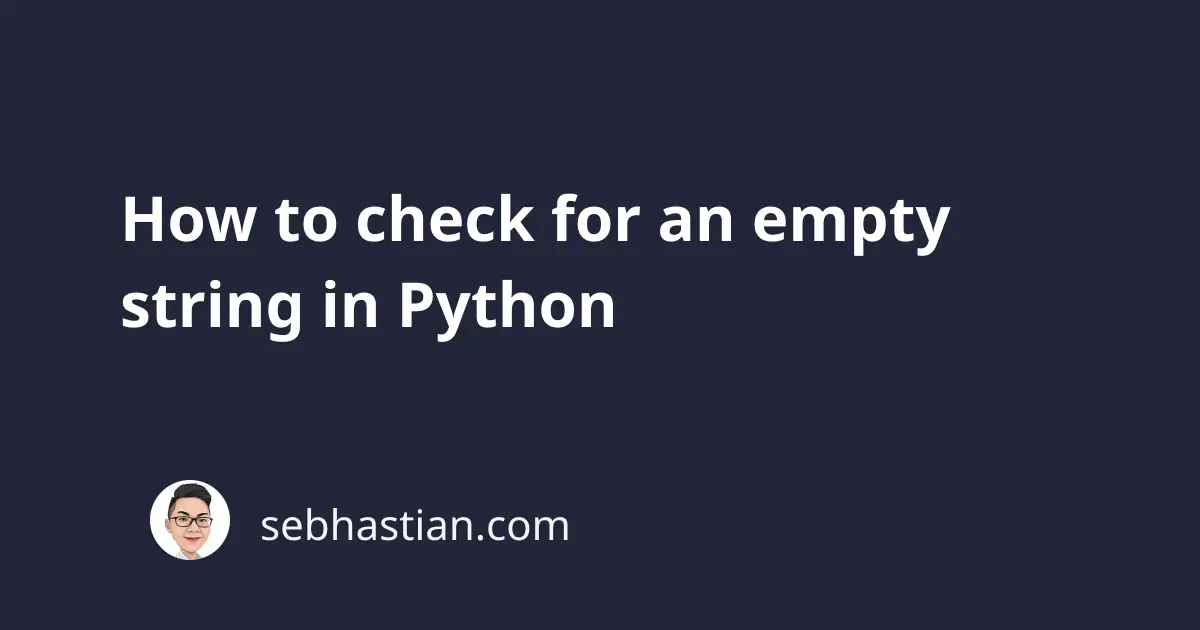
There are four ways you can check if a string is empty or not in Python:
- Use an
if
statement - Use an
if not
statement - Compare the
len()
result to0
- Use the
strip()
method
This tutorial shows how you can use each solution in practice
1. Use an if statement
An empty string evaluates to the boolean value False
internally, so you can use an if
statement to check if a string is empty.
The if
statement block below runs only when the string is not empty:
my_str = ""
if my_str:
print("My string is not empty")
else:
print("String is empty")
When the string is empty, then the else
block gets executed.
This if/else statement also works when the string is None
as follows:
my_str = None
if my_str:
print("My string is not empty")
else:
print("String is empty") # ✅
This is the most straightforward way to check if a string is empty in Python
2. Use an if not statement
The if not
statement can be used to check for an empty string in Python.
The main difference between if
and if not
is that the if not
statement executes the if
block when the string is indeed empty:
my_str = ""
if not my_str:
print("String is empty") # ✅
else:
print("My string is not empty")
In this solution, the else
statement is executed only when the string is not empty.
3. Compare the len() result to 0
You can also check if a string is empty by comparing the len()
result to 0
.
An empty string has a length of 0
, so you can use the equality comparison ==
operator as follows:
my_str = ""
if len(my_str) == 0:
print("String is empty") # ✅
else:
print("My string is not empty")
4. Use the strip() method
Keep in mind that Python considers a string as empty only when there’s no character between the quotations.
If you have a white space character in the string, then Python will think it’s not an empty string. In the example below, the my_str
variable has three white spaces:
my_str = " "
if my_str:
print("My string is not empty") # ✅
else:
print("String is empty")
Python runs the if
block because it considers the string as not empty.
You can call the str.strip()
method to remove white spaces in your string as follows:
my_str = " "
if my_str.strip():
print("My string is not empty")
else:
print("String is empty") # ✅
Because the strip()
method removes any leading and trailing white spaces, the returned string will be empty.
Depending on what you want to achieve, sometimes you might need to consider a string filled with spaces as empty.
Now you’ve learned how to check for an empty string in Python. Good work! 👍