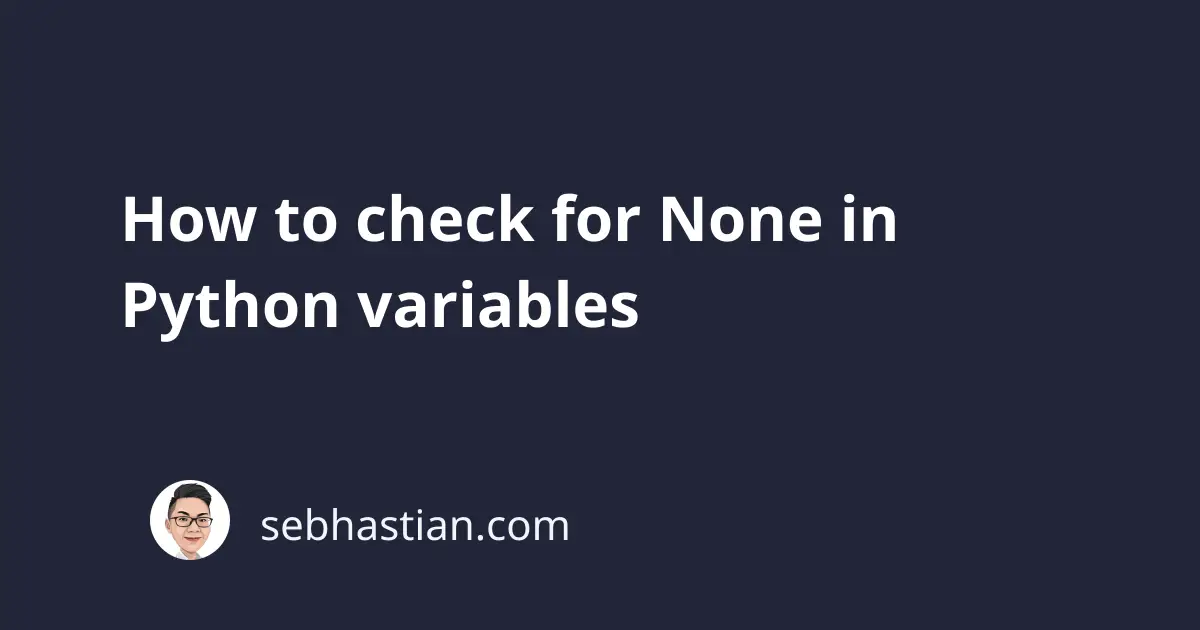
There are three ways you can check for a None
value in Python:
- Using the
is
operator keyword - Using the equality operator
==
- Using the
isinstance()
function
The None
value in Python is used to signify an “empty” value. It’s similar to the NULL
value in other programming languages.
This tutorial shows how to use the three methods mentioned above to check if a variable is None
.
1. Using the is
operator keyword
The is
operator keyword is used to check if the two operands surrounding the operator refer to the same object or value.
This operator can be used to check if a variable has a None
value. Suppose you want to check the value of the x
variable as follows:
x = "abc"
result = x is None
print(result) # False
The is
operator returns True
when the two operands refer to the same object. Otherwise it returns False
.
You can use this operator in an if
statement to check for the value of a variable:
x = "abc"
if x is None:
print("Value of x is None")
else:
print("x is not None")
The else
statement is optional, and you can omit it if you want the program to do nothing when the variable value is not None
.
2. Using the equality ==
operator
The equality ==
operator checks whether the two operands surrounding the operator are inherently equal.
Like the is
operator, you can combine this operator with an if
statement to check whether a variable contains None
:
x = "abc"
if x == None:
print("Value of x is None")
else:
print("x is not None")
However, most people would recommend you to use is
instead of ==
because the is
keyword is faster than the equality operator.
The is
keyword doesn’t check for the value of the operands. It simply sees if the two operands point to the same object.
You can test this by comparing two empty arrays as follows:
x = []
y = []
print(x is y) # False
print(x == y) # True
Although both variables have the same value, they are pointing to different objects, so the is
keyword returns False
while the equality operator returns True
.
3. Using the isinstance()
function
The isinstance()
function is used to check whether an object is an instance of a specific class.
The function returns true
when the object is an instance of the class you specified. You can use this function to check if a variable is None
as follows:
x = None
res = isinstance(x, type(None))
print(res) # True
The isinstance()
function can be used as an alternative when you need to check whether a variable is assigned a None
value.
Conclusion
This tutorial shows you three simple and effective ways to check whether a variable is None
or not. The is
keyword is the most simple and straightforward, and it’s the most popular way to check for a None
value.
I hope this tutorial helps. See you in other tutorials! 🙌