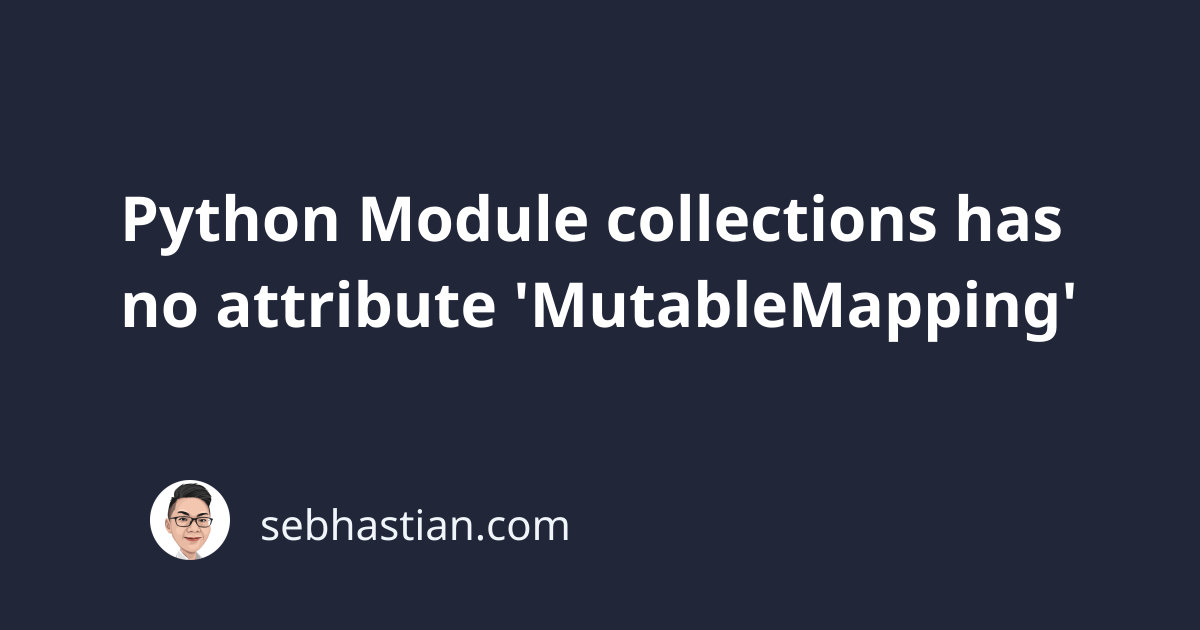
Python AttributeError: module 'collections' has no attribute 'MutableMapping'
occurs because the MutableMapping
class has been moved from the collections
module to collections.abc
module in Python version 3.10.
This tutorial will show you the best solutions to fix this error.
How to fix AttributeError: module ‘collections’ has no attribute ‘MutableMapping’
You can select one of the solutions below that fits your situation.
Solution #1: Upgrade Python packages to the latest versions
If you see this error when running pip
commands, then you can try to upgrade the built-in Python packages and see if it fixes the error.
Run one of the following commands from the terminal:
pip install --upgrade pip wheel setuptools requests
# For pip3:
pip3 install --upgrade pip wheel setuptools requests
# If pip is not in PATH:
python -m pip install --upgrade pip wheel setuptools requests
# python3:
python3 -m pip install --upgrade pip wheel setuptools requests
This is because an outdated version of one of these packages will trigger the error.
The latest versions of setuptools
and requests
have addressed this error and adjusted the import
statement in their source code.
Solution #2: Downgrade Python to version 3.9.x
Another way to fix this error is to downgrade your Python version to 3.9.
You can download recent Python versions from Python official website.
Download the latest Python version 3.9.x as shown below:
But keep in mind that Python version 3.9.16 requires you to build from source as there’s no installer for that version.
If you prefer an installable package, you need to download Python version 3.9.13 which is the latest regular maintenance release for Python 3.9.
Once you installed Python v3.9, the MutableMapping
class error should be resolved.
Solution #3: Change the import statement for MutableMapping class
Up until Python version 3.9, you can import the MutableMapping
class as follows:
from collections import MutableMapping
But in Python 3.10, the import
statement needs to be changed to work:
from collections.abc import MutableMapping
If you have access to the source code, you can find and replace all imports for the MutableMapping
class to the collections.abc
module.
If you want the import
statement to work for all Python versions, then use a dynamic import
statement with a try-except
block as follows:
try:
# Import for >=3.10
from collections.abc import MutableMapping
except ImportError:
# Import for <=3.9
from collections import MutableMapping
# Your code...
The try
statement will try to import from the collections.abc
module.
When the import causes an error, the except
block will try to import from the collections
module instead.
Conclusion
This tutorial shows you that the AttributeError: module 'collections' has no attribute 'MutableMapping'
occurred because the MutableMapping
class has been removed from the collections
module in Python version 3.10.
Some built-in packages like pip
, wheel
, setuptools
, and requests
that use the MutableMapping
class need to be upgraded so that the error can be resolved.
You can also downgrade your Python version or replace the import
statement in your code to resolve this error.
I hope this tutorial was helpful. See you in other articles! 👍