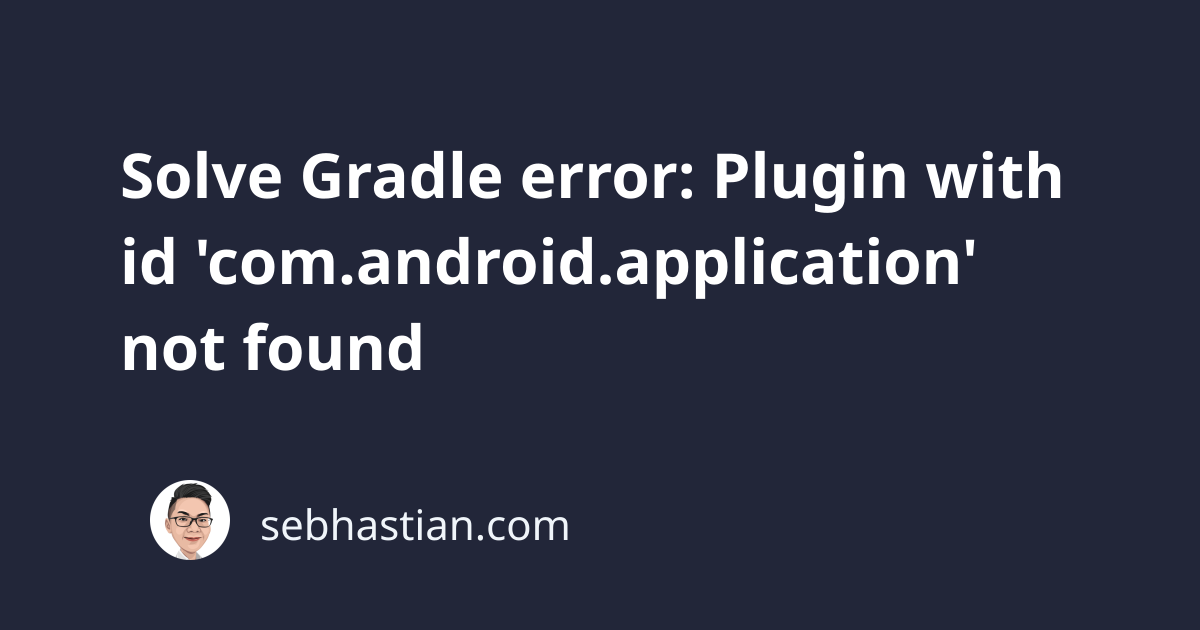
When building your Android application in Android studio, an error may occur saying Plugin with id ‘com.android.application’ was not found.
The error looks as follows:
* Exception is:
org.gradle.api.plugins.UnknownPluginException:
Plugin [id: 'com.android.application'] was not found
in any of the following sources:
The UnknownPluginException
means that Gradle could not find the Android Gradle plugin required to build your application successfully.
To resolve this error, you need to check three things:
- Check that Google Maven repository is listed in your
settings.gradle
file - Check the Android Gradle plugin is added to the project
build.gradle
file - Check the Android Gradle plugin is compatible with your Gradle version
Let’s learn how to resolve this issue together.
Check your settings.gradle file
The Android Gradle plugin is downloaded from Google’s Maven repository. You need to make sure that the repository google()
is listed under pluginManagement
in your Android project.
The settings.gradle
file is the file used to list repositories where Gradle will look to download dependencies for your Android project.
The content of the file should look as follows:
pluginManagement {
repositories {
gradlePluginPortal()
google()
mavenCentral()
}
}
dependencyResolutionManagement {
repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS)
repositories {
google()
mavenCentral()
}
}
rootProject.name = "KotlinApp"
include ':app'
If you only see the following line in settings.gradle
:
include ':app'
Then it means your Android project was created a long time ago.
The repositories for your Android project should be located in build.gradle
file instead. Let’s go there next.
Check your build.gradle file
An Android application project has two build.gradle
files:
<project>/app/build.gradle
is specific configuration for theapp
module<project>/build.gradle
is the global configuration for your current Android project
The <project>/build.gradle
file should contain the dependency to the Android Gradle plugin and its version as shown below:
plugins {
id 'com.android.application' version '7.1.2' apply false
id 'com.android.library' version '7.1.2' apply false
id 'org.jetbrains.kotlin.android' version '1.6.21' apply false
}
task clean(type: Delete) {
delete rootProject.buildDir
}
Make sure that your build.gradle
file is similar to the one shown above.
For older Android application projects, the build.gradle
file will also contain the repository list as shown below:
buildscript {
repositories {
jcenter()
}
dependencies {
classpath 'com.android.tools.build:gradle:2.1.0'
}
}
allprojects {
repositories {
jcenter()
}
}
task clean(type: Delete) {
delete rootProject.buildDir
}
If you have similar content to the one above, then you need to replace jcenter()
with mavenCentral()
and google()
.
This is because JCenter repository has become read-only since March 31st, 2021. You can still download modules from the repo, but there will be no new version release there.
Additionally, you need to update your Android Gradle dependency to the latest version as shown below:
buildscript {
repositories {
google()
mavenCentral()
}
dependencies {
classpath 'com.android.tools.build:gradle:7.1.2'
}
}
allprojects {
repositories {
google()
mavenCentral()
}
}
task clean(type: Delete) {
delete rootProject.buildDir
}
Now your Android Gradle Plugin version and the repository list is up to date.
The last thing to check is the Gradle version used by your Android project.
Check your Gradle version
Every Android project generated using Android Studio contains a gradle-wrapper.properties
file.
The file is located inside gradle/wrapper/
folder, and it defines the Gradle version used by your project.
Here’s an example of the gradle-wrapper.properties
file content:
distributionBase=GRADLE_USER_HOME
distributionPath=wrapper/dists
zipStorePath=wrapper/dists
zipStoreBase=GRADLE_USER_HOME
distributionUrl=https\://services.gradle.org/distributions/gradle-7.2-bin.zip
The distributionUrl
property highlighted above shows the version of Gradle used by your Android project.
gradle-7.2-bin.zip
means the project is using Gradle version 7.2
.
You need to use the Gradle version that supports your Android Gradle plugin version
Here’s the compatibility list between Gradle and Android Gradle:
As you have updated Android Gradle to version 7.1.2
before, you should use Gradle version 7.2+
as stated in the table.
To update your Gradle version, just change the version in the .zip
file name:
gradle-7.0-bin.zip
gradle-7.2-bin.zip
gradle-7.3-bin.zip
Keep in mind that you also need to check the Android Studio version, because older Android Studio doesn’t support the latest Android Gradle plugin (link).
Once the Gradle version is updated, build your Android application again. The issue with com.android.application
should now be resolved.
Conclusion
Now you’ve learned how to resolve the issue com.android.application
not found in Android Studio.
As Android OS gets new releases and adds new features, older versions of Gradle and Android Gradle will become obsolete.
When you import a project into Android Studio, the first build should warn you of any incompatibility in the Gradle build process.
Sometimes, you need to update Gradle and Android Gradle versions before you can build the project successfully.
In some cases, you might see an Android project with missing Gradle files. To fix it, you can try creating a new project with Android Studio.
When the new project builds successfully, copy the Gradle files and gradle/
folder to your old Android project:
After that, change the rootProject.name
attribute in your settings.gradle
file to match the old project.
I hope this tutorial has been useful for you. 🙏