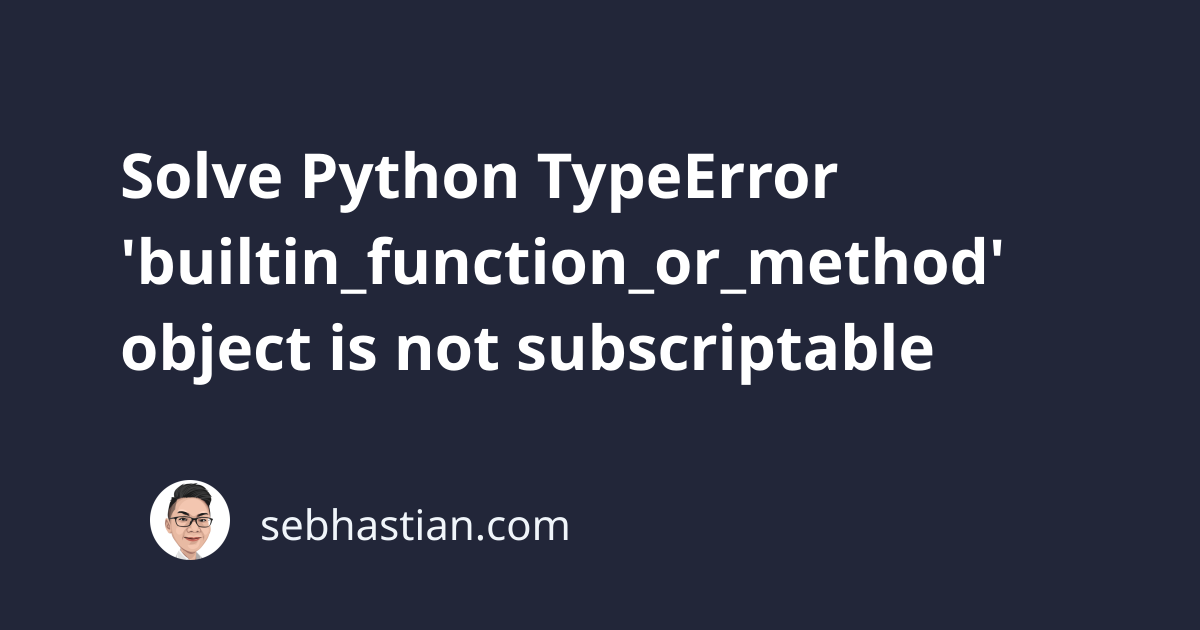
The Python output “TypeError: ‘builtin_function_or_method’ object is not subscriptable” happens when you are using square brackets []
when calling a function.
To solve this error, you need to inspect your code and change the square brackets to round brackets ()
in function calls.
Let’s see an example, suppose you have a Python script that prints something like this:
print["Hello World"]
The code above will produce the error because the print
function is called using square brackets.
To fix the error, you need to change those square brackets with round brackets:
print("Hello World")
The same also works when you have multiple arguments to pass to a function.
For example, suppose you are calling the min
function as follows:
result = min[7, 9, 4, 20]
print(result)
Because you use the square brackets when calling the min
function, the error occurs:
In Python, square brackets are used for several purposes:
- To create a new list variable
- To access the elements of string, list tuple, objects, and so on
When used for accessing elements, the square brackets become known as the subscript operator.
You can access individual letters of a string as shown below:
str = "Nathan"
# use square brackets to access string element(s)
print(str[0]) # N
print(str[1]) # a
print(str[2]) # t
print(str[3]) # h
print(str[4]) # a
print(str[5]) # n
When Python says the built-in function is not subscriptable, it is because those functions do not have anything that you can access like the string above.
Only objects that implement the __getitem__()
method are subscriptable in Python.
By default, tuples, objects, lists, and strings are the built-in objects that implement the __getitem__()
method:
# 👇 Python subscriptable objects:
# string
print("hello"[0]) # h
# tuple
print((1, 2, 3, 4)[0]) # 1
# list
print([1, 2, 3, 4][3]) # 4
# dict
print({"a": 1, "b": 2, "c": 3}["c"]) # 3
Therefore, only these objects can be accessed using the square brackets notation.
Now you’ve learned how to solve Python “TypeError: ‘builtin_function_or_method’ object is not subscriptable” issue. Nice work!