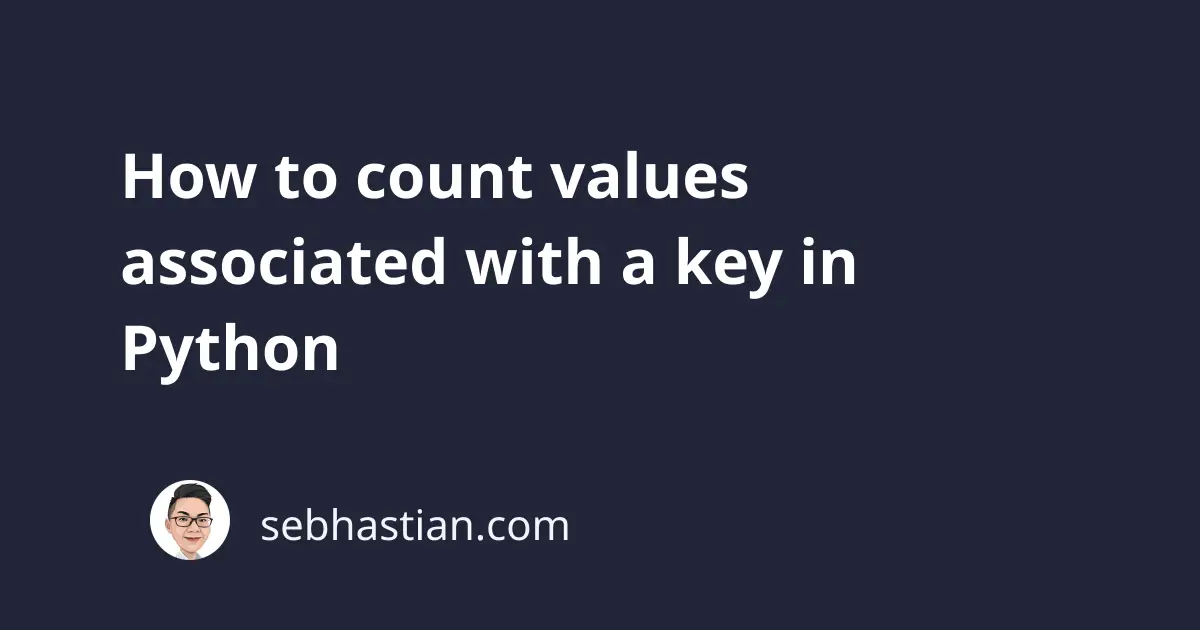
To count how many values per key in a Python dictionary object, you need to use a for
loop and the items()
method to iterate over the dictionary object.
In each iteration, you can count the values using the len()
function.
For example, suppose you have a dictionary object with list values as follows:
my_dict = {
1: ["a", "b", "c"],
2: ["z", "x"],
3: ["q", "w", "e", "r"]
}
To iterate over the dictionary, use a for
loop and the items()
method as follows:
my_dict = {
"key_1": ["a", "b", "c"],
"key_2": ["z", "x"],
"key_3": ["q", "w", "e", "r"]
}
for key, value in my_dict.items():
print(f"{key} has {len(value)} items")
Inside the loop, you need to call the print()
function and get the length of the list using the len()
function.
The output is as follows:
key_1 has 3 items
key_2 has 2 items
key_3 has 4 items
This solution works even when you have other types that can contain multiple values, such as a tuple or a set.
If you have empty strings in your value and you want to exclude it from the count, you need to use the filter()
function to filter out values that evaluate to False
as follows:
my_dict = {
"key_1": ["a", "b", "c", ""],
"key_2": ["z", "", "x"],
"key_3": ["q", "w", "", "r", ""]
}
for key, value in my_dict.items():
count = len(list(filter(bool, value)))
print(f"{key} has {count} items")
The filter()
function returns a filter object, so you need to call the list()
function to convert it again to a list.
As a result, the empty strings will be excluded from the count
value.
I hope this tutorial helps. See you in other tutorials! 👋