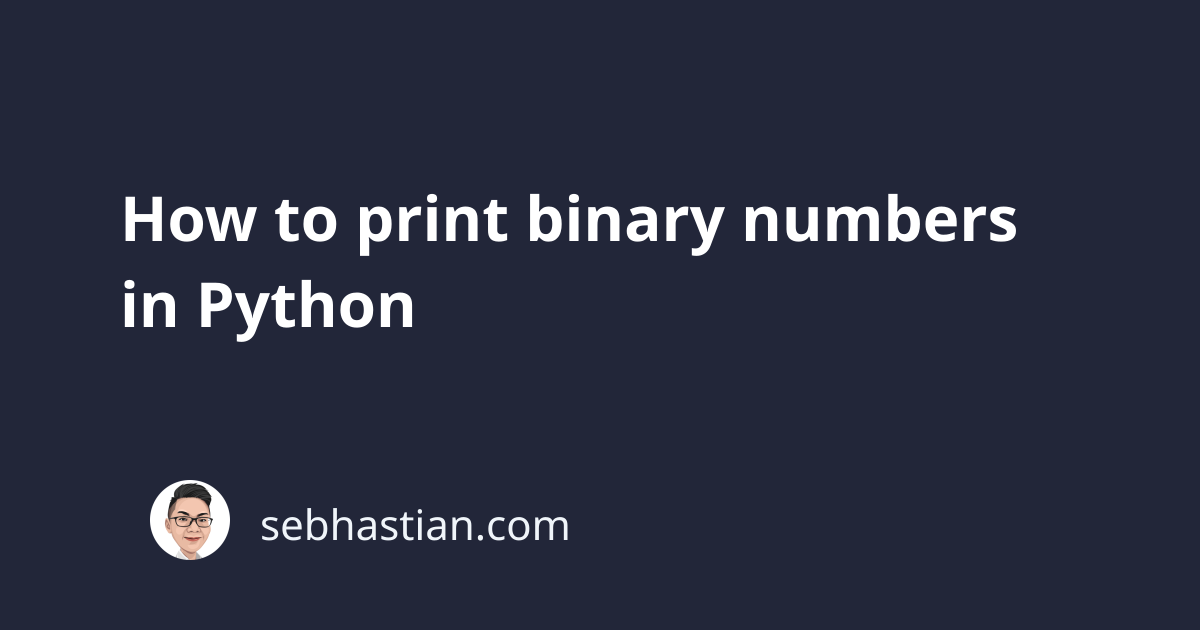
To print binary numbers in Python, you can call the bin()
function which returns the binary representation of an integer number you passed as its argument.
Here’s an example of converting an integer number to binary:
my_int = 17
print(bin(my_int))
Output:
0b10001
The bin()
function always includes the 0b
prefix in the returned binary string. If you don’t need the prefix, you can slice the string to remove it.
my_int = 17
print(bin(my_int)[2:])
Output:
10001
But this method doesn’t work when you have a negative number as it only removes the -0
literal as follows:
my_int = -17
my_bin = bin(my_int)[2:]
print(my_bin)
print(int(my_bin, 2))
Output:
b10001
Traceback (most recent call last):
File "main.py", line 6, in <module>
print(int(my_bin, 2))
ValueError: invalid literal for int() with base 2: 'b10001'
To create a solution that works with both positive and negative numbers, you need to use the str.replace()
method to remove the 0b
literal.
The code below uses the replace()
method to remove the 0b
literal, then convert it back to an integer using the int()
function:
my_int = 17
my_bin = bin(my_int).replace("0b", "")
print(f"Binary: {my_bin}")
# convert binary base 2 to base 10 integer
print(f"Back to int: {int(my_bin, 2)}")
Output:
Binary: 10001
Back to int: 17
This method also works when you have a negative number as shown below:
my_int = -170
my_bin = bin(my_int).replace("0b", "")
print(f"Binary: {my_bin}")
print(f"Back to int: {int(my_bin, 2)}")
Output:
Binary: -10101010
Back to int: -170
And that’s how you print binary numbers in Python. I hope this tutorial is useful.
Stay awesome and keep coding! 👍