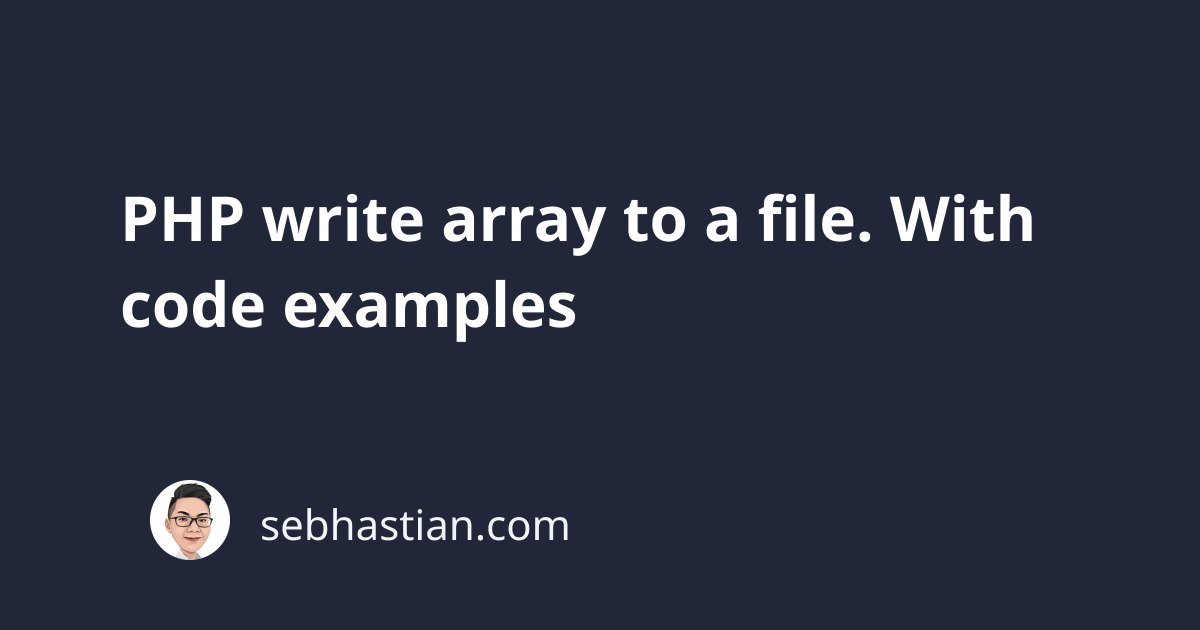
To write a PHP array to a file, you need to call the file_put_contents()
function in combination with the print_r()
or var_export()
function.
Let’s see an example of how to write PHP array to a file.
Suppose you have an array as follows:
<?php
$user = array (
"name" => "Nathan",
"age" => "29",
"skills" => array ("JavaScript", "PHP", "Python")
);
You can print the $user
above with the following code:
file_put_contents("output.txt", print_r($user, true));
In the above code, the file_put_contents()
function look for a file named output.txt
. When output.txt
isn’t found, the function will create one.
The print_r()
function is used for printing information about a variable in a human-readable format.
With the second parameter set to true
, the function will return the output instead of printing it.
Because of that, the print_r()
output will be written to the output.txt
file.
Here’s the content of the output.txt
file:
Array
(
[name] => Nathan
[age] => 29
[skills] => Array
(
[0] => JavaScript
[1] => PHP
[2] => Python
)
)
You can also use the var_export()
function as an alternative to the print_r()
function like this:
<?php
$user = array (
"name" => "Nathan",
"age" => "29",
"skills" => array ("JavaScript", "PHP", "Python"));
file_put_contents("output.txt", var_export($user, true));
The var_export()
function will print the array in a slightly different format as shown below:
array (
'name' => 'Nathan',
'age' => '29',
'skills' =>
array (
0 => 'JavaScript',
1 => 'PHP',
2 => 'Python',
),
)
Personally, I think the print_r()
function is more readable than var_export()
. But you’re free to use one of them in your code.
Finally, you can also add the FILE_APPEND
flag to the file_put_contents()
function to make it append the output instead of overwriting it.
By default, file_put_contents()
overwrite any text written in your file. Adding the FILE_APPEND
flag will make it append the text instead.
Suppose you write two arrays to the output.txt
file as follows:
$user = array (
"name" => "Nathan",
"age" => "29",
"skills" => array ("JavaScript", "PHP", "Python"));
file_put_contents("output.txt", print_r($user, true));
$user = array (
"name" => "Jane",
"age" => "24",
"skills" => array ("HTML", "CSS"));
file_put_contents("output.txt", print_r($user, true), FILE_APPEND);
The output in the text file is as follows:
Array
(
[name] => Nathan
[age] => 29
[skills] => Array
(
[0] => JavaScript
[1] => PHP
[2] => Python
)
)
Array
(
[name] => Jane
[age] => 24
[skills] => Array
(
[0] => HTML
[1] => CSS
)
)
Without the FILE_APPEND
flag, the first array will be overwritten.
Now you’ve learned how to write PHP array to a file. Nice work! 👍