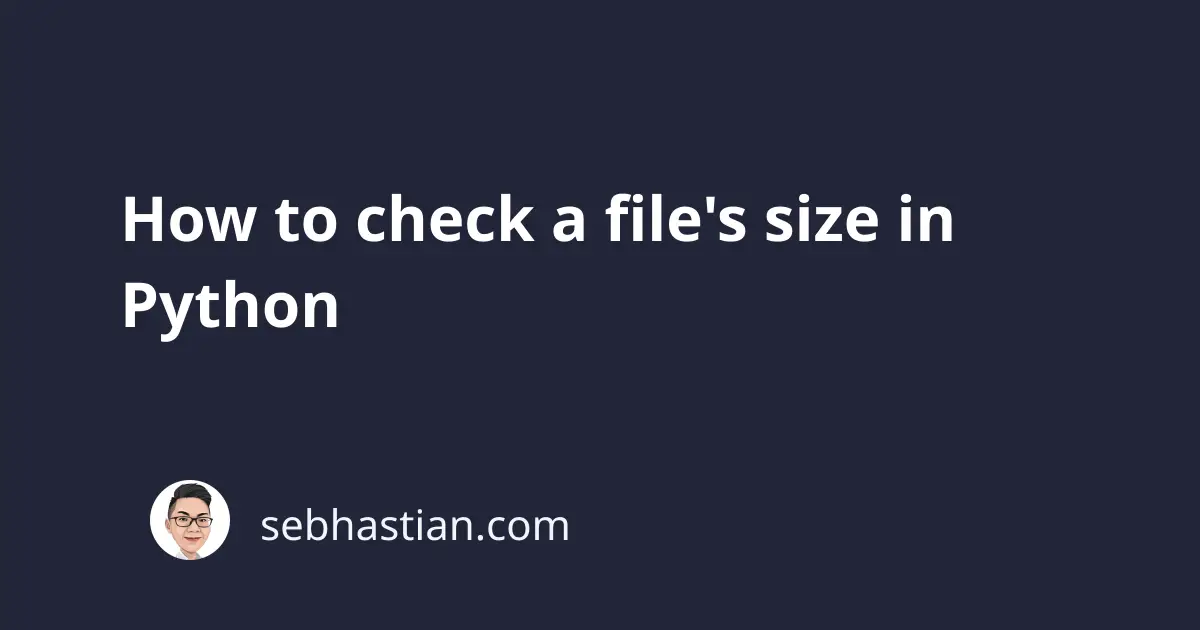
To check the size of a file using Python, you can use the built-in os
module.
In my experience, there are two easy methods you can use the os
module to help you get the file size:
- The
os.path.getsize('file_path')
function - The
os.stat('file_path').st_size
attribute
I’ve tested both methods, and this tutorial will show you how to use these them in practice.
1. Check file size with os.path.getsize(‘file_path’)
The getsize()
function from the path
module allows you to get the size of a file using its location path.
The function returns an integer number representing the file size in bytes.
For example, here’s how to get the size of an Excel file:
from os import path
file_size = path.getsize('data.xls')
print(f"data.xls file size: {str(file_size)} bytes")
Output:
data.xls file size: 33280 bytes
You can use both absolute and relative paths when specifying the file_path
argument.
If you want the output to be formatted in KB, MB, GB, or TB, then you can create a function that converts the file size as follows:
def convert_size(file_size):
for unit in ['bytes', 'KB', 'MB', 'GB', 'TB']:
if file_size < 1024.0:
return f"{file_size:3.1f} {unit}"
file_size /= 1024.0
In this function, you create a list of size units and iterate over that list with a for
loop.
In that loop, you need to check if the file_size
is less than 1024
which is equal to 1 unit of the next unit.
For example, 1024
bytes equals 1 KB
, so when the file size reaches that, you should divide the file size by 1024
and perform the next iteration.
This way, the function returns the next higher unit in the hierarchy only when the size is at least 1 unit or greater.
This way, you get the most relevant unit output:
from os import path
file_size = path.getsize('data.xls')
converted_size = convert_size(file_size)
print(f"data.xls file size: {converted_size}")
Because the file size is already returned as a string object, you don’t need to call the str()
function again.
Output:
data.xls file size: 32.5 KB
2. Get file size with os.stat(‘file_path’).st_size
As an alternative to the path.getsize()
function, the st_size
attribute from the stat()
function can also be used to get check the size of a file.
You need to specify the file path string as an argument to the stat()
function as shown below:
from os import stat
file_size = stat('data.xls').st_size
print(f"data.xls file size: {str(file_size)} bytes")
Output:
data.xls file size: 33280 bytes
The st_size
attribute returns the size of a file in bytes, just like the getsize()
function.
You can also use the same convert_size()
function to get the file size in higher units.
I hope this tutorial helps. Until next time! 👋