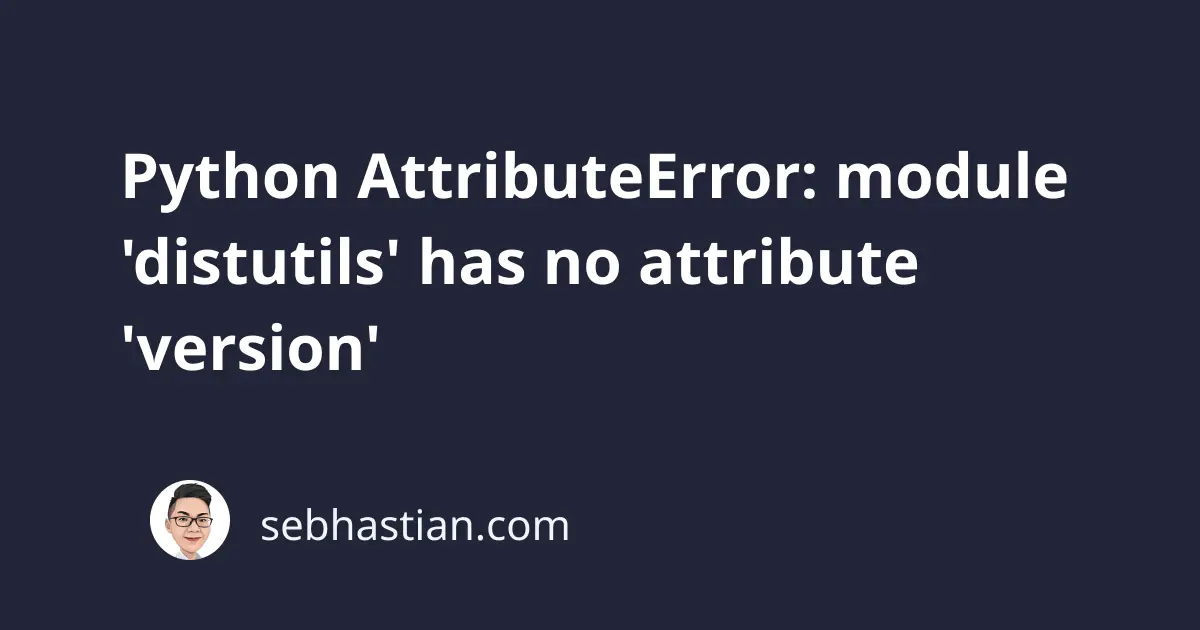
When installing or running Python code that uses PyTorch, you might see an error that says:
AttributeError: module 'distutils' has no attribute 'version'
This article will help you to understand why this error occurs and how to resolve it.
Why AttributeError: module ‘distutils’ has no attribute ‘version’ occurs
This error occurs when you try to access the version
attribute from the distutils
module as follows:
from setuptools import distutils
print(distutils.version) # ❌
This error happens because of the changes in setuptools
version 59.6.0, which somehow break the call to version
attribute.
What’s more, the version
attribute seems to have been removed from distutils
in the latest version. You can confirm this by running the dir()
function:
from setuptools import distutils
print(distutils.__version__, dir(distutils))
The output will be as follows:
3.10.9 ['_', '__builtins__', '__cached__', '__doc__', '__file__',
'__loader__', '__name__', '__package__', '__path__', '__spec__',
'__version__', '_log', 'archive_util', 'cmd', 'command', 'config', 'core',
'debug', 'dep_util', 'dir_util', 'dist', 'errors', 'extension',
'fancy_getopt', 'file_util', 'filelist', 'importlib', 'log', 'spawn',
'sys', 'util']
As you can see, there’s only the __version__
attribute shown in the output.
This error is known to happen when importing or installing the PyTorch package, which was fixed in PyTorch version 1.11.0.
How to resolve AttributeError: module ‘distutils’ has no attribute ‘version’
If you’re using PyTorch, you can resolve this error by upgrading the torch
package.
Run one of the following commands to upgrade the torch
package:
# If you use pip:
pip install --upgrade torch
# For pip3:
pip3 install --upgrade torch
# If you use conda:
conda update pytorch
Or if you want to test PyTorch version 1.11.0:
# If you use pip:
pip install torch==1.11.0
# For pip3:
pip3 install torch==1.11.0
# If you use conda:
conda install pytorch=1.11.0
Once you upgraded the PyTorch version, the error should disappear.
If you’re using any other Python package that hasn’t addressed this error, then you can pin the setuptools
version to 59.5.0
so that distutils.version
still works.
Use one of the following commands to pin the setuptools
package version:
# If you use pip:
pip install setuptools==59.5.0
# For pip3:
pip3 install setuptools==59.5.0
# If you use conda:
conda install setuptools=59.5.0
If conda responds with PackagesNotFoundError
, then you need to install pip
in the conda environment and use it to install setuptools
:
# In conda environment, install pip:
conda install pip
# Then install setuptools:
pip install setuptools==59.5.0
The distutils.version
attribute should now be accessible.
Alternatively, you can also adjust the import
statement to import distutils.version.LooseVersion
if you have access to the source code:
# from:
from setuptools import distutils
# to:
from distutils.version import LooseVersion
While importing distutils
from setuptools
will cause an error, importing the LooseVersion
class works just fine.
Conclusion
The error AttributeError: module 'distutils' has no attribute 'version'
occurs when you try to access the version
attribute from the distutils
module.
The latest version of distutils
module no longer has the version
class as seen in the dir()
function output, so you need to either import LooseVersion
class or downgrade the setuptools
package to version 59.5.0
.
If you see this error when running PyTorch, upgrade the PyTorch package to version 1.11.0 or newer.
Great work on resolving this error. See you in other articles! 🙏