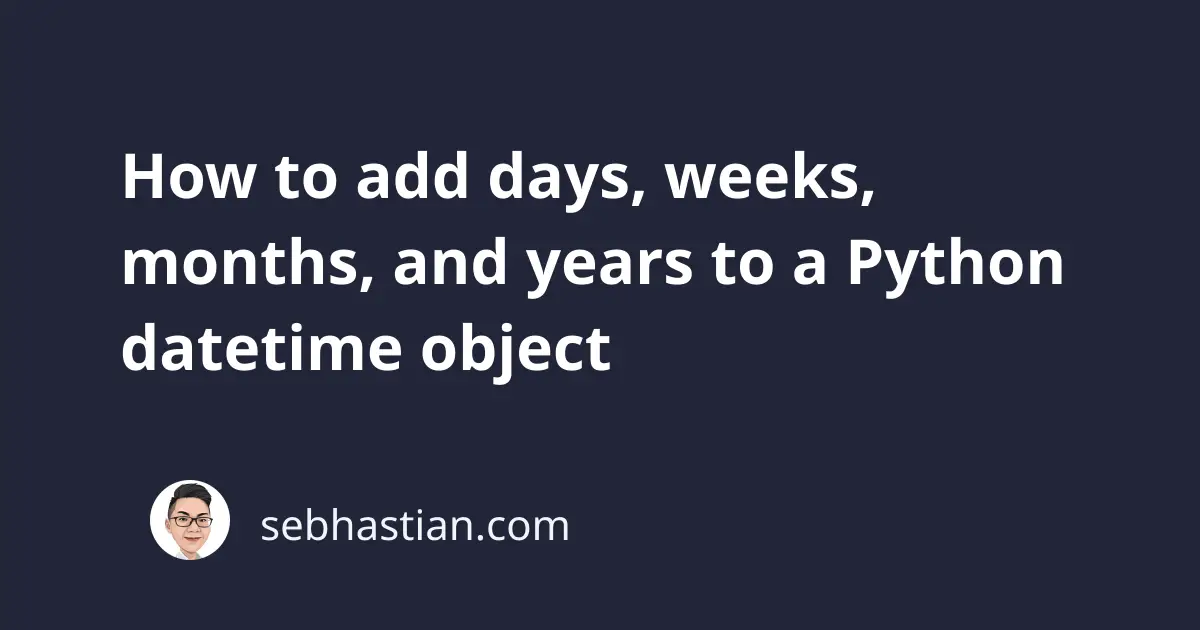
The Python datetime
module is a comprehensive date and time module that allows you to manipulate dates in your program.
The datetime
module has a timedelta
class that’s used to represent a duration of time. This duration can then be added or subtracted from a datetime
object using arithmetic operators.
In this tutorial, you’re going to learn how to add days, weeks, months, and years to a datetime
object in practice.
1. Adding days to datetime in Python
To add days to a date, you need to create a timedelta
object and add it to your existing datetime
object.
Here’s an example of adding 3 days to a datetime
object:
from datetime import datetime, timedelta
my_datetime = datetime(2023, 3, 2)
print(my_datetime) # 2023-03-02 00:00:00
# specify the days argument in timedelta
new_datetime = my_datetime + timedelta(days=3)
print(new_datetime) # 2023-03-05 00:00:00
To add days to a datetime
object, you need to create a timedelta
object and pass the days
argument so that the object represents the duration of days.
2. Adding weeks to a date in Python
To add weeks to a Python date, you need to specify the weeks
argument when creating the timedelta
object.
Here’s an example of adding 2 and 3 weeks respectively:
from datetime import datetime, timedelta
datetime_1 = datetime(2023, 3, 2)
print(datetime_1) # 2023-03-02 00:00:00
# specify the weeks argument in timedelta
datetime_2 = datetime_1 + timedelta(weeks=2)
print(datetime_2) # 2023-03-16 00:00:00
# adding 3 weeks:
datetime_3 = datetime_1 + timedelta(weeks=3)
print(datetime_3) # 2023-03-23 00:00:00
You can also pass the days
argument multiplied by 7 for each week. But hey, the object already has the weeks
argument so might as well use it!
3. Adding months to a date in Python
If you want to add months to a Python datetime
object, then you need to use another class called relativedelta
from the dateutil
module.
The relativedelta
class allows you to create a duration of months
and years
that you can use to manipulate a datetime
object.
Here’s an example of adding 1 and 2 months to a date:
from datetime import datetime
from dateutil.relativedelta import relativedelta
datetime_1 = datetime(2023, 3, 2)
print(datetime_1) # 2023-03-02 00:00:00
# specify the months argument in relativedelta
datetime_2 = datetime_1 + relativedelta(months=1)
print(datetime_2) # 2023-04-02 00:00:00
# adding 2 months:
datetime_3 = datetime_1 + relativedelta(months=2)
print(datetime_3) # 2023-05-02 00:00:00
The relativedelta
class is very convenient because a month can have different days.
If you use the timedelta
class, you need to calculate the different days each month has and sum them yourself.
4. Adding years to a date in Python
To add years to a date in Python, you can use the timedelta
or relativedelta
class depending on your preferences.
Here’s an example of adding one year to a date using timedelta
and relativedelta
:
from datetime import datetime, timedelta
from dateutil.relativedelta import relativedelta
datetime_1 = datetime(2023, 3, 2)
print(datetime_1) # 2023-03-02 00:00:00
# 1 year with timedelta
datetime_2 = datetime_1 + timedelta(days=365)
print(datetime_2) # 2024-03-01 00:00:00
# 1 year with relativedelta
datetime_3 = datetime_1 + relativedelta(years=1)
print(datetime_3) # 2024-03-02 00:00:00
Note that there’s one day difference between using timedelta
and relativedelta
. This is because 2024 is a leap year, and the month of February in that year has 29 days.
The timedelta
object won’t add this logic to the calculation, while relativedelta
has taken leap years into account.
Conclusion
Both timedelta
and relativedelta
classes can be used to add a duration of time to your datetime
object.
The timedelta
class is limited to adding weeks to your date, while relativedelta
can also add months and years.
In fact, you can use relativedelta
to add days and weeks as well. But most people prefer timedelta
because it’s in the same module as the datetime
class. You don’t need to add another import
line.
Now you’ve learned how to add days, weeks, months, and years to a Python datetime
object.
I hope this tutorial is helpful. Until next time! 👋