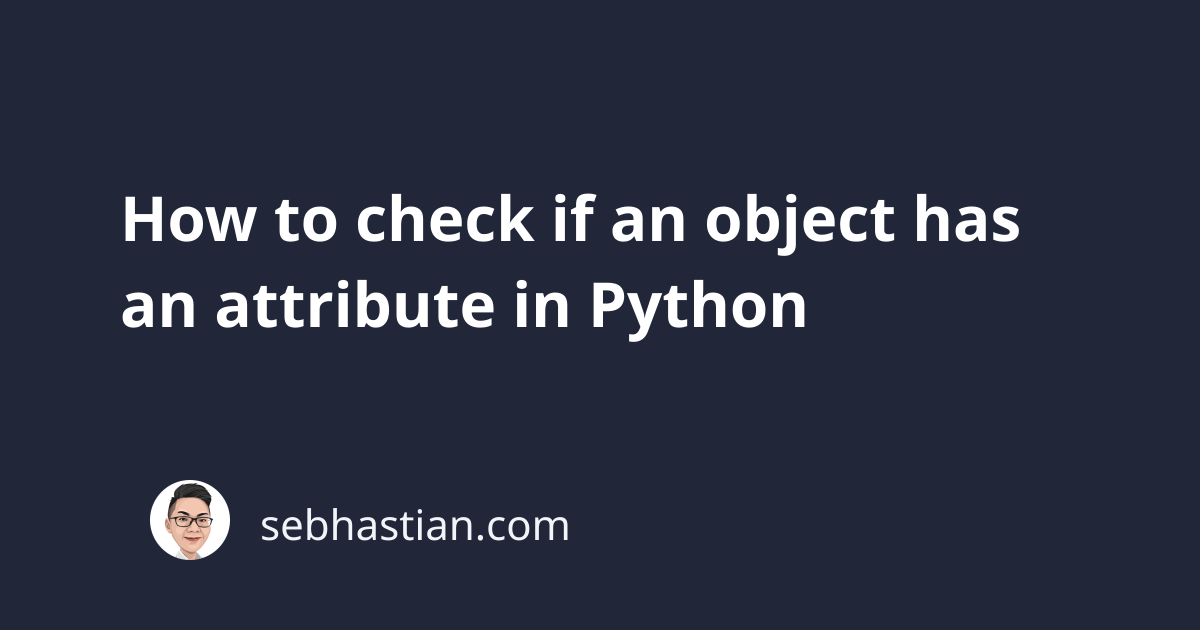
To check if an object has a certain attribute or not, you can use the hasattr()
function.
When calling the hasattr()
function, you need to pass 2 parameters: the object and the attribute name as a string.
When the attribute exists, the function returns True
. Otherwise, it returns False
.
Here’s an example of checking if a string has an attribute called join
:
my_str = 'ABC'
print(hasattr(my_str, 'join')) # True
You can also check if an object instantiated from a class has a certain attribute.
This example checks if the my_car
object has an attribute called brand
and wheels
:
class Car:
def __init__(self):
self.brand = 'Tesla'
self.year = 2002
my_car = Car()
print(hasattr(my_car, 'brand')) # True
print(hasattr(my_car, 'wheels')) # False
As you can see, the hasattr()
function output can be used to further your code by including conditionals such as an if
statement.
I hope this tutorial helps. Happy coding!