable
occurs when you try to access an element of a dict_keys
object using the subscript notation (e.g., dict_obj[0]
)
To fix the error, convert the dict_keys
object to a list such as in list(dict_obj.keys())[0]
.
Let’s see an example. Suppose you try to index or slice a dict_keys
object as follows:
items = {'a': 1, 'b': 2, 'c': 3}
keys = items.keys()
# ❌ Access keys with index notation
print(keys[0])
# ❌ Access keys with slice notation
print(keys[0:2])
The keys()
method of the dict
object returns an iterable object containing the dictionary key values. This object is called dict_key
object.
In Python version 3, the dict_key
object can’t be accessed like a list because it doesn’t implement the __getitem__
method which is called by the subscript notation internally.
The code above gives the following output:
Traceback (most recent call last):
File ...
print(keys[0])
TypeError: 'dict_keys' object is not subscriptable
To fix this error, you need to convert the dict_keys
object into a subscriptable object, such as a list or a tuple.
You can do this using the built-in list()
or tuple()
functions as shown in the code below:
items = {'a': 1, 'b': 2, 'c': 3}
keys = items.keys()
# convert dict_keys object to list
keys = list(keys)
print(keys[0]) # 'a'
print(keys[0:2]) # ['a', 'b']
# convert dict_keys object to tuple
keys = tuple(keys)
print(keys[0]) # 'a'
Alternatively, you can iterate over the dict_keys
object using a for
loop like this:
items = {'a': 1, 'b': 2, 'c': 3}
keys = items.keys()
# iterate over dict_keys object
for key in keys:
print(key)
Depending on how you want to use the dict_keys
object, you can choose between converting it to a subscriptable object, or iterating over the dict_keys
using a for
loop.
Conclusion
The dict_keys
object is an iterable object that represents the keys of a dict
object.
It is not a subscriptable object, which means you cannot access its elements using the subscript notation.
When you use a subscript notation such as indexing or slicing syntax, Python shows the TypeError: 'dict_keys' object is not subscriptable
error.
To fix this error, you need to convert the dict_keys
object into a subscriptable object like a list or a tuple. Following the examples in this article should help you fix the error.