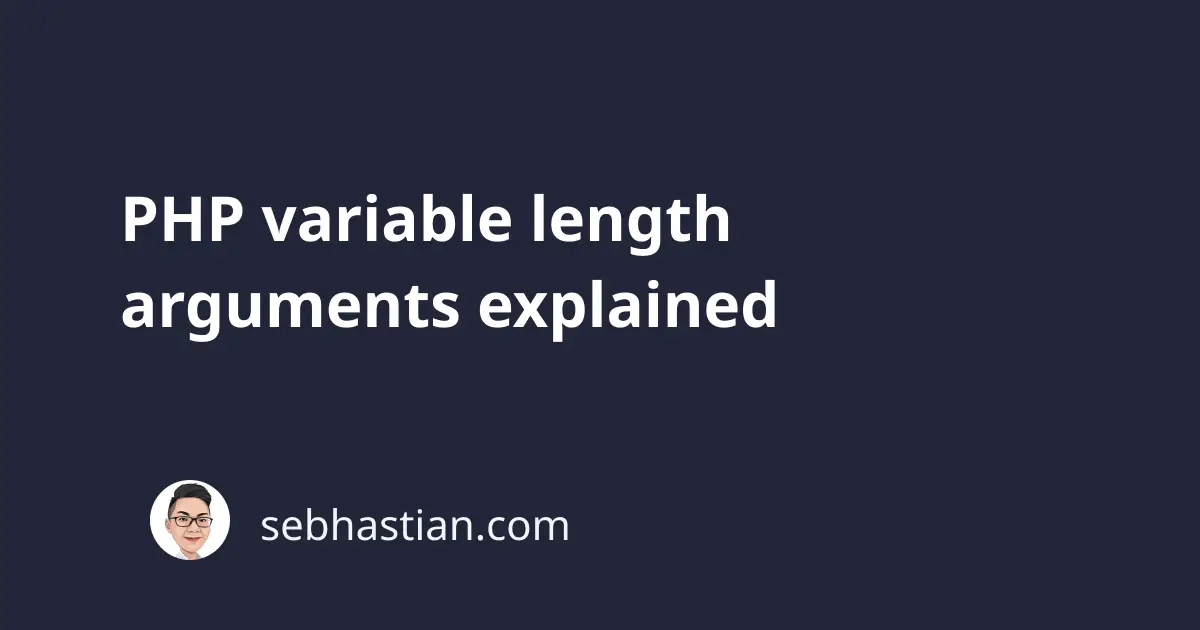
PHP variable length arguments is a feature that allows a function to accept a variable number of arguments.
With this feature, you can define a function that accepts an (x) number of arguments. That (x) can be 0
, 1
, or as many arguments as you want.
To use this feature, you need to add 3 dots ...
before you define the parameter.
Below is an example of defining a variable length argument called $numbers
:
<?php
function sum(...$numbers) {
$total = 0;
foreach ($numbers as $n) {
$total += $n;
}
return $total;
}
print sum(1, 2, 3, 4); // 10
print sum(); // 0
?>
The variable length argument will be put in an array, and you can use that array however you want inside the function.
You can also define the type of the variable length argument before the ...
token.
The following example shows how to define a variable length argument of type string
:
<?php
function print_words(string ...$strings) {
foreach ($strings as $s) {
print $s . "\n";
}
}
print_words("Hello", "Good morning!", "Good night!");
// Hello
// Good morning!
// Good night!
print_words(null);
// Fatal error: Uncaught TypeError
?>
Using the variable length argument, you can create a function that accepts a dynamic number of arguments.
When used correctly, this feature allows you to develop a sophisticated function.