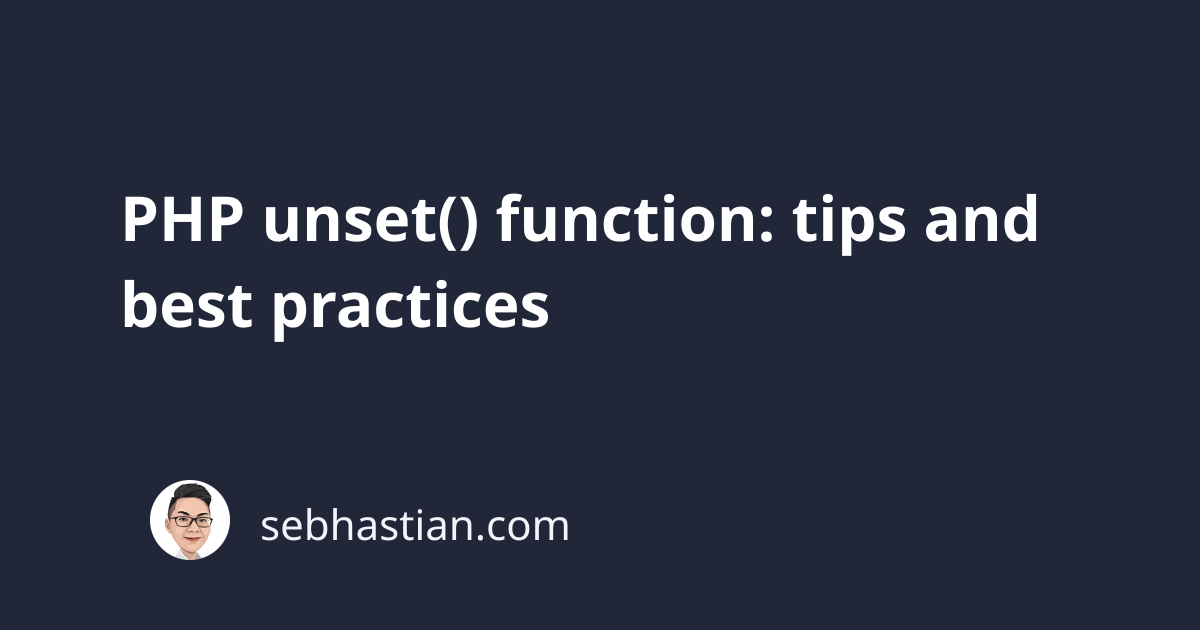
The unset()
function in PHP is used to destroy a variable or an element of an array.
This function is commonly used to free up memory or to remove data that is no longer needed in a script.
To use the unset()
function, you simply need to pass the name of the variable or array element you want to destroy as the argument.
For example, to destroy a simple variable called $name
, you would use the following syntax:
$name = "Nathan";
echo $name . "\n"; // Nathan
unset($name);
echo $name . "\n"; // Warning: Undefined variable $name
The unset()
function completely removes a variable or array element. It’s as if you never declare that variable.
To destroy an element of an array, you need to specify the array name and the key of the element you want to destroy.
Suppose you want to destroy the element with the key “age” in an array called $person
, you would use the following syntax:
// create an array
$person = [
"name" => "Nathan",
"age" => 29,
];
// destroy age element
unset($person["age"]);
print_r($person);
The code above will remove the “age” element, and the print_r()
output is as shown below:
Array
(
[name] => Nathan
)
You can also remove the whole array using the unset()
function by passing the array variable name, without a key:
$person = [
"name" => "Nathan",
"age" => 29,
];
// unset the array
unset($person);
print_r($person); // Warning: Undefined variable $person
In summary, the unset()
function in PHP is used to destroy a variable or an array element.
It is important to use this function carefully, as it can cause errors if you try to use a destroyed variable or array element.
Now you’ve learned how the PHP unset()
function works. Nice!