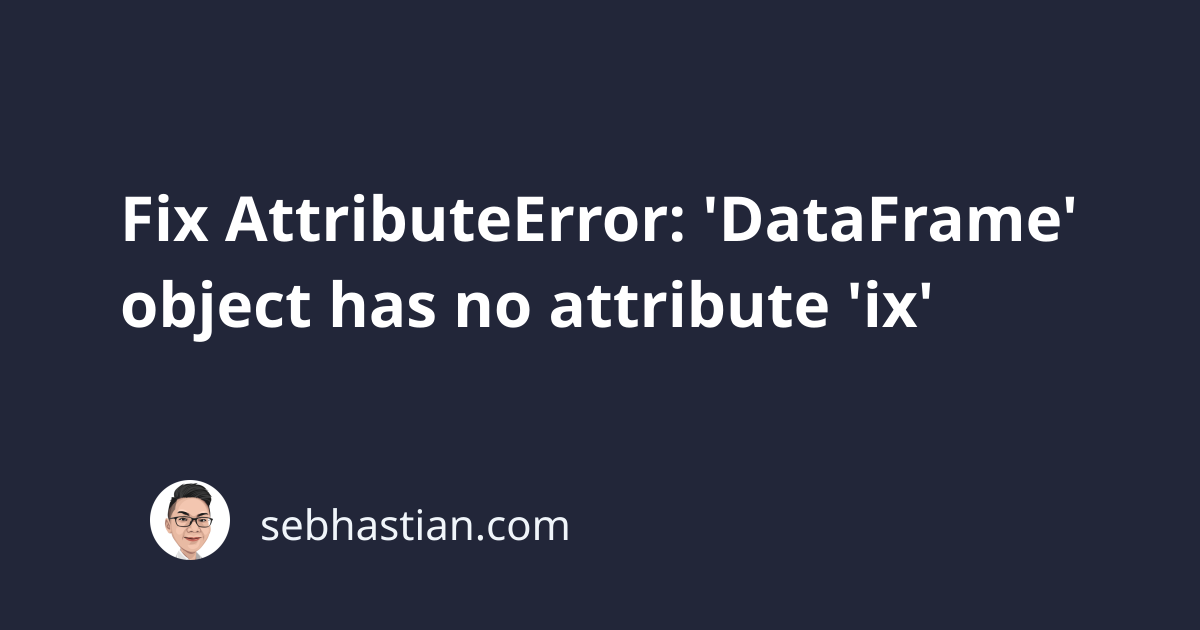
The Pandas module is a popular Python module used for data analysis purposes.
When working with a Pandas DataFrame object, you may encounter an error that says:
AttributeError: 'DataFrame' object has no attribute 'ix'
You get this AttributeError: 'DataFrame' object has no attribute 'ix'
because the ix
method has been deprecated and removed in the latest Pandas version.
The following article will show you how to fix this error.
How to reproduce the error
For example, imagine you have the following DataFrame in your Python project:
Age Score
Nathan 29 72
Lisa 25 88
Mike 22 67
Now you are tasked to get the first two rows of the data.
If you use the ix
method as follows:
import pandas as pd
df = pd.DataFrame(
{"Age": [29, 25, 22], "Score": [72, 88, 67]},
index=["Nathan", "Lisa", "Mike"]
)
print(df.ix[:2])
But the ix
method has been deprecated and removed in Pandas version 1.0.0, so running the code above will produce the error below:
Traceback (most recent call last):
File ...
print(df.ix[:2])
...
return object.__getattribute__(self, name)
AttributeError: 'DataFrame' object has no attribute 'ix'
To fix this error, you need to replace the ix
method with either iloc
or loc
method.
Let’s see an example of using these methods next!
Replace ix with iloc or loc
The iloc
method is used to access a row by its integer index, so you can use it like this:
import pandas as pd
df = pd.DataFrame(
{"Age": [29, 25, 22], "Score": [72, 88, 67]},
index=["Nathan", "Lisa", "Mike"]
)
print(df.iloc[:2])
# Output:
# Age Score
# Nathan 29 72
# Lisa 25 88
On the other hand, the loc
method is used for label-based indexing.
Because the example data has the index
parameter, you can use the value for label-based slicing as follows:
import pandas as pd
df = pd.DataFrame(
{"Age": [29, 25, 22], "Score": [72, 88, 67]},
index=["Nathan", "Lisa", "Mike"]
)
print(df.loc["Lisa"])
# Output
# Age 25
# Score 88
# Name: Lisa, dtype: int64
Keep in mind that when you pass the index
parameter to the iloc
method, Python will show another error.
Previously, the ix
method can process both label-based and integer-based indexing. It will first process label-based indexing, then fall back to integer-based indexing when it fails.
But because the ix
method wasn’t consistent, it was deprecated in favor of loc
and iloc
methods for slicing and indexing.
If you still want to use the ix
method for indexing, then you need to downgrade your pandas
module to below version 1.0.0
.
Conclusion
Now you’ve learned why the AttributeError: 'DataFrame' object has no attribute 'ix'
occurs and how to fix it.
To avoid this error, you need to replace the call to ix
method with either iloc
or loc
method.
Good work solving the error. I look forward to seeing you next time! 👋