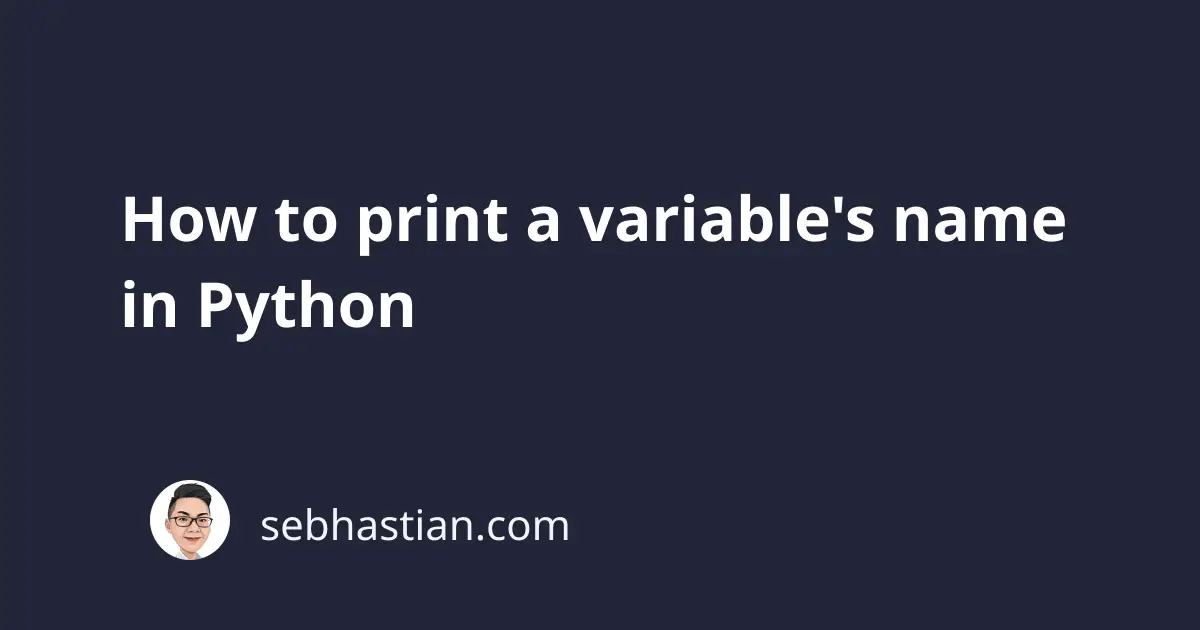
Python doesn’t provide a function to print a variable’s name, so you need to use a custom solution if you want to print the name of a variable.
There are 3 different ways you can print a variable’s name in Python:
- Using the f-string format and
split()
method - Using the
globals()
function - Using the
locals()
function
This tutorial will show you how to use the above methods in practice.
1. Use the f-string format
In Python version 3.8, the f-string format can now be used to print a variable’s name and value by adding the assignment =
operator after the variable’s name.
Consider this example:
name = "Nathan"
print(f"{name=}")
Output:
name='Nathan'
Knowing this feature, you can use the split()
method and get the first item in the resulting list to get a variable’s name.
Here’s an example:
name = "Nathan"
print(f"{name=}".split('=')[0])
Output:
name
The .split('=')
method will split the string returned by the f-string
format, so you get a list of two items: the name of the variable and the value.
From there, you only need to get the variable name using the subscript notation [0]
.
And that’s how you can print a variable’s name in Python. Note that this solution only works for Python version 3.8+, so you need to use the other solutions if you need to support older Python versions.
2. Use the globals() function
The Python globals()
function is used to get a dictionary containing the current scope’s global variables.
To show you how the globals()
function work, run the following code:
first_variable = "A"
my_pet = "Leo"
global_dict = globals()
print(global_dict)
You should see the two variables first_variable
and my_pet
listed in the global_dict
object as follows:
{
'__cached__': None,
'first_variable': 'A',
'my_pet': 'Leo',
'global_dict': {...}
}
Knowing this, you can return the key of the item that has the same value as the variable.
You can use a list comprehension to iterate over the global dictionary as follows:
first_variable = "A"
global_dict = globals()
the_name = [name for name in global_dict if global_dict[name] == first_variable]
print(the_name)
Output:
['first_variable']
Since a list comprehension returns a list, you need to add the subscript notation [0]
if you want the variable name as a string object.
Note that if you use a for
loop to replace list comprehension, Python will raise the RuntimeError: dictionary changed size during iteration
.
I’m not sure why, but it seems the for
loop add something to the global dictionary object.
If you want to use a for
loop, you need to copy the dictionary as follows:
first_variable = "A"
global_dict = globals()
for key, value in global_dict.copy().items():
if value == first_variable:
print(key)
But I suggest you create a custom function to get the variable name as follows:
def get_name(x):
global_dict = globals()
for key, value in global_dict.items():
if value == x:
return key
first_variable = "A"
my_pet = "Leo"
print(get_name(first_variable))
print(get_name(my_pet))
Output:
first_variable
my_pet
When you call the globals()
function inside a function, iterating over the dictionary object doesn’t raise the RuntimeError.
Use the locals() function
Python also has the locals()
function, which is used to create a dictionary containing the current scope’s local variables.
You can use the locals()
function to get a variable’s name as follows:
first_variable = "A"
local_dict = locals()
for key, value in local_dict.copy().items():
if value == first_variable:
print(key)
The locals()
function works in a similar manner to the globals()
function, but you can’t create a custom function to get a variable’s name using locals()
.
This is because the local scope inside the function won’t store the reference to the variable outside of it. If you run the following code:
def get_name(x):
local_dict = locals()
for key, value in local_dict.items():
if value == x:
return key
first_variable = "A"
my_pet = "Leo"
print(get_name(first_variable))
print(get_name(my_pet))
Output:
x
x
As you can see, the get_name()
function returns the parameter name instead of the actual variable name.
You need to use the globals()
function when creating a custom function to extract variable names.
Conclusion
Usually, you don’t need to print a variable name when developing a Python program.
But you might need to do this when you encounter a bug in your program, so you need to create a workaround since Python doesn’t provide a property that returns the variable name.
In the latest version of Python, you can use the f-string format and split()
method to get the variable name.
If you need to support older Python versions, I recommend you create a custom function using the globals()
function as shown in this tutorial.
I hope this tutorial has been useful. Happy coding and see you another time! 👋