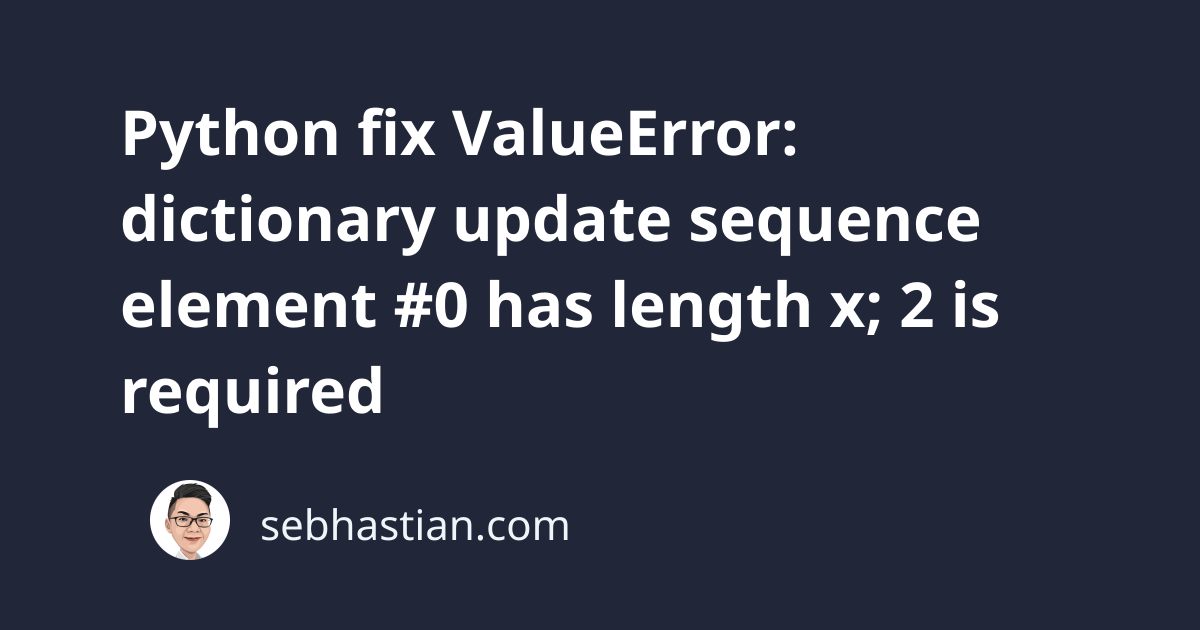
One error you may encounter when working with Python dictionaries is the update sequence error.
Here’s an example of this error:
Traceback (most recent call last):
File ...
my_dict.update("b")
ValueError: dictionary update sequence element #0 has length 1;
2 is required
This error usually occurs when you try to call the update()
method from a dictionary object.
The dictionary update()
method inserts the items you specified as its arguments into the dictionary.
To make the method runs smoothly, you need to ensure that you’re passing a key-value pair to the method.
How to reproduce the error
To reproduce the error, try to pass a single value to the update()
method as shown below:
my_dict = {"a": "Nathan"}
my_dict.update("b") # ❌
print(my_dict)
In the code above, a single string value b
is passed to the update()
method, causing the error.
How to fix the error
To fix this error, you need to pass the right argument to the update()
method.
For example, you can pass a dictionary as follows:
my_dict = {"a": "Nathan"}
my_dict.update({"b": "Anna"}) # ✅
print(my_dict) # {'a': 'Nathan', 'b': 'Anna'}
Aside from a dictionary, the update()
method also accepts an iterable object that has a key-value pair.
Here’s another example of passing a list to the method:
my_dict = {"a": "Nathan"}
my_dict.update([["b", "Anna"]]) # ✅
print(my_dict) # {'a': 'Nathan', 'b': 'Anna'}
You can also pass a list of tuples to the update()
method like this:
my_dict = {"a": "Nathan"}
my_dict.update([("b", "Anna"), ("c", "Joy")]) # ✅
print(my_dict)
# {'a': 'Nathan', 'b': 'Anna', 'c': 'Joy'}
On rare occasions, you will also see this error when you convert a string to a dictionary.
For example, suppose you want to convert the following string into a dictionary:
my_str = "{'a': 'Nathan', 'b': 'Lori'}"
my_dict = dict(my_str)
# ❌ ValueError: dictionary update sequence element #0
# has length 1; 2 is required
To solve this error, you need to first call the literal_eval()
function to evaluate the string expression.
This is done so that Python can identify that the string resembles a dictionary and convert it accordingly:
from ast import literal_eval
my_str = "{'a': 'Nathan', 'b': 'Lori'}"
my_dict = dict(literal_eval(my_str))
print(my_dict) # {'a': 'Nathan', 'b': 'Lori'}
Now you’ve learned how to solve Python ValueError: dictionary update sequence element #0 has length x; 2 is required
.
Always remember that when you call the dictionary update()
method, you need to pass the right amount of elements in a key-value pair structure.
Cheers! 🍻