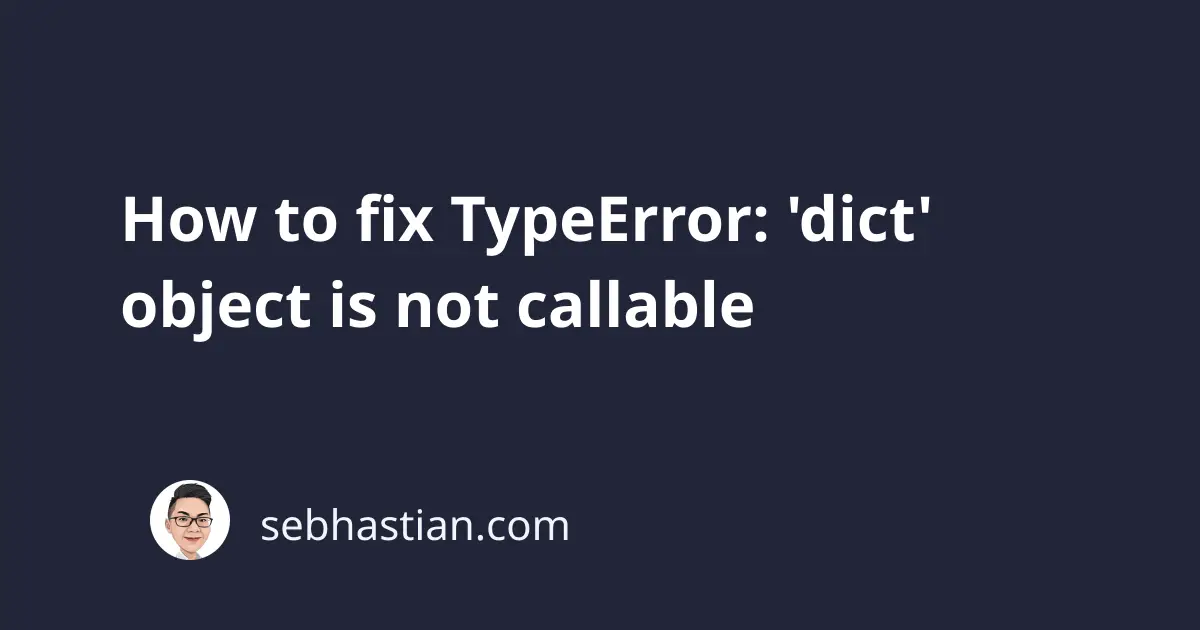
When you’re working with dictionaries in Python, you might see the following error:
TypeError: 'dict' object is not callable
This error usually occurs when you use round brackets ()
to access a dictionary value. You need to use square brackets []
when accessing a value from a dictionary.
Let’s see an example that causes this error and how to fix it
Why this error occurs
Suppose you created a dictionary object in your code as follows:
dic = {1: "Nathan", 2: "Jane", 3: "John"}
Then, you proceed to access the value at key 1
, so you write the code below:
dic(1)
But the round brackets ()
are used to call a function in Python.
Since a dictionary isn’t a function, you get this error:
Traceback (most recent call last):
File "main.py", line 2, in <module>
dic(1)
TypeError: 'dict' object is not callable
The ‘dict’ object in the message refers to the dic
variable that we’ve created.
By adding parentheses, Python thinks you’re trying to call a function, but a dictionary is not a function!
How to fix this error
To fix this error, you need to access a dictionary value by using square brackets.
Here’s the right syntax:
dic = {1: "Nathan", 2: "Jane", 3: "John"}
dic[1] # Nathan
If you use strings as the keys to the dictionary, then you can pass the key in the square brackets like this:
dic = {"person_1": "Nathan", "person_2": "Jane", "person_3": "John"}
print(dic["person_1"]) # Nathan
print(dic["person_2"]) # Jane
By appropriately using the square brackets, you can access the values contained in the dictionary object and avoid the error.
I hope this tutorial is helpful. See you in other articles! 🙌