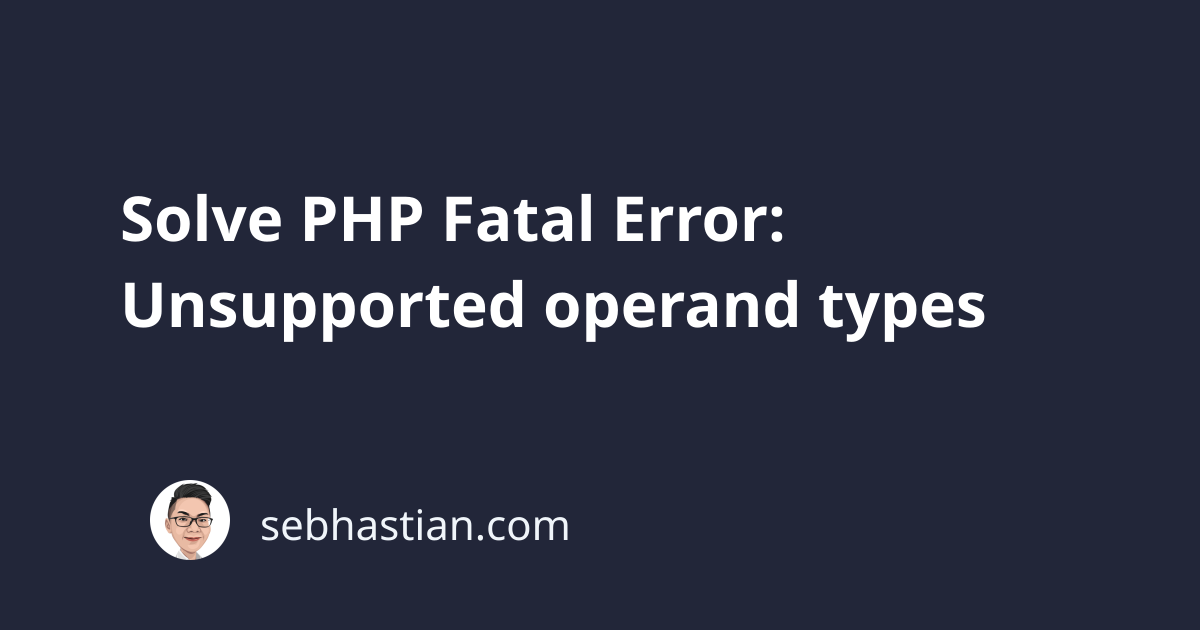
The PHP Fatal Error: Unsupported operand types is an error message that indicates you have used operators on values that are not supported.
PHP operators like +
and -
can be used on int
type values. When you try to combine values like array or string with an int
, the error message will appear:
// 👇 unsupported operand types: string + int
print "Hello" + 29;
This fatal error commonly occurs in PHP v8, where the execution of operands is made stricter.
In PHP v7 or below, the string will be ignored and PHP will print out the int
value:
// 👇 php v7 or below
print "Hello" + 29; // 29
To solve this fatal error, you need to make sure that the operands (the values on the left or right side of the operator) are supported by PHP.
Arithmetic operators like +
, -
, *
, and /
support int
and float
types.
You can’t add an array
and an int
, or a string
and a float
when using these operators:
<?php
$arr = [
"name" => "Orange",
"price" => 5,
];
// 👇 unsupported operands: array + int
$arr + 8;
Even PHP v5 will show “Unsupported operand types” when you add an array
and an int
as in the example above.
When you don’t know how to fix the issue, use var_dump()
to see the type of the variable like this:
<?php
$arr = [
"name" => "Orange",
"price" => 5,
];
var_dump($arr);
The above example will output the content of the $arr
variable:
array(2) {
["name"]=>
string(6) "Orange"
["price"]=>
int(5)
}
Knowing that it’s an array, you can choose to perform addition to the number element, which is the price
element:
<?php
$arr = [
"name" => "Orange",
"price" => 5,
];
// 👇 add to the price element
$arr['price'] += 8;
print $arr['price']; // 13
PHP requires you to use supported operand types for the code.
When you see the message “Fatal Error: Unsupported operand types”, you need to pay attention to the variables used as operands.
They are the variables on the left or right side of the operator symbol. You can find information about them using the var_dump()
function.
Once you know the operand types, adjust your code accordingly to solve this error.
And that’s how you handle PHP Fatal Error: Unsupported operand types. Nice work! 😉