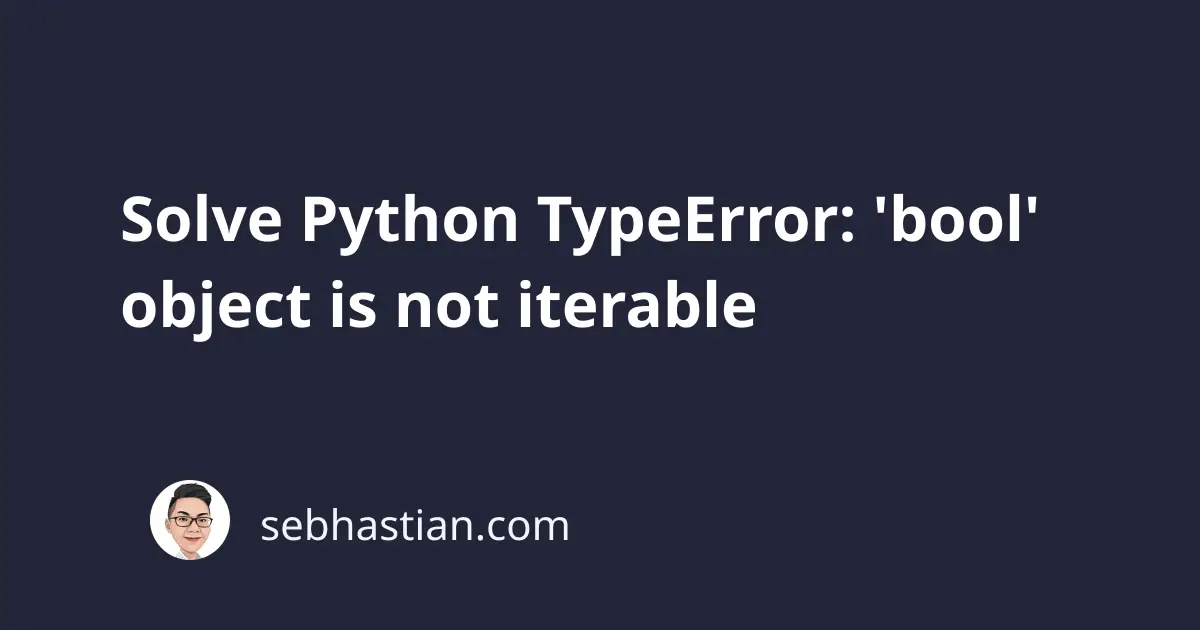
Python TypeError: 'bool' object is not iterable
message means a Boolean object (True
or False
) is being used in a context where an iterable object (like a list or tuple) is expected.
To solve this error, you need to correct the line where you iterate over a bool
object.
An iterable object is an object that can be looped over, such as a list, tuple, or string.
In contrast, a Boolean object is a single value and cannot be looped over. Consider the code below:
x = True
for value in x: # ❗️
print(value)
The for
loop in the code above tries to loop over the x
variable, which is a Boolean.
This causes the following error:
Traceback (most recent call last):
File ...
for value in x:
TypeError: 'bool' object is not iterable
To avoid the error above, you need to pass an iterable object such as a list, a string, or a tuple to the for
statement:
x = ["apple", "orange"]
for value in x:
print(value)
# apple
# orange
One common cause of this error is when you reassign your variable value after it has been created.
In the following example, the x
variable is first initialized as a list, but got reassigned as Boolean down the line:
# x initialized as a list
x = [1, 2, 3]
# x reassigned as a boolean
x = True
When you use the x
variable after the x = True
line, then you will get the 'bool' object is not iterable
error.
Another common scenario where this error occurs is when you pass a Boolean object to a function that requires an iterable.
For example, the list()
, tuple()
, set()
, and dict()
functions all require an iterable to be passed:
x = True
# ❌ TypeError: 'bool' object is not iterable
list(x)
tuple(x)
set(x)
dict(x)
You can also add an if
statement to check if your variable is a boolean:
x = True
if type(x) == bool:
print("x is of type Boolean.") # ✅
else:
print("x is not of type Boolean.")
Or you can also use the isinstance()
function to check if x
is an instance of the bool class:
x = True
if isinstance(x, bool):
print("x is of type Boolean.") # ✅
else:
print("x is not of type Boolean.")
When you see a variable identified as a Boolean, you need to inspect your code and make sure that the variable is not re-assigned as Boolean after it has been declared.
You will avoid this error as long as you don’t pass a Boolean object where an iterable is expected.