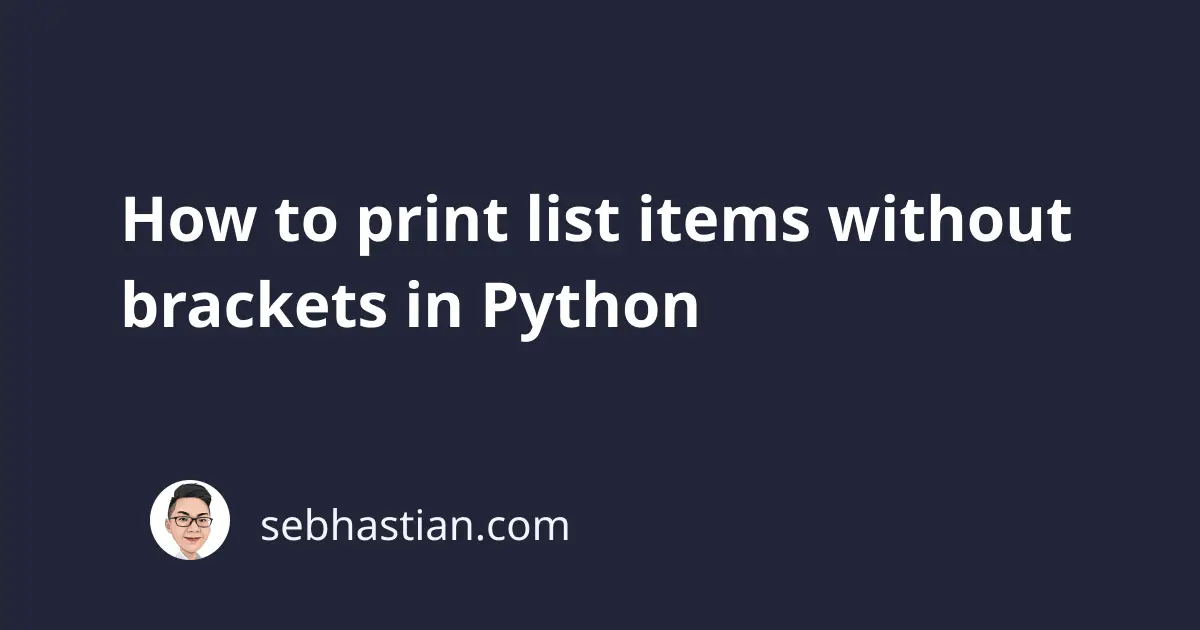
Sometimes, you want to take a Python list like this:
my_list = ['Jack', 'Sam', 'Amy', 'Dan']
And print it without brackets as follows:
Jack, Sam, Amy, Dan
There are two ways you can print a list without brackets in Python:
- Call the
str.join()
method on a comma - Use the asterisk
*
symbol to unpack the list
This tutorial will show you how to code both methods above in practice.
1. Call the str.join() method
To print a list without brackets, you need to call the join()
method on a comma as follows:
my_list = ['Jack', 'Sam', 'Amy', 'Dan']
print(', '.join(my_list))
Output:
Jack, Sam, Amy, Dan
The join()
method takes the string from which you call the method and insert that string in between each item on the list.
When you call the print()
function, the list will be printed without the square brackets because it’s no longer a list but a string.
One limitation of this solution is that you can only print a list of strings.
If you have other types like integers or booleans, the solution will raise an error. For example:
my_list = [1, 2, 3, True, False]
print(', '.join(my_list))
Output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
print(', '.join(my_list))
TypeError: sequence item 0: expected str instance, int found
As you can see, the join()
method expects a string, but it gets an int instead.
To overcome this limitation, you need to use the second solution explained below.
2. Use the asterisk *
symbol to unpack the list
The second way to print a list without brackets is to add the asterisk *
symbol when passing the list to the print()
function.
You also need to specify a comma as the separator sep
argument for this solution to work:
my_list = ['Jack', 'Sam', 'Amy', 'Dan']
print(*my_list, sep=', ')
Output:
Jack, Sam, Amy, Dan
As you can see, using the *
symbol makes Python print each element in the list instead of the list itself.
You need to change the separator to a comma or Python will use a single white space for the separator:
my_list = ['Jack', 'Sam', 'Amy', 'Dan']
print(*my_list)
Output:
Jack Sam Amy Dan
The benefit of this method is that you can print a list that stores all available types in Python.
You can print a combination of int and boolean types as follows:
my_list = [1, 2, 3, True, False]
print(*my_list, sep=', ')
Output:
1, 2, 3, True, False
When using the str.join()
method, this kind of list will cause an error.
And that’s how you print a list without brackets in Python. Happy coding! 👍