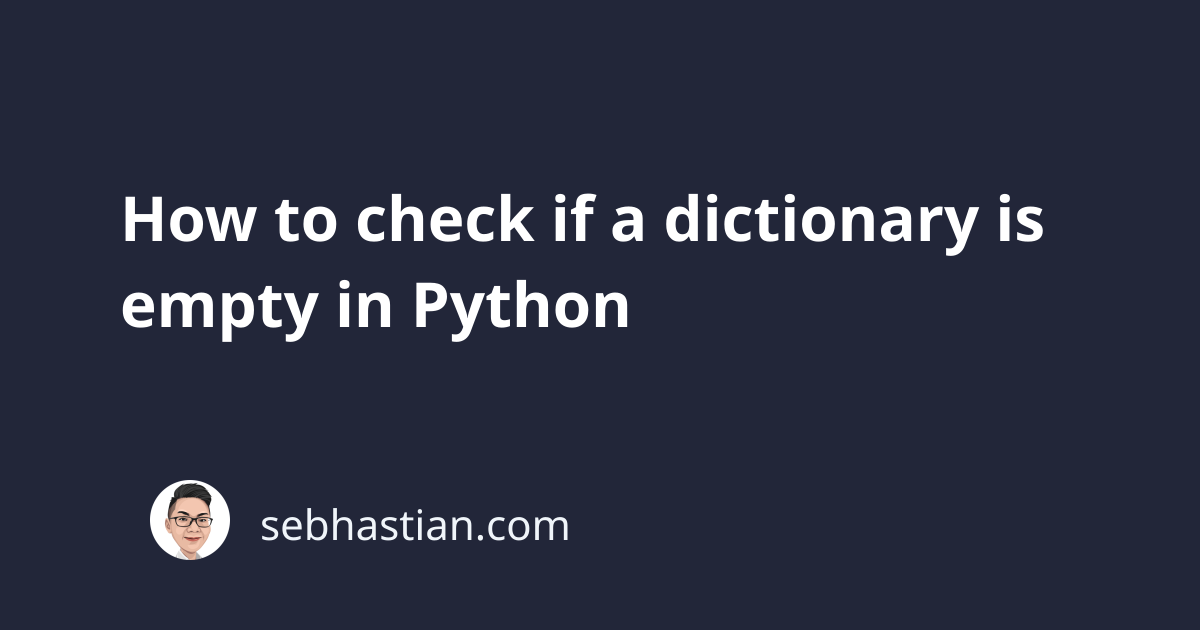
There are three ways you can check for an empty dictionary in Python:
- Use the if-else statement
- Use the
len()
function - Use the equality comparison
==
operator
This tutorial shows how you can use the three solutions above in practice.
1. Use the if-else statement
To check if a dictionary is empty in Python, you can use an if
statement followed by the dictionary variable name.
An empty dictionary will evaluate to a boolean False
when empty, so the if
statement below only runs when the dictionary is not empty:
dict1 = {}
if dict1:
print("Dictionary is not empty")
else:
print("Dictionary is empty") # ✅
Output:
Dictionary is empty
Because the dictionary is empty, the else
statement is executed.
2. Use the len() function
You can also check for an empty dictionary by using the len()
function.
The len()
function returns the length of the dictionary object you passed as its argument, which means it shows how many key-value pairs you have in the dictionary.
To check if the dictionary is empty, check if the object’s length is greater than 0
as follows:
dict1 = {"name": "Nathan"}
if len(dict1) > 0:
print("Dictionary is not empty") # ✅
else:
print("Dictionary is empty")
Again, the if
statement is only executed when the dict1
object is not empty.
3. Use the equality comparison == operator
The third way you can check if a dictionary is empty is by using the equality comparison ==
operator.
Use this operator and compare the object with an empty dictionary {}
as follows:
dict1 = {"name": "Nathan"}
if dict1 == {}:
print("Dictionary is empty")
else:
print("Dictionary is not empty") # ✅
This check is usually the best as you exactly need an empty dictionary to trigger the if
statement.
When you use the if dict1
statement, the evaluation returns True
when you pass a truthy value, such as True
or any integer except 0
:
dict1 = True
if dict1:
print("Dictionary is not empty") # ✅
else:
print("Dictionary is empty")
Even though the dict1
object above is not a dictionary, the if
statement is executed anyway because it evaluates to True
.
By using the equality comparison ==
operator, the object must exactly be an empty dictionary to trigger the if
statement.
I hope this tutorial is helpful. Happy coding! 👍