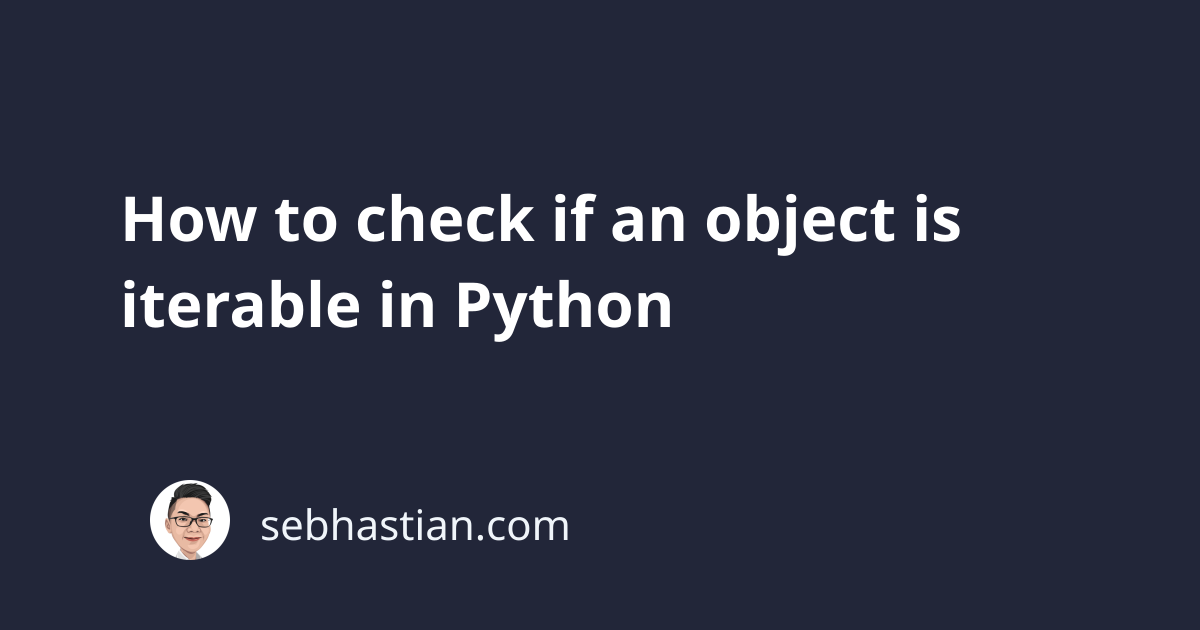
An iterable object is any object that can be iterated over using a for
loop. Some examples of iterable objects in Python are strings, lists, and tuples.
When developing with Python, you may get a variable or custom object, but you don’t know if it’s iterable or not.
Knowing if a given object is iterable or not is important because Python will raise a TypeError
when you try to iterate over a non-iterable object.
In this article, you will learn different ways to check if an object is iterable in Python.
1. Using the iter()
function
The iter()
function takes an object as an argument and returns an iterator object if the object is iterable.
Under the hood, the iter()
function checks if the object has __iter__()
or __getitem__()
method implemented. If not, the function will return a TypeError
.
You need to wrap the call to this function in a try-except
block as follows:
list = [1, 2, 3]
try:
iterable = iter(list)
print("The object is iterable")
except TypeError:
print("The object is not iterable")
Since the list
object is iterable, Python won’t execute the except
block.
2. Using the isinstance() function
You can also use the isinstance()
function together with the Iterable
class to check if an object is iterable or not.
This function takes two parameters: an object and a type.
The function returns True
if the object is an instance of the given type. Otherwise, it returns False
.
Here’s an example of using the isinstance()
function to check if a string is an instance of the Iterable
class:
from collections.abc import Iterable
my_string = "Nathan"
is_iterable = isinstance(my_string, Iterable)
print(is_iterable) # True
Note that you need to import the Iterable
class from the collections.abc
module.
Also, this solution is less preferred than the iter()
function because the Iterable
class only checks for the modern __iter__()
method while ignoring the existence of the __getitem__()
method in the object.
If you don’t need to support old versions of Python, then using this solution may not cause any issues.
3. Make checking easy with isiterable()
If you have many variables and objects with unknown data types, it will be inconvenient to check the objects one by one.
I recommend you create a custom function that returns True
when an object is iterable or False
otherwise.
You can name the function as isiterable()
:
def isiterable(obj):
try:
iterable = iter(obj)
return True
except:
return False
Anytime you want to check if an object is iterable, you just call the function as follows:
# Test 1
score = 90
if isiterable(score):
print("Object is iterable")
else:
print("Object is not iterable") # ✅
# Test 2
my_list = [1, 2, 3]
if isiterable(my_list):
print("Object is iterable") # ✅
else:
print("Object is not iterable")
The isiterable()
function will make checking for iterable objects convenient and easy. 😉
Conclusion
This article has shown different ways to check if an object is iterable in Python.
You can use the iter()
function and a try-except
block, or isinstance()
and an if-else
block.
Knowing how to check if an object is iterable can be useful to avoid TypeError
when you do a for
loop or a list comprehension with the object.
I hope this article was helpful. See you again in other articles! 👋