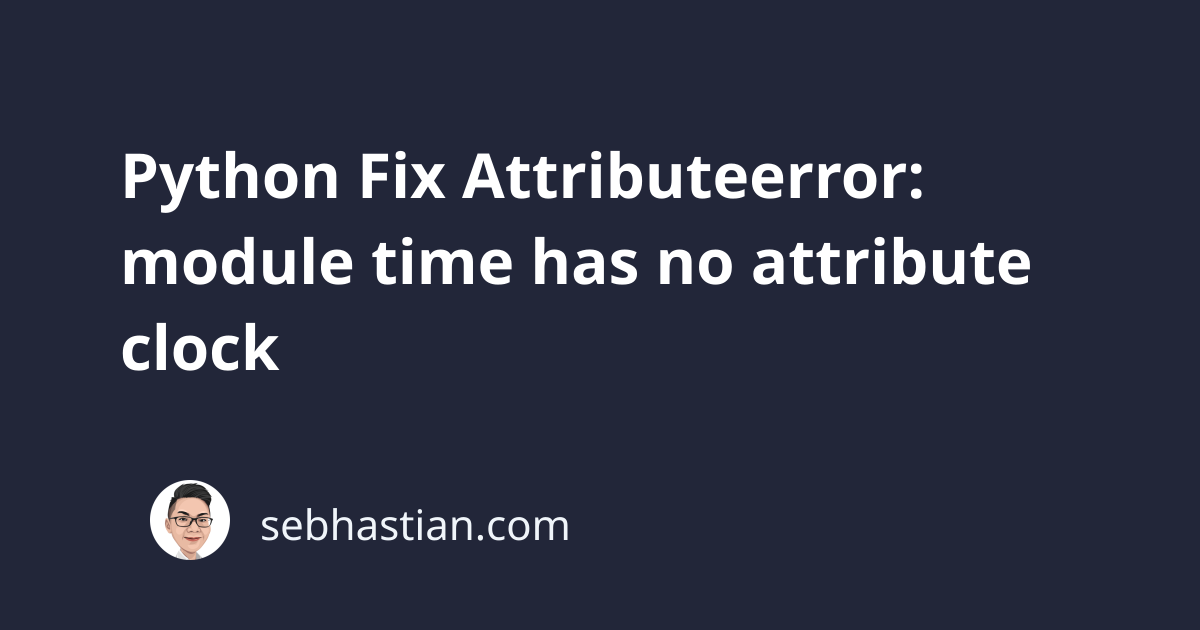
The Python error Attributeerror: module time has no attribute clock
happens when you try to use the time.clock()
method in Python version 3.8 or above.
This error happens because the clock()
method was removed from the time
module in Python version 3.8.
Here’s an example of this error:
Traceback (most recent call last):
File ...
start_time = time.clock()
AttributeError: module 'time' has no attribute 'clock'
To fix this error, you need to replace the call to the clock()
method with either perf_counter()
or process_time()
method. Let’s learn about these two methods next.
The perf_counter()
method returns a float value of a performance counter in fractional seconds.
This function includes time elapsed during sleep or waiting for other processes. Here’s an example of using the function:
import time
start_time = time.perf_counter()
time.sleep(1)
end_time = time.perf_counter()
print(end_time - start_time) # 1.005034167028498
The process_time()
method is similar to the perf_counter()
method, but it excludes the time spent waiting for other processes (sleep, I/O operations, network latency)
Here’s an example code that uses the process_time()
method:
import time
start_time = time.process_time()
time.sleep(1)
end_time = time.process_time()
print(end_time - start_time) # 0
You may not get 0
when running the code above, but the result should still be less than the perf_counter()
method.
In my case, perf_counter()
returns a value of 1.005065375007689
, larger than the value returned by process_time()
which is 4.300000000000137e-05
.
If you didn’t use the clock()
method but receive this error, then you probably have imported modules that use this method.
For example, it’s well known that the PyQtGraph and SQLAlchemy libraries cause this error in their older versions.
You need to upgrade these libraries to the latest version using pip
as follows:
pip install sqlalchemy pyqtgraph --upgrade
# or if you use pip3:
pip3 install sqlalchemy pyqtgraph --upgrade
Once you upgraded the libraries to the latest versions, you should be able to run your code without seeing this error.
If your module doesn’t release a newer version to address this issue, then you can downgrade your Python version to 3.7 to use the clock()
method.
Conclusion
The time.clock()
method was removed in Python version 3.8 because the method was platform-dependent.
On Windows, it returns wall-clock seconds elapsed since the first call to this function. On Unix, it returns the current processor time as a floating point number expressed in seconds1.
The perf_counter()
and process_time()
methods were introduced to address this inconsistent behavior by the clock()
method.
perf_counter
measures the time required for a process to complete, while process_time
measures the time spent by the CPU for the process. It doesn’t count the time when the CPU is running anything else.
perf_counter
is system-wide, while process_time
is process-wide.
Now you’ve learned how to solve the Python Attributeerror: module time has no attribute clock
. Good work! 👍
Until next time.
Python time.clock() documentation https://docs.python.org/3.5/library/time.html?highlight=clock#time.clock ↩︎