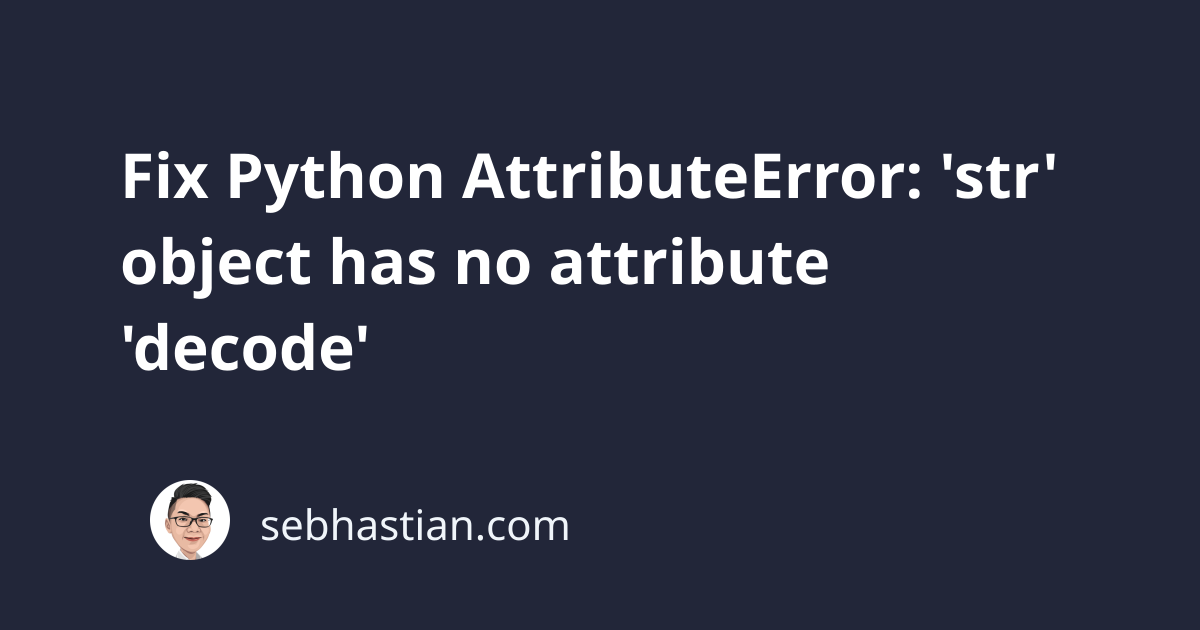
Python shows “AttributeError: ‘str’ object has no attribute ‘decode’” error when you try to use the decode()
method on a string object.
To fix this error, you need to remove the call to decode()
method from your string.
The decode()
method is used to convert encoded strings, such as those in bytes or bytearrays, back into their original Unicode representation.
In Python version 3, strings are already Unicode by default, so there is no need to decode them. Therefore the decode()
method is removed from string objects.
The following code example would trigger this error:
string = "Hello, world!"
# ❌ AttributeError: 'str' object has no attribute 'decode'
decoded_string = string.decode()
print(decoded_string)
The error message would be:
To fix this error, you need to make sure that you are only attempting to use the decode()
method on objects that are encoded, such as bytes or bytearrays.
When you have a string, you don’t need to decode the value anymore, so you can immediately print it:
string = "Hello, world!"
# Just print it
print(decoded_string)
Encoding converts a string to bytes while decoding converts bytes to a string.
You can use the encode()
method on a string object to convert it into an encoded form, then call decode()
on it. For example:
string = "Hello, world!"
# Encode the string into bytes
encoded_string = string.encode()
# Decode the bytes back into a string
decoded_string = encoded_string.decode()
print(decoded_string) # "Hello, world!"
If you are not sure whether you have a string or bytes object, you can use an if
statement to check on the type of the variable before calling either encode()
or decode()
.
See the example below:
def decode_string(string):
if isinstance(string, bytes):
# String is encoded in bytes, so decode it
return string.decode()
else:
# String is already Unicode, so return it as-is
return string
# Test the function with a string and a bytes object
print(decode_string("Hello, world!")) # "Hello, world!"
print(decode_string(b"SGVsbG8sIHdvcmxkIQ==")) # "Hello, world!"
First, you create a function named decode_string()
that will decode a bytes object.
The isinstance()
function is used to check the type of the string variable. If it is a bytes
object, then the decode()
method is called on it. Otherwise, the string is returned without any modification.
The test results show that a string isn’t modified, while a base64-encoded bytes object is decoded into a string.
Feel free to use the above function in your Python project. 👍
Conclusion
To conclude, the Python error “AttributeError: ‘str’ object has no attribute ‘decode’” occurs when you try to use the decode()
method on a string object.
To fix this error, make sure you are only using the decode()
method on encoded objects.
When you are not sure about your variable type, use the isinstance()
function to validate the type and continue to process your object accordingly.