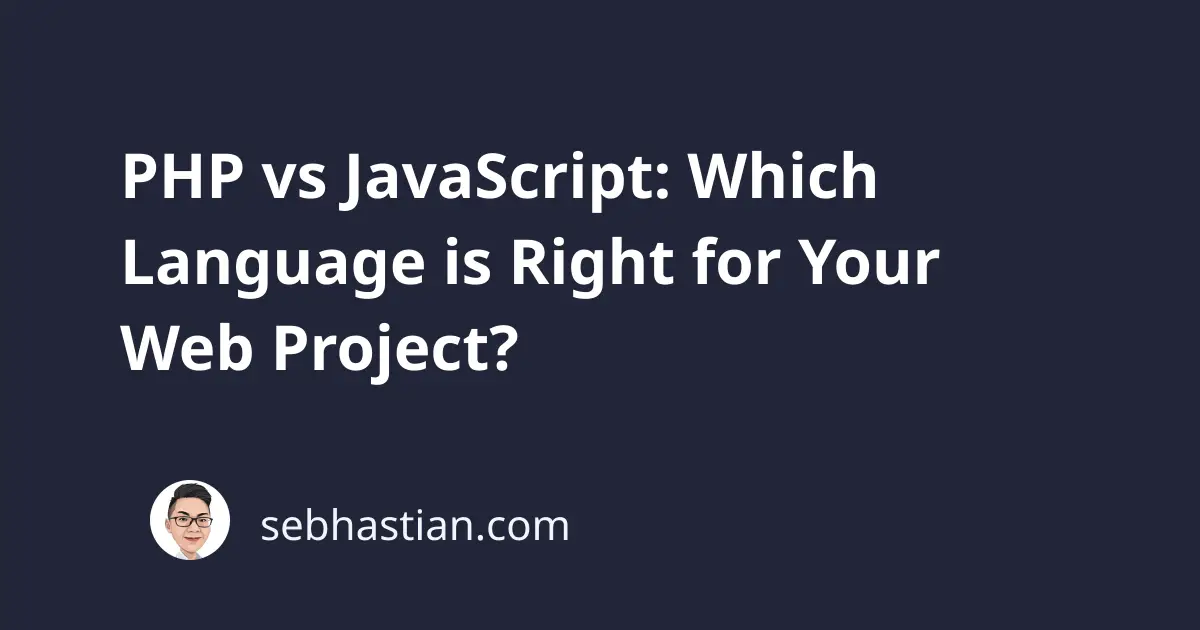
PHP and JavaScript are two of the most popular and widely used programming languages for web development.
Both languages have a long history and have evolved significantly over the years, providing developers with a wide range of tools and capabilities for building dynamic and interactive websites.
PHP, which stands for “Hypertext Preprocessor,” is a server side scripting language that is commonly used for creating web applications and content management systems.
It is often integrated with other technologies such as databases and web servers to create powerful and scalable web solutions.
JavaScript, on the other hand, is a client side scripting language that is used to add interactive and dynamic elements to web pages.
It is typically executed in the user’s web browser, allowing developers to create responsive and engaging user interfaces without the need for constant communication with the server.
Nowadays, JavaScript can also serve as the server side language, allowing developers to use only JavaScript to create a powerful web application.
In this article, we will compare the features, capabilities, and performance of PHP and JavaScript, and provide some guidance on when to use each language in web development.
History of PHP and JavaScript
PHP was first created in 1994 by Rasmus Lerdorf as a set of Common Gateway Interface (CGI) programs written in the C programming language.
He named the project “Personal Home Page” because it was originally intended for his own use in maintaining his personal homepage.
Over time, other developers contributed to the project and it evolved into a full-fledged scripting language with support for object-oriented programming and other modern features.
JavaScript, on the other hand, was created in 1995 by Brendan Eich while he was working at Netscape Communications.
It was originally known as LiveScript, but was later renamed to JavaScript to capitalize on the popularity of Java, a programming language developed by Sun Microsystems.
JavaScript has also evolved significantly over the years, with new versions introducing features such as classes, modules, and asynchronous programming.
Both PHP and JavaScript are now widely used in web development, and have large and active communities of developers who contribute to their ongoing evolution.
They are both supported by a wide range of tools, libraries, and frameworks that make it easier to build web applications with these languages.
Syntax and semantics of PHP and JavaScript
PHP and JavaScript have some similarities in their syntax, but there are also some significant differences.
Both languages use the same basic data types, such as numbers, strings, and booleans, and support variables and control structures such as if-else
statements and for
loops.
Here are some examples of the syntax differences between PHP and JavaScript:
PHP uses the php
tag to enclose its code. This tag lets the PHP interpreter knows which part of the code needs to be executed as PHP code.
Consider the following example to print the text “Hello, world!” in PHP:
<?php
echo "Hello, world!";
?>
In JavaScript, the code is typically included directly in the HTML of a web page with the <script>
element, and is executed automatically when the page is loaded.
To print the text “Hello, world!” in JavaScript, you would use the following code:
<script>
alert("Hello, world!");
</script>
If you run JavaScript on the server side or using the .js
file, you don’t need the <script>
tag to write JavaScript code.
In PHP, functions are defined using the function
keyword, and are called by using the function name followed by a pair of parentheses.
