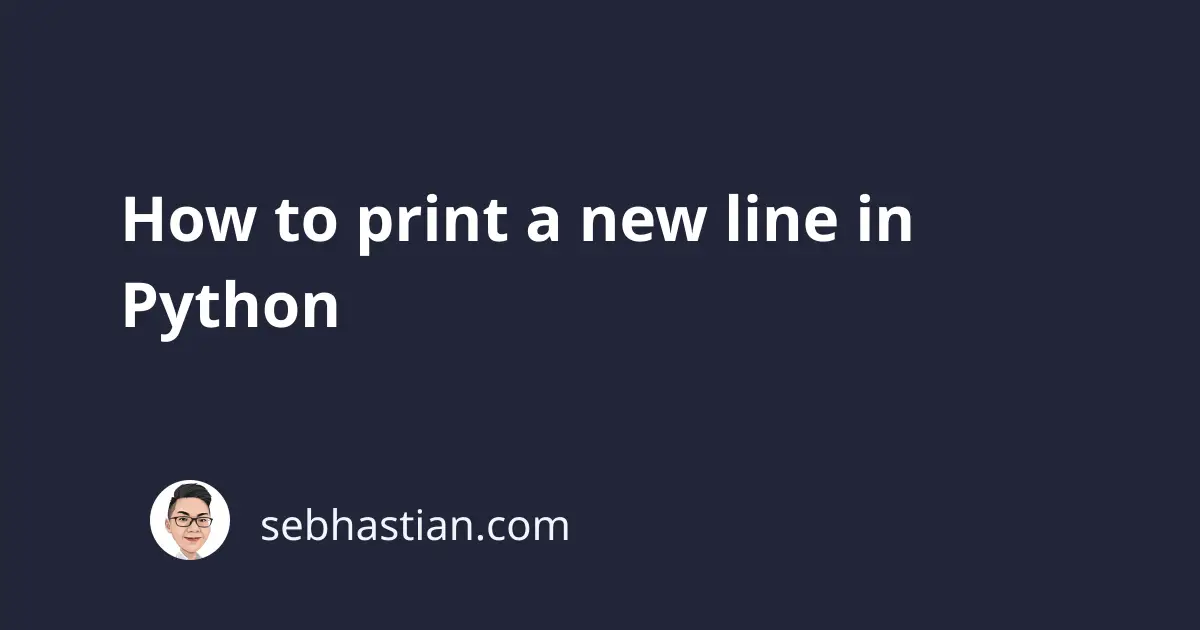
The new line \n
character is used to tell Python when to break a text of string to a new line.
By default, Python already included a new line character by the end of your print
statement.
When you call the print()
function twice as follows:
print("Hello")
print("World")
Then the output will be:
Hello\n
World
But the new line character is never literally printed to the terminal because the Operating System knows how to handle it.
If you want to print multiple lines without calling the print()
function multiple times, you can add the new line \n
character in your string argument.
Here’s an example:
print("Hello\nWorld")
That single print statement will print two lines as follows:
Hello
World
You can also use this new line when you’re using a for
loop as follows:
names = ["Amy", "Andy", "Max"]
my_str = ""
for name in names:
my_str += f"Hello, {name}!\n"
print(my_str)
Output:
Hello, Amy!
Hello, Andy!
Hello, Max!
The print()
function in Python accepts an argument named end
that specifies what to print at the end of the string you printed.
By default, the value of end
is \n
. If you want to print without a new line in Python, you can change this argument to a space like this:
names = ["Amy", "Andy", "Max"]
for name in names:
print(name, end=" ")
# Output: Amy Andy Max
As you can see, the print()
function is quite flexible that you can decide when to print without appending a new line at the end of the string.
I hope this tutorial is helpful. Happy coding! 👍