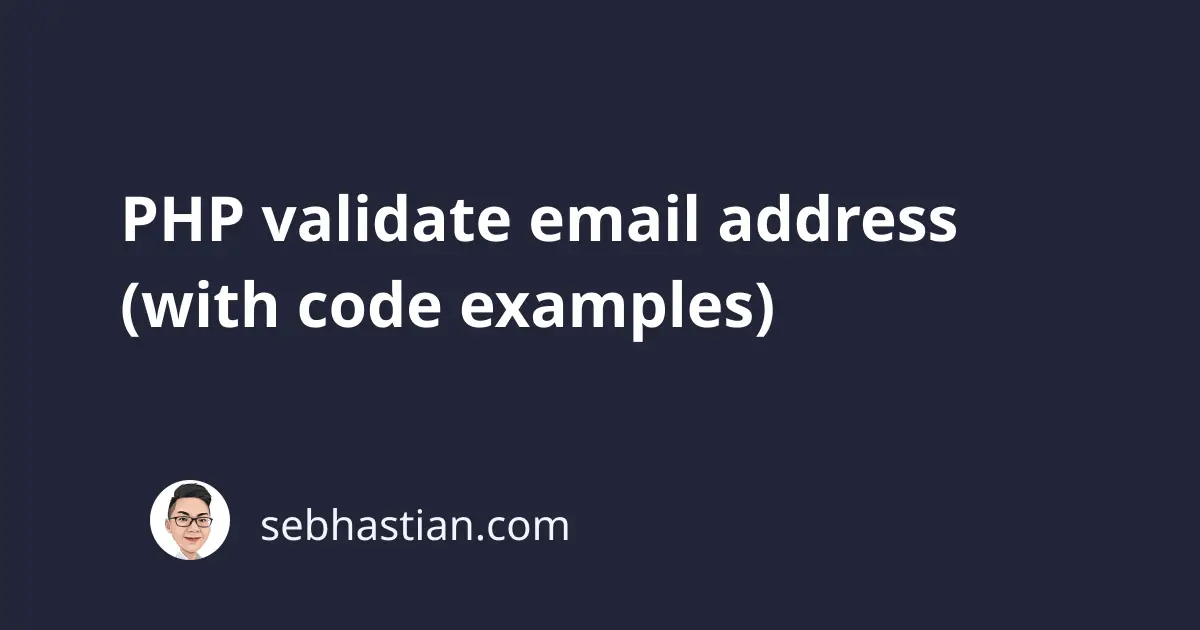
Validating an email address is one of the most common tasks required when creating a web application. One way to validate an email address is to use the filter_var
function.
The filter_var
function allows you to validate various types of data, including email addresses, using a set of predefined filters.
To validate an email address with filter_var
, you can use the FILTER_VALIDATE_EMAIL
filter as shown below:
<?php
$email = "[email protected]";
if (filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo "Email is valid";
} else {
echo "Email is invalid";
}
The filter_var
function returns true
if the email is valid and false
if it is invalid.
When you receive an email input through a form, you can pass the form data to the filter_var()
function as shown below:
<?php
// check if post contains an email input
if (isset($_POST["email"])) {
// validate the email input
if (filter_var($_POST["email"], FILTER_VALIDATE_EMAIL)) {
echo "Email is valid";
} else {
echo "Email is invalid";
}
}
And that’s how you validate email addresses in PHP. Very easy!
You can use this email validation method when you validate form data, as shown in my PHP form validation article.