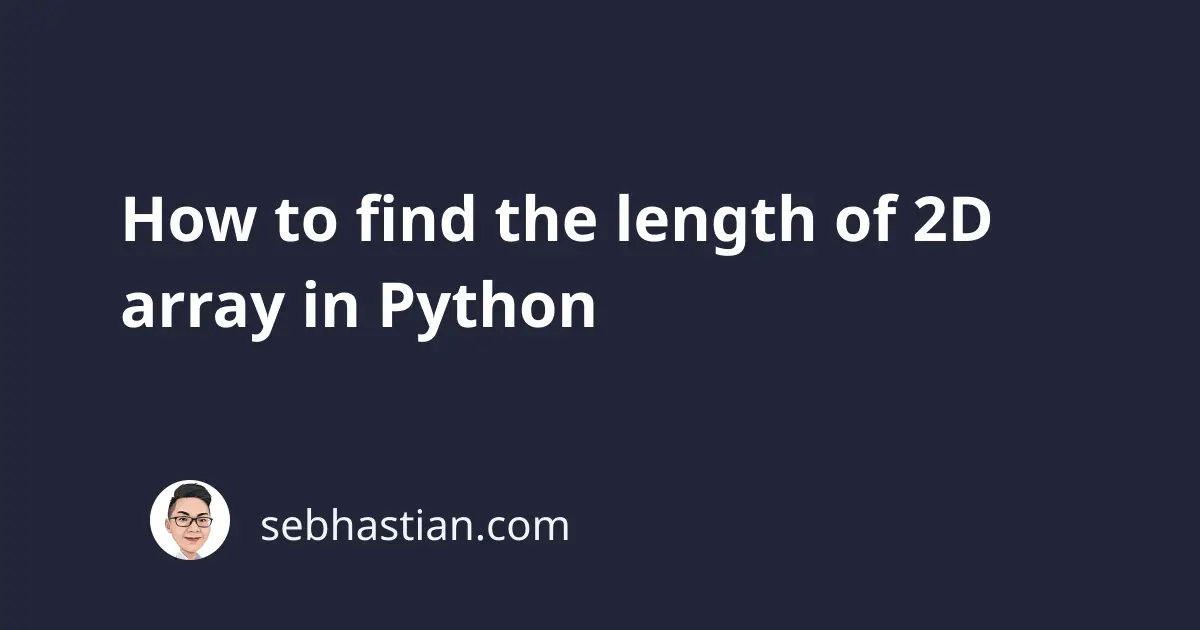
To find the length of a 2D array, you need to find the total number of items stored in that array with the len()
function.
The len()
function returns the length (number of items) of your array. You can use the len()
function to get the number of rows and columns that the 2D array has.
If the 2D array has the same number of columns for each row, you can multiply the number of rows * columns
to get the array length.
Let’s see an example. Suppose you have a 2D array as follows:
my_array = [[1, 2, 3], [7, 8, 9]]
# get the rows
rows = len(my_array)
# get the columns
cols = len(my_array[0])
print("Total rows:", rows)
print("Total columns:", cols)
print("2D array length:", rows * cols)
The output will be:
Total rows: 2
Total columns: 3
2D array length: 6
Note that this solution assumes that the 2D array has the same number of items for each row.
If you have an array with a different number of items, then you need to use a for
loop to manually get the length of each row.
Here’s how you do it:
my_array = [
[1, 2], [7, 8, 9], [10], [100, 101, 102], [200]
]
# get the rows
rows = len(my_array)
total_items = 0
# Sum the length of each row
for i in range(rows):
total_items += len(my_array[i])
print("Total rows:", rows)
print("2D array length:", total_items)
Output:
Total rows: 5
2D array length: 10
Inside the for
loop, you get the length of each row and add them to the total_items
variable.
This way, you can get the length of a 2D array that has a different number of items in each row.
You can also use the for
loop in one line with the help of the sum()
function:
my_array = [
[1, 2], [7, 8, 9], [10], [100, 101, 102], [200]
]
total_items = sum(len(col) for col in my_array)
print(total_items) # 10
The sum()
function will sum the values you set as its arguments. The above example pass the length of each column by using an inline for
loop.
Get the length of NumPy 2D array
If you use NumPy to create the 2D array, then you can use the shape
and size
attributes to get the array length information.
Here’s how it works:
import numpy as np
np_array = np.array([[1, 2, 3], [4, 5, 6]])
rows, cols = np_array.shape
size = np_array.size
print("Total rows:", rows)
print("Total columns:", cols)
print("2D array length:", size)
The shape
attribute returns a tuple that represents the number of rows and columns the 2D array has.
The size
attribute returns the total number of items the array has.
Note that if you have a 2D array with different numbers of columns, then the shape
and size
attributes won’t work.
You need to use the for
loop and sum()
function like a regular array:
import numpy as np
np_array = np.array([[1, 2], [4, 5, 6]], dtype=object)
total_items = sum(len(col) for col in np_array)
print("NumPy 2D array length:", total_items)
Output:
NumPy 2D array length: 5
You also need to specify the dtype
parameter when creating the array, or Python will show you a warning in the output.