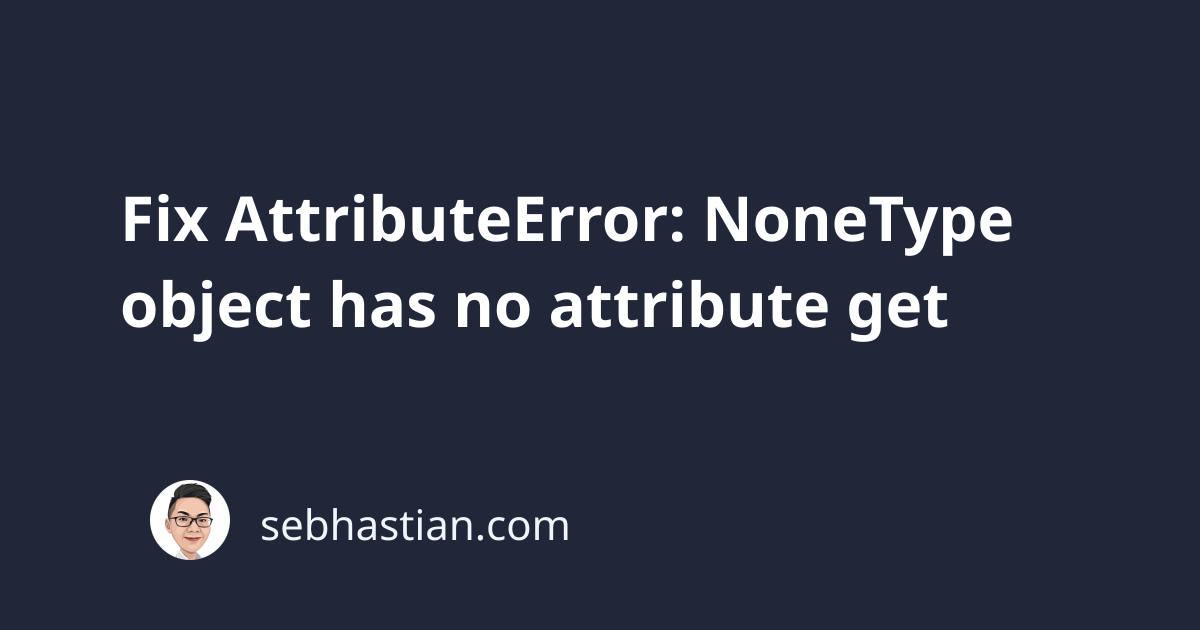
Python AttributeError: NoneType object has no attribute get
means that you are trying to call the get()
method on a None
object.
This error usually occurs when you mistake a None
object for something else.
Why this error occurs
To generate this error you can call the get()
method from a None
object as shown below:
obj = None
result = obj.get("url") # ❌
Because the obj
variable contains a None
value, Python responds with the following error message:
Traceback (most recent call last):
File ...
result = obj.get("url")
AttributeError: 'NoneType' object has no attribute 'get'
Let’s see how to fix this error next
How to fix ‘NoneType’ object has no attribute ‘get’
get()
is a method of the dictionary object that’s used to get the value of a key in a dictionary object.
Here’s an example to use the method:
my_dict = {"name": "Nathan"}
result = my_dict.get("name")
print(result) # Nathan
The error means you are calling the get()
method from a NoneType
object instead of a dictionary object.
One way to avoid this error is to check the type of your variable with the isinstance()
function.
Add an if-else
statement so that you only call the get()
method when the variable is a dictionary as follows:
obj = {"name": "Nathan"}
if isinstance(obj, dict):
print("obj is a dictionary.")
obj.get("name") # ✅
else:
print("obj is not a dictionary.")
If you know that you only get a dictionary object, you can also use the is not None
expression to avoid calling get
on a NoneType
object.
This is especially useful when you are looping over a list of dictionary objects, where you may get one or two elements that have None
value:
result = [
{"name": "Nathan"},
None,
{"name": "Lysa"},
None,
]
for element in result:
if element is not None:
print(element.get("name"))
This way, the None
values in the list won’t cause an error as they will be skipped.
Now you’ve learned how to fix Python AttributeError: NoneType object has no attribute get
. Very nice! 👍