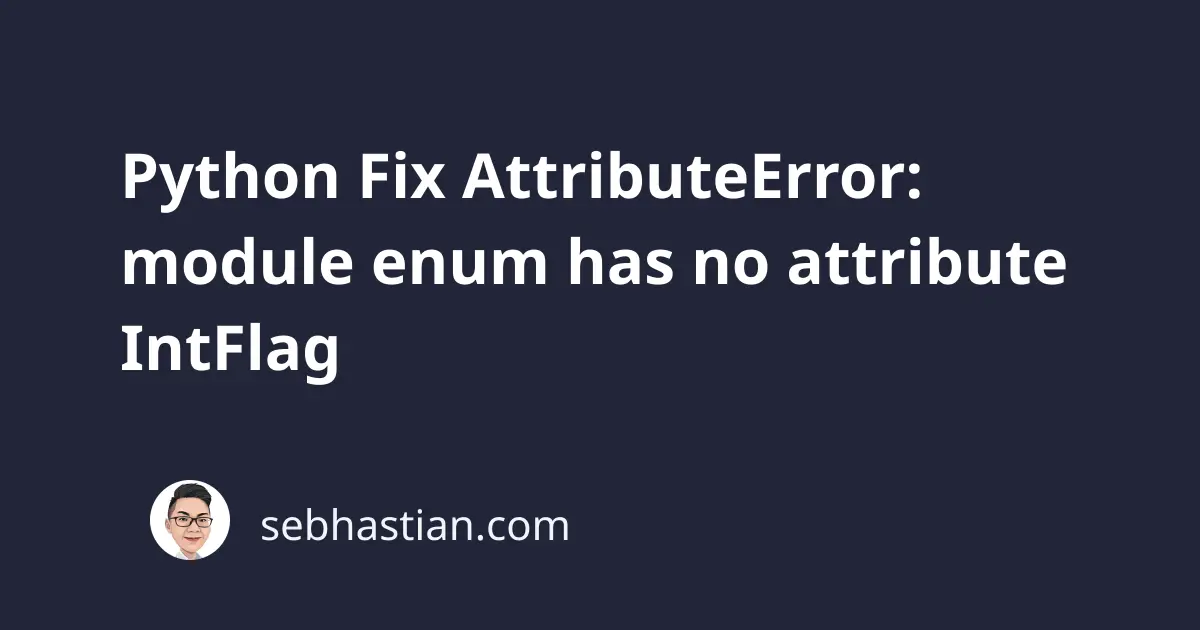
When working with the enum
module in Python, sometimes you get an error saying AttributeError: module enum has no attribute IntFlag
.
This error happens because Python can’t find the IntFlag
class from the enum
module.
This error is often encountered when trying to define a set of flags represented by integers as shown below:
import enum
class Permissions(enum.IntFlag):
READ = 1
WRITE = 2
EXECUTE = 4
The enum
module was added in Python version 3.4, but the IntFlag
class was added later in Python version 3.6.
This means when you use Python below 3.6, the IntFlag
class won’t be available. You can use the IntEnum
class instead:
import enum
class Permissions(enum.IntEnum):
READ = 1
WRITE = 2
EXECUTE = 4
If you already use Python version 3.6 and above, then you may have the enum34
module installed.
If you have enum34
module installed, Python will use that module instead of the built-in enum
module. This module was created as a backport for Python version 2.7 to 3.3.
Run the following command to remove enum34
:
pip uninstall -y enum34
# or if you have pip3:
pip3 uninstall -y enum34
Once the enum34
module is uninstalled, you should be able to use IntFlag
class in your code.
If you still see the error, then another possibility is that you have a Python file named enum.py
in your current project.
You can check where Python gets the enum
module with the following code:
import enum
print(enum.__file__)
When you have a file named enum.py
in your project, the code above will show a similar output like this:
/Users/nsebhastian/Desktop/DEV/python/enum.py
The right path to the enum.py
should be from your Python installation directory as follows:
/usr/lib/python3.10/enum.py
You need to rename or delete the project file named enum.py
so that Python can get the built-in module.
If you still have this error, then try to unset your PYTHONPATH
variable.
Run the following command from the terminal:
unset PYTHONPATH
The PYTHONPATH
variable tells the Python interpreter about where to find various libraries installed on your computer.
Resetting this path should cause Python to seek the built-in module in the default location.
Conclusion
The Python IntFlag
class was included in the enum
module in Python version 3.6, so you can’t use this class when you have a Python version lower than that.
Alternatively, you can also have this error because you installed the enum34
module.
The enum34
module doesn’t have the IntFlag
class because it was a backport of the enum
module released in Python version 3.4. This version of enum
module doesn’t have the IntFlag
class yet.
Finally, make sure you don’t have a file named enum.py
in your Python project because it would override the built-in enum
module. You need to rename or remove the file if you have one.
And now you’ve learned how to solve the AttributeError: module enum has no attribute IntFlag
in Python. Cheers! 🍻