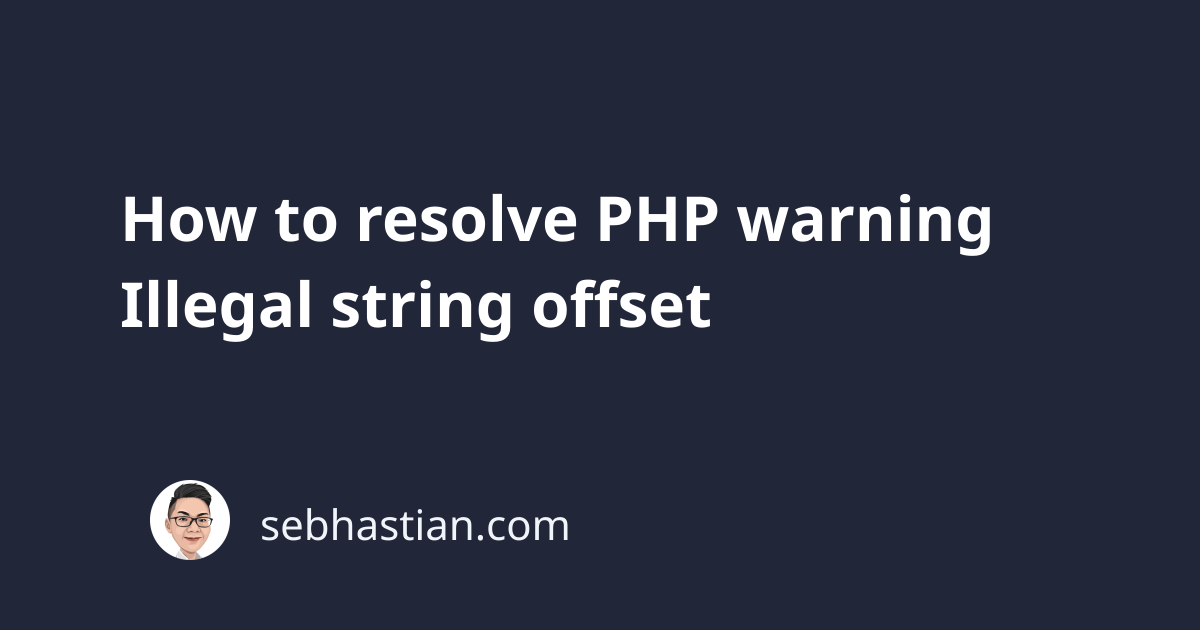
The PHP warning: Illegal string offset happens when you try to access a string
type data as if it’s an array
.
In PHP, you can access a character from a string
using the bracket symbol as follows:
$myString = "Hello!";
// 👇 access string character like an array
echo $myString[0]; // H
echo $myString[1]; // e
echo $myString[4]; // o
When you try to access $myString
above using an index that’s not available, the PHP warning: Illegal string offset occurs.
Here’s an example:
$myString = "Hello!";
// 👇 these cause illegal offset
echo $myString["state"]; // PHP Warning: Illegal string offset 'state' in..
echo $myString["a"]; // PHP Warning: Illegal string offset 'a' in ..
To resolve the PHP Illegal string offset warning, you need to make sure that you are accessing an array instead of a string.
To check if you’re actually accessing an array, you can call the is_array()
function before accessing the variable as follows:
$myArray = [
"state" => true,
"port" => 1331,
];
// 👇 check where myArray is really an array
if (is_array($myArray)) {
echo $myArray["port"];
echo $myArray["L"];
}
This warning will also happen when you have a multi-dimensional PHP array where one of the arrays is a string
type.
Consider the following example:
$rows = [
["name" => "John"],
"",
["name" => "Dave"]
];
foreach ($rows as $row) {
echo $row["name"]. "\n";
}
The $rows
array has a string at index one as highlighted above.
When you loop over the array, PHP will produce the illegal string offset as shown below:
John
PHP Warning: Illegal string offset 'name' in ...
Dave
To avoid the warning, you can check if the $row
type is an array before accessing it inside the foreach
function:
foreach ($rows as $row) {
if(is_array($row)){
echo $row["name"]. "\n";
}
}
When the $row
is not an array, PHP will skip that row and move to the next one.
The output will be clean as shown below:
John
Dave
When you see this warning in your code, the first step to debug it is to use var_dump()
and see the output of the variable:
var_dump($rows);
From the dump result, you can inspect and see if you have an inconsistent data type like in the $rows
below:
array(3) {
[0]=>
array(1) {
["name"]=>
string(4) "John"
}
[1]=>
string(0) ""
[2]=>
array(1) {
["name"]=>
string(4) "Dave"
}
}
For the $rows
variable, the array at index [1]
is a string instead of an array.
Writing an if
statement and calling the is_array()
function will be enough to handle it.
And that’s how you solve the PHP warning: Illegal string offset. Good luck! 👍