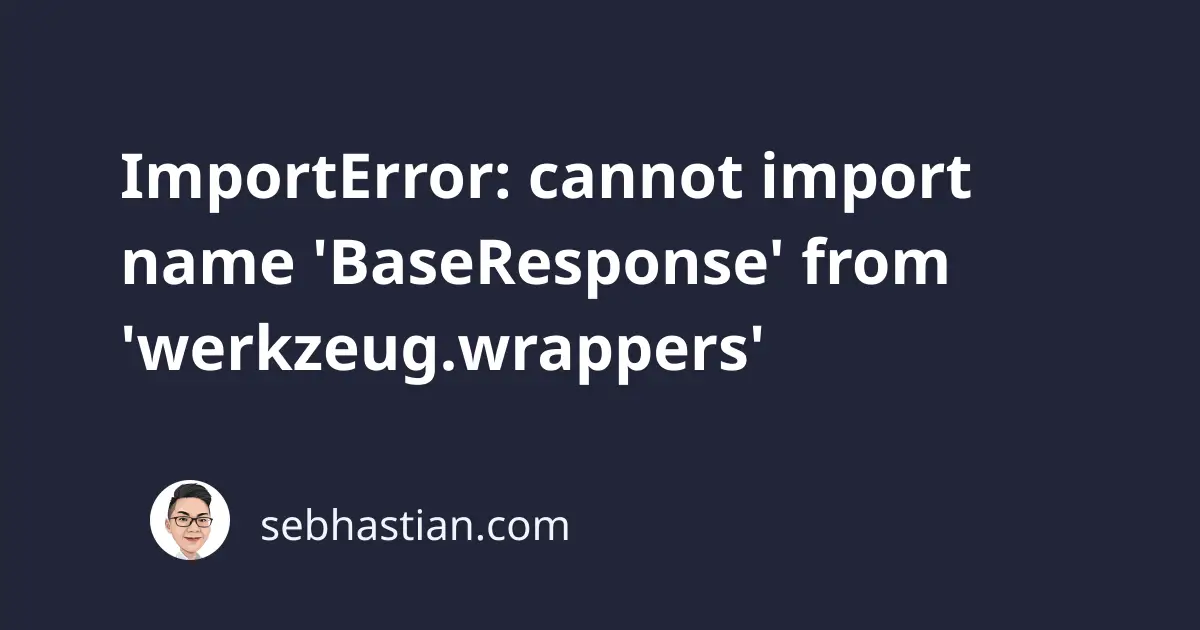
The werkzeug
package is a WSGI library used for developing web frameworks in Python.
Sometimes, you may encounter an error message that says:
ImportError: cannot import name 'BaseResponse' from 'werkzeug.wrappers'
The error above is caused by breaking changes introduced in werkzeug
version 2.1.0.
This tutorial will show you why this error occurs and the steps you can take to fix this error:
Why ImportError: cannot import name ‘BaseResponse’ from ‘werkzeug.wrappers’ occurs
This ImportError occurs because werkzeug
version 2.1.0 refactored and moved BaseRequest
and BaseResponse
modules to Request
and Response
respectively.
When you import one of these modules into your code, you’ll get the error below:
ImportError: cannot import name 'BaseRequest' from 'werkzeug.wrappers'
ImportError: cannot import name 'BaseResponse' from 'werkzeug.wrappers'
Let’s see how to fix this error next.
Upgrade packages that use werkzeug to the latest versions
If you use flask
or flask-lambda
package, then this error was resolved in the latest versions of these packages.
You can upgrade these packages using pip
:
pip install --upgrade flask-lambda
# For pip3:
pip3 install --upgrade flask-lambda
# If pip is not in PATH:
python -m pip install --upgrade flask-lambda
# python3:
python3 -m pip install --upgrade flask-lambda
But if the package you used has not released a new version to address this issue, then you need to downgrade your werkzeug
version.
Downgrade werkzeug to 2.0.3
Run one of the following commands to downgrade werkzeug
to version 2.0.3:
pip install werkzeug==2.0.3
# For pip3:
pip3 install werkzeug==2.0.3
# If pip is not in PATH:
python -m pip install werkzeug==2.0.3
# python3:
python3 -m pip install werkzeug==2.0.3
If you have a requirements.txt
file, then you can specify the werkzeug
version as follows:
werkzeug==2.0.3
Once you downgraded the package, the import to BaseResponse
and BaseRequest
should work again.
Update the import statement to use Request or Response
If you’re building an application that uses werkzeug
directly, you can update your import
statement as follows:
# from:
from werkzeug.wrappers import BaseResponse, BaseRequest
# to:
from werkzeug.wrappers import Response, Request
To make the code support both werkzeug
version 2.0.x and 2.1.x, you can wrap the import in a try-except
block.
When importing Request
, add an alias as BaseRequest
as follows:
try: # werkzeug <= 2.0.3
from werkzeug.wrappers import BaseRequest
except: # werkzeug >= 2.1.x
from werkzeug.wrappers import Request as BaseRequest
This way, the rest of your code can work without needing to replace BaseRequest
with Request
.
If you see another error after upgrading werkzeug
, then the best way to fix the error is to downgrade it.
Conclusion
The werkzeug
package has refactored and moved BaseRequest
and BaseResponse
modules to Request
and Response
respectively.
You will get an ImportError
when you import either one of the modules.
To fix this error, you can upgrade the packages that depend on werkzeug
to the latest version, or downgrade werkzeug
to version 2.0.x.
If you’re building an application with werkzeug
, then you can adjust the import
statement so that it supports all versions of werkzeug
.
I hope this tutorial was helpful. See you around! 🍻