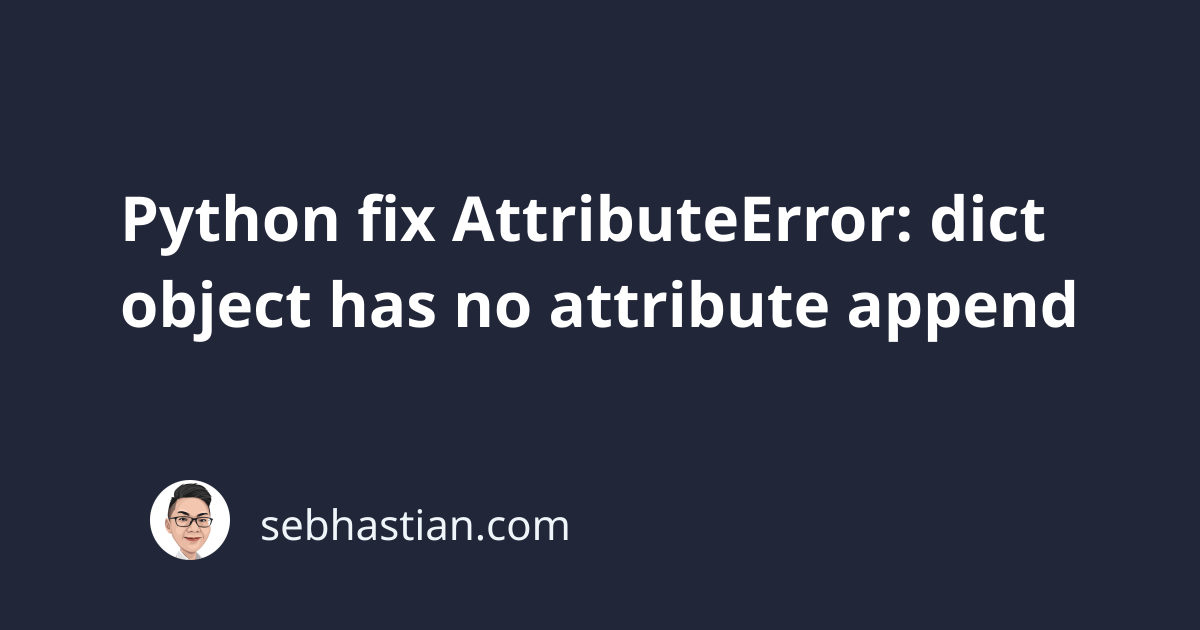
You will get AttributeError: dict object has no attribute append
in Python when you call the append()
method on a dictionary object.
To fix this error, you need to call the append()
method on a list instead of a dictionary.
To add an element to a dictionary object, you need to use the index operator using square brackets instead.
What AttributeError: dict object has no attribute append means
The Python AttributeError: dict object has no attribute append
occurs when you call the append()
method on a dictionary object.
A dictionary object is defined by using the curly brackets as follows:
val = {'a': 10, 'b': 20}
val.append(30)
# ❌ AttributeError: dict object has no attribute append
In the code above, the val
variable is assigned a dictionary object as its value.
Calling the append()
method on the variable will produce the following error:
Traceback (most recent call last):
File ...
val.append()
AttributeError: 'dict' object has no attribute 'append'
Let’s see how to fix this error next.
How to fix AttributeError: dict object has no attribute append
If you mean to add a value to the dictionary object, you need to add it using the square brackets notation.
Here’s the right way to add a value to a dictionary in Python:
val = {'a': 10, 'b': 20}
val['c'] = 30
print(val) # {'a': 10, 'b': 20, 'c': 30}
# Access the value in key c
print(val['c']) # 30
In the code above, the value 30
is added to the val
dictionary under the key c
.
When you need to access the value later, you use the same square brackets notation.
The append()
method is used when you want to add elements to a list object.
A list object can be defined using the square brackets. The following example shows how to create a list in Python:
my_list = [10 , 20]
# Append new elements to the list
my_list.append(30)
my_list.append(40)
print(my_list) # [10, 20, 30, 40]
A list object also doesn’t require a key-value pair to be defined for each element stored in it.
Depending on what you want to achieve, you need to adjust your code so that the append()
method is called only on list objects.
Now you’ve learned how to fix the Python AttributeError: dict object has no attribute append
. Nice! 👍