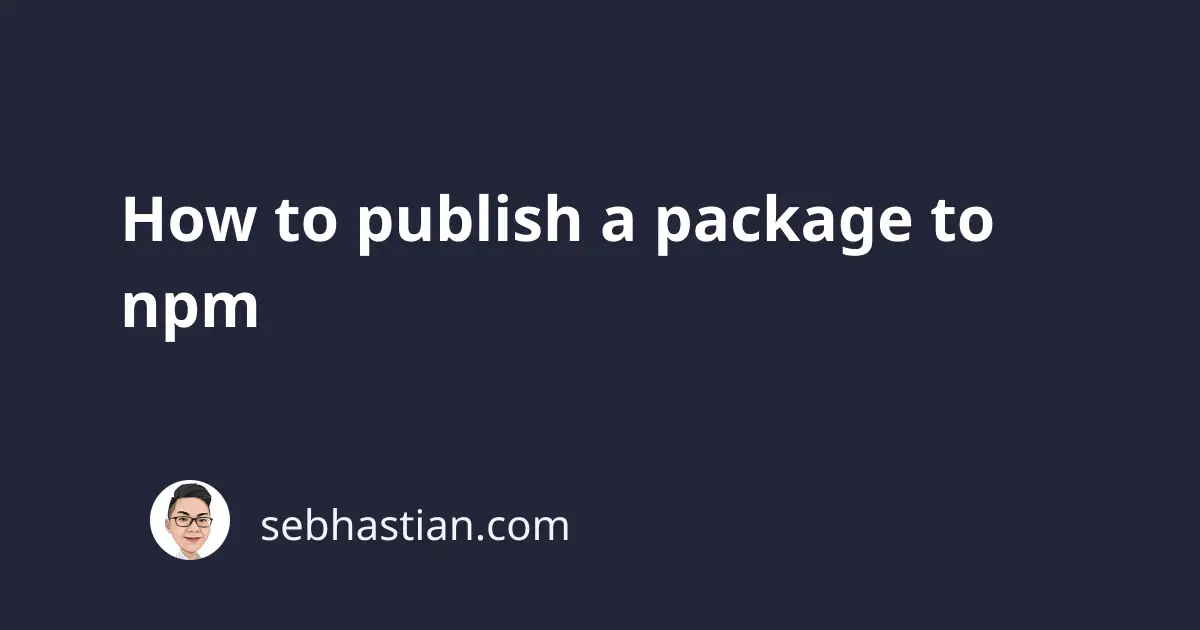
The npm is a public Node package manager where everyone can publish a package and share JavaScript code with others.
To publish a package to npm, you need to follow these steps:
- Create an npm account in npmjs.com
- Sign in to npm from your terminal
- Prepare your package for publishing
- Publish the package from the terminal
The first thing you need to do is sign up for a free account on npmjs.com.
npm has a paid pro account where you can publish private packages, but the free account will be enough to follow this tutorial.
Once you have an npm account, sign in to that account from the terminal by running the npm login
command.
The terminal will ask for your username, password, and email as shown below:
$ npm login
npm notice Log in on https://registry.npmjs.org/
Username: nsebhastian
Password:
Email: (this IS public)
Logged in as nsebhastian on https://registry.npmjs.org/.
At times, npm may also ask for an OTP send to your email. Check the inbox of the email that you use to register the account, and you should see an email containing the OTP.
Once you’re logged in to the npm registry, the next step is to prepare a package for publishing.
The only requirement for you to publish a package to npm is that it has a package.json
file available in the root directory.
For this tutorial, you can create a simple package JSON file as follows:
// package.json
{
"name": "dx-app",
"version": "1.0.0",
"description": "Learning how to publish your first package",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "Nathan Sebhastian",
"license": "ISC"
}
You may also want to add important fields like the homepage
and the repository
where you publish the source code.
Once your package is ready, you can publish it using the npm publish
command.
npm will log some information on the terminal as shown below:
$ npm publish
npm notice
npm notice 📦 [email protected]
npm notice === Tarball Contents ===
npm notice 165B README.md
npm notice 85B index.js
npm notice 286B package.json
npm notice === Tarball Details ===
npm notice name: dx-app
...
npm notice Publishing to https://registry.npmjs.org/
+ [email protected]
The above output means that the dx-app
package has been published successfully.
You can visit the package from npmjs.com website: https://www.npmjs.com/package/dx-app
You’ve now learned how to publish an npm package to npm registry. But publishing an empty npm package is not that useful for others, so let’s add some code to the package.
Create an index.js
file in your package with the following code:
function greetings() {
console.log("Hello World!");
}
module.exports = { greetings };
To publish an update to your npm package, you need to move the version number up in the package.json
file:
{
"name": "dx-app",
"version": "1.0.1",
"description": "..."
}
Now you can publish the latest package version to the npm registry with the npm publish
command.
Alternatively, you can also unpublish your package first using npm unpublish
.
Run the following command from the root directory of your package:
# 👇 unpublish the whole package
npm unpublish -f
# 👇 unpublish a single version
npm unpublish <package-name>@<version>
You can unpublish a package from npm registry within the first 72 hours after the initial publish.
Beyond the first 72 hours, you can still unpublish a package if it follows the criteria:
- Has a single owner/maintainer
- Has no public dependents listed in npmjs.com
- Had less than 300 downloads over the last week
Also, keep in mind that you can’t republish the same package until 24 hours have passed after unpublishing with the -f
option.
It’s better to publish a new version and ignore the previous version altogether. Only unpublish the previous version if you have leaked some sensitive information.
Next, you can try to use the package in your other project by running npm install
:
npm install dx-app
Once the package is installed, import the code into your application:
// 👇 write the code in your index.js file
const dx = require("dx-app");
dx.greetings();
Then run the code using node
and you will see the following output:
$ node index.js
Hello World!
This means the package has been installed in your node_modules
folder, and the code is accessible for everyone from npm.
Congratulations on publishing your first npm package! 👍
What to do if your npm package name is taken
The npm registry requires your package name to be unique.
This means if someone has already published a package with the same name as yours, you won’t be able to publish your package.
For example, there’s an existing package named vn-app
in the npm registry.
When I try to publish the same package, the output is as follows:
$ npm publish
npm notice Publishing to https://registry.npmjs.org/
npm ERR! code E403
npm ERR! 403 403 Forbidden - PUT https://registry.npmjs.org/vn-app -
You do not have permission to publish "vn-app".
Are you logged in as the correct user?
npm ERR! 403 In most cases, you or one of your dependencies are requesting
npm ERR! 403 a package version that is forbidden by your security policy, or
npm ERR! 403 on a server you do not have access to.
npm ERR! A complete log of this run can be found in:
npm ERR! /Users/nsebhastian/.npm/_logs/2022-07-01T04_52_11_548Z-debug-0.log
To handle this issue, you need to change your package name in the package.json
file to one that doesn’t exist in the npm registry already.
Or you can publish the package under a scope.
An npm scope is a way of grouping packages together. You can put your package under your user/organization name.
To put your package under a scope, write @username/package-name
as the name
in your package.json
file.
Use the example below as reference:
{
"name": "@nsebhastian/vn-app",
"version": "1.0.0",
"description": "..."
}
Finally, publish the package with --access public
option:
npm publish --access public
You should see the package published under your username like this:
https://www.npmjs.com/package/@nsebhastian/vn-app
And that’s how you publish an npm package under a scope. 😉