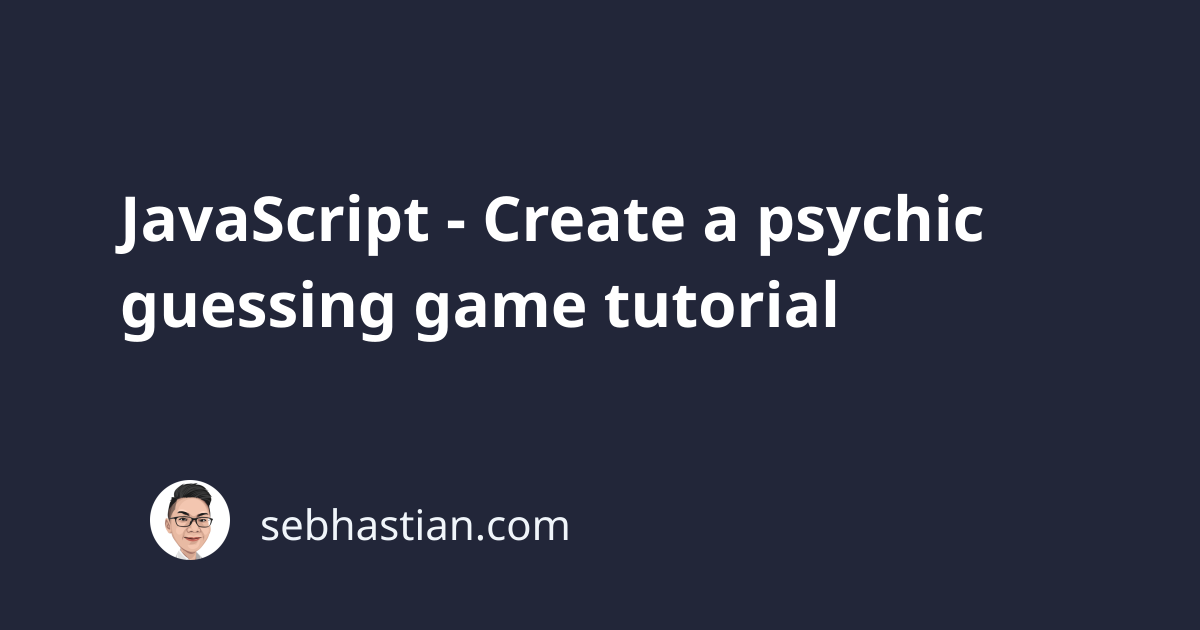
This tutorial will help you to create a psychic game where you can guess a number that’s randomly picked by the code.
You can view the demo of the psychic game here.
First, you need to create the HTML <body>
tag with some instructions as follows:
<body>
<h1>The Psychic Game</h1>
<p>Guess what letter I'm thinking of! Press any letter to begin.</p>
<div id="currentSituation"></div>
</body>
The <div id="currentSituation">
will be used to update the user with the current game situation later.
Next, you need to write JavaScript code that stores all possible answer in an array as follows:
const possibleAnswers = ['a','b','c','d','e','f','g','h','i',
'j','k','l','m','n','o','p', 'q','r',
's','t','u','v','w','x','y','z'];
You also need to store the current status of the game using variables as follows:
let wins = 0;
let losses = 0;
let chances = 9;
let guesses = [];
The user will have 9 chances
to guess the correct letter. All wins
and losses
along with all guesses
in the current session will be recorded.
Then, you need a function that will pick a random letter from the list of possibleAnswers
and keep it as the answer for the current session.
Here’s how you can do so:
function getNewAnswer(){
let randomIndex = Math.floor(Math.random() * possibleAnswers.length);
return possibleAnswers[randomIndex];
}
let answer = getNewAnswer();
The getNewAnswer()
function above will return a random letter from the letters contained in the possibleAnswers
array. The letter will be saved in the answer
variable, which will be reset once a session of the game is over.
Now that you have the answer
, you need to run JavaScript code every time the user presses a key. This can be done by adding a listener to the keydown
event, which will be triggered each time a key is pressed:
document.addEventListener('keydown', logKey);
function logKey(e) {
console.log(`The pressed key: ${e.key}`);
}
Using the example code above, you can create a function that processes the user’s guess each time a key is pressed. Let’s call the function processGuess()
:
document.addEventListener('keydown', processGuess);
Time to write the processGuess()
function code. First, you need to get the event
object passed by the event listener and fetch the key
pressed by the user.
