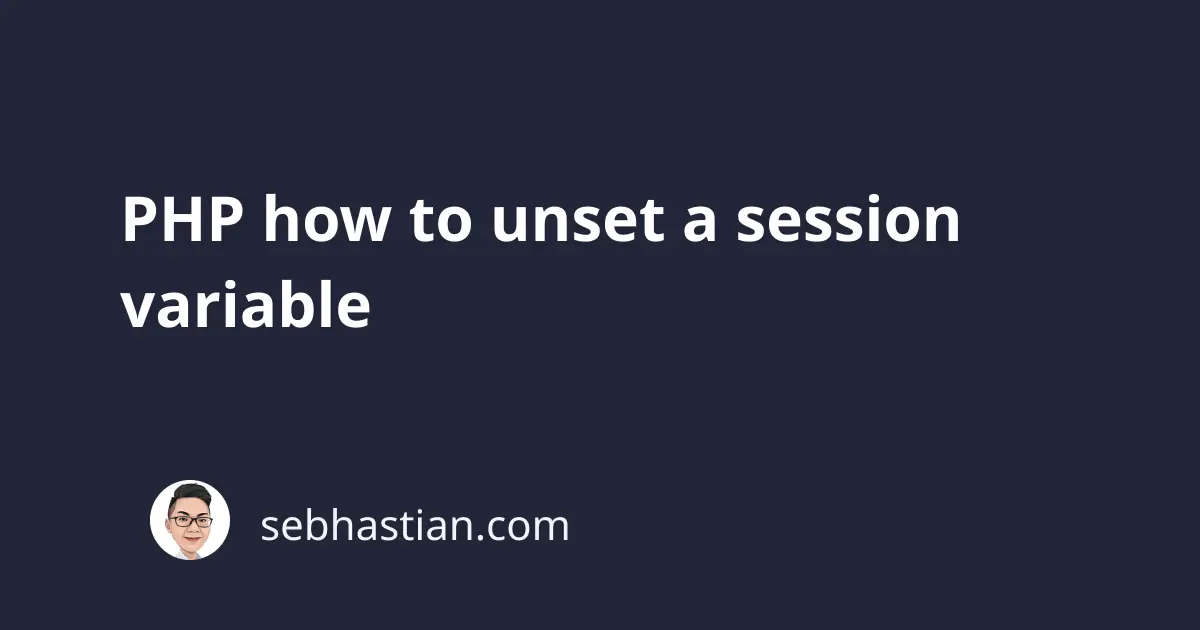
There are three ways you can unset session variables in PHP:
This tutorial will help you learn the methods mentioned above.
Unset session variable with unset
To unset your session variable in PHP, you need to call the unset
construct and pass the $_SESSION
variable as its parameter.
Here’s an example:
<?php
session_start();
$_SESSION["User"] = "Nathan";
$_SESSION["lang"] = "en";
// 👇 unset User session
unset($_SESSION["User"]);
// 👇 Array ( [lang] => en )
print_r($_SESSION);
?>
Although unset
is a construct, you need to surround the parameters with round brackets.
The unset
construct allows you to unset multiple variables with one call as well:
<?php
session_start();
$_SESSION["User"] = "Nathan";
$_SESSION["lang"] = "en";
$_SESSION["theme"] = "dark";
// 👇 unset two session variables
unset($_SESSION["User"], $_SESSION["lang"]);
// 👇 Array ( [theme] => dark )
print_r($_SESSION);
?>
Using the unset
construct you can remove parts of your session data selectively.
Unset session variable with session_unset()
If you want to clear the entire session, you can use either the session_unset()
or session_destroy()
function.
The session_unset()
function unset all your session variables:
<?php
session_start();
$_SESSION["User"] = "Nathan";
$_SESSION["lang"] = "en";
// 👇 unset all variables
session_unset();
// 👇 Array ()
print_r($_SESSION);
?>
session_unset()
immediately clears the session variables. It’s the same as assigning the $_SESSION
variable to an empty array:
$_SESSION = array();
This function doesn’t interrupt any concurrent requests that require access to the session.
If you need to immediately clear the session from all requests to your server, you need to call the session_destroy()
function.
Unset session variable with session_destroy()
session_destroy()
destroys the session, so PHP will generate a new session id when you call session_start()
again
To get rid of the entire session, you also need to remove the session cookie as follows:
<?php
session_start();
// Unset all of the session variables.
$_SESSION = array();
// or
session_unset();
// If it's desired to kill the session, also delete the session cookie.
// Note: This will destroy the session, and not just the session data!
if (ini_get("session.use_cookies")) {
$params = session_get_cookie_params();
setcookie(session_name(), '', time() - 42000,
$params["path"], $params["domain"],
$params["secure"], $params["httponly"]
);
}
// Finally, destroy the session.
session_destroy();
?>
PHP sessions are used to store information about your web application.
Most of the time, you don’t want to clear the entire session data. The unset
construct is the preferred way to remove session data.
But if you have cases where you want to remove the session completely, then using the above example with session_destroy()
should be the way to go.
Now you’ve learned how to unset the session variable in PHP. Great! 👍