For example, the following code defines a function named hello()
that takes a single argument and prints a greeting:
<?php
function hello($name) {
echo "Hello, $name";
}
hello("Nathan"); // Output: Hello, Nathan
?>
In JavaScript, functions are defined and called in the same way as in PHP:
<script>
function hello(name) {
alert("Hello, " + name);
}
hello("Leyla"); // Output: Hello, Leyla
</script>
In PHP, variables are declared using the $
symbol followed by the variable name.
The data type of the variable is determined automatically based on the value that is assigned to it.
For example, the following code declares a variable named $name and assigns it the string value “John”:
<?php
$name = "John";
?>
In JavaScript, variables are declared using the var
keyword, and the data type is determined automatically like in PHP.
For example, the following code declares a variable named name and assigns it the string value “John”:
<script>
var name = "Nathan";
</script>
In PHP, comments are written using the #
or //
symbols for a single-line comment and /* */
for a multi-line comment.
For example, the following code contains a single-line comment and a multi-line comment:
<?php
# This is a single-line comment
/*
This is a multi-line comment
that can span multiple lines
*/
?>
In JavaScript, comments are written using the //
or /* */
symbols:
<script>
// This is a single-line comment
/*
This is a multi-line comment
that can span multiple lines
*/
</script>
In PHP, control structures such as if
statements and for
loops use a similar syntax to other programming languages.
For example, the following code uses an if
statement to check a condition and a for
loop to iterate over an array:
<?php
if ($name == "John") {
echo "Hello, John!";
}
for ($i = 0; $i < count($names); $i++) {
echo $names[$i];
}
?>
Controls structures and loops in JavaScript use a similar syntax:
<script>
if (name == "John") {
alert("Hello, John!");
}
for (var i = 0; i < names.length; i++) {
alert(names[i]);
}
</script>
Finally, semicolons are mandatory to end a statement in PHP, while it’s optional in JavaScript.
This is because JavaScript has an Automatic Semicolon Insertion mechanism that adds the semicolon before execution.
Overall, the syntax and semantics of PHP and JavaScript are similar in many ways.
But they have noticeable differences in declaring variables and semicolon insertion.
Popularity
JavaScript is the most popular language in the world because it’s the only language that can be used in the browser.
PHP is a server side language, and it has competitions with Python, Ruby, Golang, C#, Java, and JavaScript.
This is why it’s natural for JavaScript to be more popular than PHP.
According to StackOverflow Developer Survey in 2022, JavaScript has been the most commonly used programming language for 10 years:
In contrast, PHP is the ninth most popular language, behind other server side languages like Java and C#.
Typical use cases and integration
PHP and JavaScript are typically used for different kinds of web development projects.
This is because the solutions offered by the developer community tend to be used for special use cases.
For example, PHP has ready-made CMS (Content Managemen System) that you can customize like WordPress and Drupal.
On the other hand, JavaScript has static site generators like Gatsby and VuePress.
For a full-stack solution, PHP has the LAMP (Linux, Apache, MySQL, PHP) stack.
JavaScript has MERN, MEAN, and MEVN stacks for a full-stack solution.
PHP is commonly used for creating content management systems, e-commerce platforms, and other web applications that require a high level of server side processing.
JavaScript is commonly used for creating user interfaces that are responsive and engaging. You can integrate web technologies such as Google Maps and Facebook Login to add additional features to your application.
Additionally, JavaScript can also be used to develop mobile applications using frameworks like Ionic and React Native.
Performance and scalability
PHP and JavaScript have similar performance characteristics when they are used on the server side.
Node.js has some advantages over PHP due to its asynchronous and event-driven architecture.
It can handle a large number of concurrent connections and requests without the need for expensive thread management, which makes it well-suited for applications that need to handle high volumes of data or traffic.
But PHP also has great performance, as it can take advantage of the server’s resources, such as memory and CPU, to perform complex operations quickly.
PHP also has a number of performance-enhancing features, such as opcode caching and bytecode optimization, that can further improve its speed and efficiency.
On the other hand, client side JavaScript is executed in the user’s web browser, which means that it is subject to the limitations of the client device.
JavaScript can be slowed down by factors such as the speed of the user’s internet connection, the amount of available memory and CPU, and the number of other scripts that are running in the browser.
Conclusion: When to use PHP vs JavaScript
PHP and JavaScript are two popular programming languages for web development, but they are typically used in different kinds of web projects.
You are recommended to use PHP when your project requires CMS solutions like WordPress and Drupal.
But if you require a static site generator, it’s better to use JavaScript.
When you need a powerful client side application, you can use JavaScript libraries like React, Vue, or Angular.
If you require a full-stack solution, you can use what you prefer (LAMP or MERN/ MEVN/ MEAN.)
Also, PHP has web development frameworks like Laravel and Yii, while JavaScript has Angular and Astro.
In terms of performance and scalability, both PHP and JavaScript are capable of handling large amounts of data and traffic.
The choice of PHP or JavaScript for a web project should be based on the specific requirements of the application and the preferences of the development team.
Both languages have their strengths and weaknesses, and can be used effectively in different scenarios to develop a scalable web application.
But keep in mind that even when you decide to use PHP, you may still write JavaScript for the frontend development.
Now you’ve learned about the differences between PHP and JavaScript. Good work!