Since we need to compare only lowercase letters, use the toLowerCase()
string method to convert the key to lowercase:
function processGuess(event){
const userGuess = event.key.toLowerCase();
}
Once you get the letter guessed by the user, check if the letter is between a-z
in lowercase and it has never been guessed before. If the letter hasn’t been guessed before, save it inside the guesses
array:
function processGuess(event){
const userGuess = event.key.toLowerCase();
if (/^[a-z]+$/.test(userGuess) && guesses.indexOf(userGuess) == -1) {
guesses.push(userGuess);
}
}
It’s time to process the answer and see if the user guesses the right letter. First, create an if
block to see if the userGuess
value is equal to the answer
value. When it is, you need to do the following:
- Increment the
wins
variable by one - Reset
chances
number back to nine - Reset the
guesses
array to empty alert()
the user that his/ her guess is correct- And get a new
answer
for the next session
Here’s the code to do just that:
function processGuess(event){
const userGuess = event.key.toLowerCase();
if (/^[a-z]+$/.test(userGuess) && guesses.indexOf(userGuess) == -1) {
guesses.push(userGuess);
if (userGuess === answer) {
// the guess is correct
wins++;
chances = 9;
guesses.length = 0;
alert(`That's right! The letter is ${answer}`);
answer = getNewAnswer();
}
}
}
Next, create an else
block to let the user know that the guess was incorrect and reduce the chances
by one:
function processGuess(event){
const userGuess = event.key.toLowerCase();
if (/^[a-z]+$/.test(userGuess) && guesses.indexOf(userGuess) == -1) {
guesses.push(userGuess);
if (userGuess === answer) {
// the guess is correct
wins++;
chances = 9;
guesses.length = 0;
alert(`That's right! The letter is ${answer}`);
answer = getNewAnswer();
} else {
// the guess is wrong
alert(`Not ${userGuess}! Try again`);
chances--;
}
}
}
Finally, create an else if
block to reset the game session and increment the user losses
score by one. This block will be executed when the user chances
value has been reduced to one:
function processGuess(event){
const userGuess = event.key.toLowerCase();
if (/^[a-z]+$/.test(userGuess) && guesses.indexOf(userGuess) == -1) {
guesses.push(userGuess);
if (userGuess === answer) {
// the guess is correct
wins++;
chances = 9;
guesses.length = 0;
alert(`That's right! The letter is ${answer}`);
answer = getNewAnswer();
} else if (chances === 1) {
// all chances are used
losses++;
chances = 9;
guesses.length = 0;
alert(`Whoops you lose! The correct letter is ${answer}`);
answer = getNewAnswer();
} else {
// the guess is wrong
alert(`Not ${userGuess}! Try again`);
chances--;
}
}
}
Now you have covered all possible outcomes from the user’s guess. You only need to update the situation of the game by displaying the previous guess(es), wins, losses and chances left as follows:
let html = `<p>Your previous guess(es): </p>
<p> ${guesses.join(", ")} </p>
<p>wins: ${wins} </p>
<p>losses: ${losses} </p>
<p>chances left: ${chances} </p>`;
document.getElementById("currentSituation").innerHTML = html;
Here’s the complete HTML and JavaScript code in a single page:
<body>
<h1>The Psychic Game</h1>
<p>Guess what letter I'm thinking of! Press any letter to begin.</p>
<p>The right answer is between A to Z.</p>
<div id="currentSituation"></div>
<script>
const possibleAnswers = ['a','b','c','d','e','f','g','h','i',
'j','k','l','m','n','o','p', 'q','r',
's','t','u','v','w','x','y','z'];
let wins = 0;
let losses = 0;
let chances = 9;
let guesses = [];
function getNewAnswer(){
let randomIndex = Math.floor(Math.random() * possibleAnswers.length);
return possibleAnswers[randomIndex];
}
let answer = getNewAnswer();
document.addEventListener("keydown", processGuess);
function processGuess(event){
const userGuess = event.key.toLowerCase();
if (/^[a-z]+$/.test(userGuess) && guesses.indexOf(userGuess) == -1) {
guesses.push(userGuess);
if (userGuess === answer) {
// the guess is correct
wins++;
chances = 9;
guesses.length = 0;
alert(`That's right! The letter is ${answer}`);
answer = getNewAnswer();
} else if (chances === 1) {
// all chances are used
losses++;
chances = 9;
guesses.length = 0;
alert(`Whoops you lose! The correct letter is ${answer}`);
answer = getNewAnswer();
} else if (userGuess !== answer) {
// the guess is wrong
alert(`Not ${userGuess}! Try again`);
chances--;
}
let html = `<p>Your previous choice(s): </p>
<p> ${guesses.join(", ")} </p>
<p>wins: ${wins} </p>
<p>losses: ${losses} </p>
<p>chances left: ${chances} </p>`;
document.querySelector("#currentSituation").innerHTML = html;
}
}
</script>
</body>
You’ve just learned how to create a psychic guessing game using HTML and plain JavaScript 😉
Thank you for reading and I hope you’ve learned something new from this tutorial.