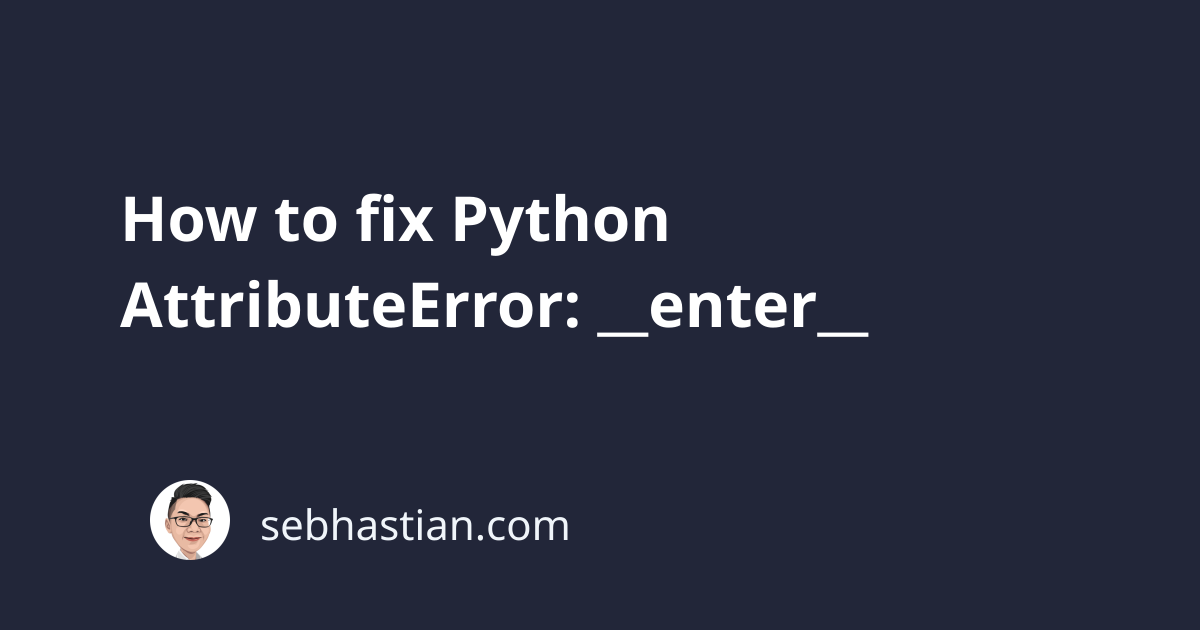
One error that you might encounter in Python is:
AttributeError: __enter__
This error usually occurs when you use the with
statement to call a context manager.
There are 4 possible causes for this error:
- The
__enter__
attribute is not defined - Using a string in the
with
statement - You replaced the
open()
function - You forget to instantiate the class in the
with
statement
This tutorial explains why this error occurs and how to fix it.
1. The __enter__
attribute is not defined
A context manager is a way to manage resources in Python. It allows you to automatically create and release resources following the execution of your program.
A context manager is called using the with
statement. One of the most familiar examples of using a context manager is when you use Python to open a file and write a string in it:
with open("file.txt", "w") as f_obj:
f_obj.write("first line")
Using the with
statement, a context manager allows you to access the file stream inside the statement’s body.
When you’re done with the file, the with
statement automatically releases the file stream object.
Without the with
statement, you need to close the file stream manually as follows:
f_obj = open("file.txt", "w")
f_obj.write("first line")
# Close the file stream
f_obj.close()
You might be wondering, what’s this got to do with the error?
When you call a context manager using the with
statement, the __enter__
attribute is executed when the context manager creates the resources.
Let’s see an example by defining a class named Car
as follows:
class Car:
def __enter__(self):
print('__enter__: Creating Resources...')
return self
def __exit__(self,exc_type, exc_val, exc_tb):
print('__exit__: Releasing resources...')
The Car
class has both __enter__
and __exit__
attributes. The former is called when you start the context manager, and the latter is called when the context manager is cleared.
Let’s create an object of the Car
class and access the object as follows:
with Car() as obj:
print(obj)
You’ll get the following output:
__enter__: Creating Resources...
<__main__.Car object at 0x10503fac0>
__exit__: Releasing resources...
As you can see, the __enter__
attribute is called when the with
statement is executed.
The error occurs when you use the with
statement with an object that doesn’t have the __enter__
attribute:
class Car:
def __init__(self):
print("Initializing car...")
with Car() as obj:
print(obj)
Output:
Initializing car...
Traceback (most recent call last):
File "main.py", line 6, in <module>
with my_car as obj:
AttributeError: __enter__
To resolve this error, make sure that you have an __enter__
attribute defined in your class.
You also need to add an __exit__
attribute in your class, or you’ll get the AttributeError: __exit__
message.
class Car:
def __init__(self):
print("Initializing car...")
def __enter__(self):
print('__enter__: Creating Resources...')
return self
def __exit__(self,exc_type, exc_val, exc_tb):
print('__exit__: Releasing resources...')
Once you define the attributes, the error should disappear.
2. Using a string in the with
statement
You also get this error when you tried to access a file by directly specifying the file name in the with
statement:
with 'file.txt' as f_obj:
lines=f_obj.readlines()
Output:
Traceback (most recent call last):
File "main.py", line 1, in <module>
with 'file.txt' as file_object:
AttributeError: __enter__
You can’t access a file directly, so use the open()
function instead:
with open("file.txt", "r") as f_obj:
Using the open()
function as shown above should fix the error.
3. You replaced the open()
function
You could also get this error when running the with open()
function:
with open("file.txt", "r") as f_obj:
AttributeError: __enter__
This error occurs when you reassign the open()
function to something else.
You might have created a function that uses the same name like def open()
in your code, or you assign something to it like open = ...
Python will replace the default open()
function with the one you have in your code, so you need to search your code for the cause.
4. You forget to instantiate the class in the with
statement
The with
statement must be used to manage a class instance, which is an object.
If you forget to instantiate the object and use the class instead, you’ll get the same error:
with Car as obj:
print(obj)
# with Car as obj:
# AttributeError: __enter__
The Car
in the above example is written without the parentheses, so the with
statement tries to access the class itself and not the class instance.
You need to create an instance of the class by adding parentheses, e.g. Car()
to fix this error.
Conclusion
This tutorial explains that the AttributeError: __enter__
occurs when the context manager can’t find and execute the __enter__
attribute.
You need to make sure that the __enter__
attribute is defined in your class, and that you’re using the right object in the with
statement.
I hope this tutorial is helpful. See you again in other tutorials! 👋