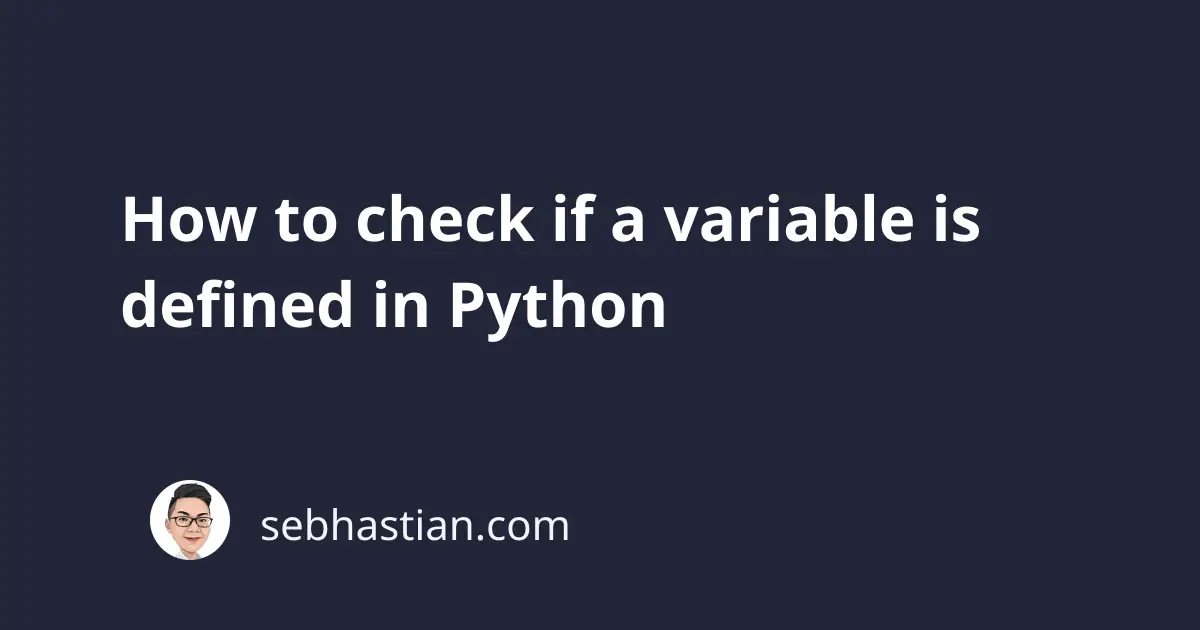
Python expects any variable you declared to be defined with a value.
This is different from another language like, say, JavaScript, where you can declare a variable without defining a value for that variable.
This example code works in JavaScript:
let my_string
if (my_string == undefined){
my_string = 'Hello'
}
console.log(my_string)
But in Python, declaring a variable without assigning any value will result in an error:
my_string
if my_string is None:
my_string = "Hello"
print(my_string)
The code above raise the following error:
Traceback (most recent call last):
File "main.py", line 1, in <module>
my_string
NameError: name 'my_string' is not defined
The error originates from line 1, in which we declared the my_string
variable without assigning any value.
You’re not allowed to define a variable without assigning any value in Python. If you have no real value, then you can assign the None
object:
my_string = None
if my_string is None:
my_string = "Hello"
print(my_string)
This is useful when your variable only has a meaningful value when a certain condition is met.
You can certainly check if a variable is defined or not using a try-except
block as follows:
try:
print(my_string)
except:
print("my_string is not defined")
my_string = "hello"
The try-except
block as shown above prevents a runtime error from happening when the my_string
variable is undefined.
This is useful when you’re not sure whether the variable is already defined or not. You are then free to define the variable in the except
block.
I hope this tutorial is helpful. See you in other tutorials! 🙌